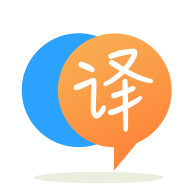
[英]Java/Eclipse: “Lambda expressions cannot be used in an evaluation expression”
[英]In Lambda Expressions (Java), how is an expression without parameter used?
據我所知,有3種java lambda表達式。
(int x, int y) -> { return x + y; }
x -> x * x
( ) -> x
第三個似乎從未使用過。
你能舉一個例子來說明3個案例中的每個案例(案例3的另一個例子是好的)來說明它們的用法嗎? 請使它們盡可能簡單(最好從list.stream()....開始)
第一個表達式將用於獲取方法的2個參數並返回值的位置。
第二個表達式將使用
x -> x * x
,其中您獲得方法的1個參數並返回值。第三個表達式
( ) -> x
將用於( ) -> x
,其中您獲得方法的0個參數並返回一個值。
我們來看第三個。 假設您有一個不帶參數的接口並返回一個值。
static interface RandomMath {
public int random();
}
現在您想要實例化此接口及其實現。 不使用lambda,將按如下方式完成: -
Random random = new Random();
RandomMath randomMath = new RandomMath() {
@Override
public int random() {
return random.nextInt();
}
};
使用lambda就像: -
Random random = new Random();
RandomMath randomMath = () -> random.nextInt(); //the third type.
類似地,對於前兩個,它可以用於采用兩個和一個參數並返回值的方法。
static interface PlusMath {
public int plus(int a, int b);
}
PlusMath plusMath = (a, b) -> a + b;
static interface SquareMath {
public int square(int a);
}
SquareMath squareMath = a -> a * a;
前兩個例子與上一個例子不同。 函數中的變量(lambda表達式)指的是其參數。
而在第三個示例中, x
指的是lambda表達式之外但在詞法范圍內的變量(可以是方法或實例變量中的局部變量)。
示例1(通常是stream reduce)通過將列表中到目前為止計算的sum和next項傳遞給lambda函數來計算總和:
int sum = list.stream().reduce((int x, int y) -> x+y);
例2,計算元素的方塊:
squares = list.stream().map((int x) -> x*x).collect(Collectors.toList());
示例3,如果元素在列表中為null,則將元素設置為默認值:
final int x = MY_DEFAULT_VALUE;
// lambda refers the the variable above to get the default
defaults = list.stream().map((Integer v) -> v != null ? v : x);
或者更好的例如3是地圖原子方法:
int x = MY_DEFAULT_VALUE;
// lambda refers the the variable above to get the default
map.computeIfAbsent(1, (Integer key) -> x);
// the same could be achieved by putIfAbsent() of course
// but typically you would use (Integer key) -> expensiveComputeOfValue(x)
// ...
// or quite common example with executor
public Future<Integer> computeAsync(final int value) {
// pass the callback which computes the result synchronously, to Executor.submit()
// the callback refers to parameter "value"
return executor.submit(() -> computeSync(value));
}
在完成以下示例之前,請注意,可以為任何SAM(也稱為Functional)接口編寫Lambda表達式 (Infact, Lambda表達式是用於替換Java中的詳細匿名類(使用單個方法) 的語法糖 )。
單個抽象方法接口或功能接口是一個只包含一個abstract
方法的接口,您可以在此處查看 。 如果您知道這一點,您可以編寫(使用)任意數量的自己的Functional接口,然后根據每個Functional接口方法編寫不同的Lambda表達式。
下面的示例是通過使用現有的JDK(1.8)功能接口(如Callable
, Function
, BiFunction
(就像這些, 在JDK 1.8中有許多內置的功能接口,大多數時候它們很容易滿足我們的要求 )。
(1)(int x,int y) - > {return x + y; }
//Below Lamda exp, takes 2 Input int Arguments and returns string
BiFunction<Integer, Integer, String> biFunction = (num1, num2) ->
"Sum Is:" +(num1 + num2);
System.out.println(biFunction.apply(100, 200));
(2)x - > x * x的例子
//Below Lamda exp, takes string Input Argument and returns string
list.stream().map((String str1) ->
str1.substring(0, 1)).
forEach(System.out::println);
(3)() - > x的例子
//Below Lambda exp, Input argument is void, returns String
Callable<String> callabl = () -> "My Callable";
ExecutorService service = Executors.newSingleThreadExecutor();
Future<String> future = service.submit(callable);
System.out.println(future.get());
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.