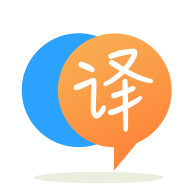
[英]C How can I acces struct members of a struct that is part of a struct array in another struct?
[英]how can i change the struct in C array?
我在更改作為數組的結構時遇到問題。
我正在使用C語言開發一個圖書館項目,需要將一本書(Book結構)添加到圖書館中,我有一個包含所有書籍的數組,我需要將我的新書添加到該數組中。
我做到了,有人可以幫助我,並給我一點有關此的信息嗎?
#include <stdio.h>
#include <string.h>
#define BOOK_NUM 50
#define NAME_LENGTH 200
#define AUTHOR_NAME_LENGTH 100
#define PUBLISHER_NAME_LENGHT 50
#define GENRE_LENGHT 50
typedef struct _Book
{
char name[NAME_LENGTH];
char author[AUTHOR_NAME_LENGTH];
char publisher[PUBLISHER_NAME_LENGHT];
char genre[GENRE_LENGHT];
int year;
int num_pages;
int copies;
}Book;
Book books_arr[BOOK_NUM],*ptr=books_arr;
void add_book()
{
char book_name[NAME_LENGTH],author_name[AUTHOR_NAME_LENGTH],publisher_name[PUBLISHER_NAME_LENGHT],book_genre[GENRE_LENGHT];
int book_year,book_pages,book_copies,cnt=0,cnt2=0;
printf("Please enter book name:\n");
scanf("%s",&book_name);
printf("Please enter author name:\n");
scanf("%s",&author_name);
printf("Please enter publisher name:\n");
scanf("%s",&publisher_name);
printf("Please enter book genre:\n");
scanf("%s",&book_genre);
printf("Please enter the year of publishment:\n");
scanf("%d",&book_year);
printf("Please enter the number of pages:\n");
scanf("%d",&book_pages);
printf("Please enter the number of copies:\n");
scanf("%d",&book_copies);
for (ptr=books_arr;ptr<&books_arr[BOOK_NUM];ptr++)
{
if (strcmp(book_name,(*ptr).name)==0)
(*ptr).copies=(*ptr).copies+book_copies;
if(strcmp(book_name,(*ptr).name)!=0)
cnt++;
if((*ptr).name!=NULL)
cnt2++;
}
if(cnt==BOOK_NUM)
{
if(cnt2==BOOK_NUM)
printf("There is no place in the library for this book\n");
if(cnt2<BOOK_NUM)
{
(*ptr).name=book_name;
(*ptr).author=author_name;
(*ptr).publisher=publisher_name;
(*ptr).genre=book_genre;
(*ptr).year=book_year;
(*ptr).num_pages=book_pages;
(*ptr).copies=book_copies;
}
}
}
每次編譯代碼時,都會出現“表達式必須是可修改的左值”的問題。
謝謝
而不是這樣做
(*ptr).name=book_name;
您應該使用strcpy
復制一個字符串
strcpy((*ptr).name,book_name);
另外,考慮使用其他安全功能,例如strncpy()
。
請注意使用ptr->name
而不是(*ptr).name
。
ptr->name
是一個數組,您不能更改數組,但是可以更改數組的內容,這就是錯誤提示。
您正在嘗試將一個數組分配給另一個數組。 在C語言中這是不合法的。
如果要將包含字符串的字符數組的內容復制到另一個字符數組,請使用strcpy
。
strcpy(ptr->name, book_name);
strcpy(ptr->author, author_name);
strcpy(ptr->publisher, publisher_name);
strcpy(ptr->genre, book_genre);
還要注意使用->
運算符來訪問指向成員的指針。
除此之外,您還無法正確讀取這4個字符串。 用於scanf
的%s
格式說明符期望一個指向char
數組中第一個元素的指針。 您正在做的是傳遞數組本身的地址。
為此,只需輸入數組的名稱。 數組名稱在傳遞給函數時會衰減為指向第一個元素的指針。
scanf("%s",book_name);
printf("Please enter author name:\n");
scanf("%s",author_name);
printf("Please enter publisher name:\n");
scanf("%s",publisher_name);
printf("Please enter book genre:\n");
scanf("%s",book_genre);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.