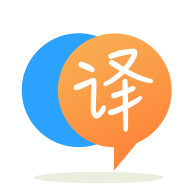
[英]Library not loaded: /System/Library/Frameworks/Contacts.framework/Contacts?
[英]Exporting all contacts in one .vcf file using Contacts.Framework in Objective - C
我使用AddressBook.framework從所有聯系人創建Contacts.vcf,並將其保存在Documents Directory中。 這是我以前使用的代碼:
ABAddressBookRef addressBook1 = ABAddressBookCreate();
NSArray *arrayOfAllPeople = (__bridge_transfer NSArray *) ABAddressBookCopyArrayOfAllPeople(addressBook1);
long cnt = (unsigned long)[arrayOfAllPeople count];
if (cnt==0) {
ABAddressBookRequestAccessWithCompletion(addressBook1, nil);
}
if(ABAddressBookGetAuthorizationStatus() == kABAuthorizationStatusAuthorized)
{
ABAddressBookRef addressBook2 = ABAddressBookCreate();
CFArrayRef contacts = ABAddressBookCopyArrayOfAllPeople(addressBook2);
CFDataRef vcards = (CFDataRef)ABPersonCreateVCardRepresentationWithPeople(contacts);
NSString *vcardString = [[NSString alloc] initWithData:(__bridge NSData *)vcards encoding:NSUTF8StringEncoding];
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *folderPath = [paths objectAtIndex:0];
NSString *filePath = [folderPath stringByAppendingPathComponent:@"Contacts.vcf"];
[vcardString writeToFile:filePath atomically:YES encoding:NSUTF8StringEncoding error:nil];
CFRelease(addressBook2); }
如何使用Contacts.framework創建具有所有設備聯系人的Contacts.vcf文件並將其保存在documents目錄中?
您可以使用此方法來獲取.vcf文件中的所有聯系人。 它返回與使用AddressBook.framework相同的輸出。
- (void)getContacts {
NSMutableArray *contactsArray=[[NSMutableArray alloc] init];
CNContactStore *store = [[CNContactStore alloc] init];
[store requestAccessForEntityType:CNEntityTypeContacts completionHandler:^(BOOL granted, NSError * _Nullable error) {
if (!granted) {
dispatch_async(dispatch_get_main_queue(), ^{
});
return;
}
NSMutableArray *contacts = [NSMutableArray array];
NSError *fetchError;
CNContactFetchRequest *request = [[CNContactFetchRequest alloc] initWithKeysToFetch:@[[CNContactVCardSerialization descriptorForRequiredKeys], [CNContactFormatter descriptorForRequiredKeysForStyle:CNContactFormatterStyleFullName]]];
BOOL success = [store enumerateContactsWithFetchRequest:request error:&fetchError usingBlock:^(CNContact *contact, BOOL *stop) {
[contacts addObject:contact];
}];
if (!success) {
NSLog(@"error = %@", fetchError);
}
// you can now do something with the list of contacts, for example, to show the names
CNContactFormatter *formatter = [[CNContactFormatter alloc] init];
for (CNContact *contact in contacts) {
[contactsArray addObject:contact];
// NSString *string = [formatter stringFromContact:contact];
//NSLog(@"contact = %@", string);
}
//NSError *error;
NSData *vcardString =[CNContactVCardSerialization dataWithContacts:contactsArray error:&error];
NSString* vcardStr = [[NSString alloc] initWithData:vcardString encoding:NSUTF8StringEncoding];
NSLog(@"vcardStr = %@",vcardStr);
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *folderPath = [paths objectAtIndex:0];
NSString *filePath = [folderPath stringByAppendingPathComponent:@"Contacts.vcf"];
[vcardStr writeToFile:filePath atomically:YES encoding:NSUTF8StringEncoding error:nil];
}];
}
從iOS 9+版本開始,不推薦使用AddressBookUI.framework和Addressbook.framework。 Apple引入了ContactUI.framework和Contact.framework,並且對AddressBookUI.framework和Addressbook.framework進行了增強。 在此博客中,我們將討論如何使用這兩個新框架以及如何導出VCard。 讓我們開始從電話聯系人中選擇聯系人並訪問該人的基本信息。
步驟1.創建新的Xcode項目名稱ContactDemo
並導入Contacts.framework和ContactsUI.framework
,如圖所示。
步驟2.在項目中添加UIButton
, UIImageView
和3個UILabel
,如圖所示:
步驟3.在相應的視圖控制器中創建按鈕動作,imageview和標簽的出口,如下所示:
@property (weak, nonatomic) IBOutlet UIImageView *personImage;
@property (weak, nonatomic) IBOutlet UILabel *personName;
@property (weak, nonatomic) IBOutlet UILabel *emailId;
@property (weak, nonatomic) IBOutlet UILabel *phoneNo;
- (IBAction)selectAction:(id)sender;
步驟4.將委托CNContactPickerDelegate
添加到viewController
。
步驟5.添加委托方法:
- (void) contactPicker:(CNContactPickerViewController *)picker
didSelectContact:(CNContact *)contact {
[self getContactDetails:contact];
}
該委托方法將以CNContact
對象的形式返回聯系人,該聯系人將在本地方法中進一步處理
-(void)getContactDetails:(CNContact *)contactObject {
NSLog(@"NAME PREFIX :: %@",contactObject.namePrefix);
NSLog(@"NAME SUFFIX :: %@",contactObject.nameSuffix);
NSLog(@"FAMILY NAME :: %@",contactObject.familyName);
NSLog(@"GIVEN NAME :: %@",contactObject.givenName);
NSLog(@"MIDDLE NAME :: %@",contactObject.middleName);
NSString * fullName = [NSString stringWithFormat:@"%@ %@",contactObject.givenName,contactObject.familyName];
[self.personName setText:fullName];
if(contactObject.imageData) {
NSData * imageData = (NSData *)contactObject.imageData;
UIImage * contactImage = [[UIImage alloc] initWithData:imageData];
[self.personImage setImage:contactImage];
}
NSString * phone = @"";
NSString * userPHONE_NO = @"";
for(CNLabeledValue * phonelabel in contactObject.phoneNumbers) {
CNPhoneNumber * phoneNo = phonelabel.value;
phone = [phoneNo stringValue];
if (phone) {
userPHONE_NO = phone;
}}
NSString * email = @"";
NSString * userEMAIL_ID = @"";
for(CNLabeledValue * emaillabel in contactObject.emailAddresses) {
email = emaillabel.value;
if (email) {
userEMAIL_ID = email;
}}
NSLog(@"PHONE NO :: %@",userPHONE_NO);
NSLog(@"EMAIL ID :: %@",userEMAIL_ID);
[self.emailId setText:userEMAIL_ID];
[self.phoneNo setText:userPHONE_NO];
}
步驟6.創建CNContactPickerViewController
類對象,並在按鈕IBAction方法中注冊其委托:
- (IBAction) selectAction:(id)sender {
CNContactPickerViewController *contactPicker = [CNContactPickerViewController new];
contactPicker.delegate = self;
[self presentViewController:contactPicker animated:YES completion:nil];
}
[self presentViewController:contactPicker animated:YES completion:nil];
將顯示聯系人列表視圖。
步驟7.運行項目
一種 。 主視圖
B.點擊“選擇聯系人”按鈕, CNContactPickerViewController
將打開,如圖所示:
C.選擇一個聯系人,視圖將關閉,您將獲得該聯系人的詳細信息,如圖所示:
之前我們有寫權限代碼來訪問聯系人,但是現在它隱式地授予了訪問聯系人的權限。 使用此框架,我們還可以生成VCard(VCF)並在其他平台之間共享。 這是創建VCard的步驟。
步驟1.從CNContactPickerViewController
選擇聯系人, CNContactPickerViewController
,您將在委托中獲得CNContact
對象。
步驟2.將聯系人保存在文檔目錄中。 由於數據以NSData
形式存儲,因此要將聯系人轉換為NSData
使用以NSData
格式表示VCard的CNContactVCardSerialization
類。
- (NSString *) saveContactToDocumentDirectory:(CNContact *)contact {
NSArray *paths = NSSearchPathForDirectoriesInDomains (NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
NSString * VCardPath = [documentsDirectory stringByAppendingString:@"/VCard.vcf"];
NSArray *array = [[NSArray alloc] initWithObjects:contact, nil];
NSError *error;
NSData *data = [CNContactVCardSerialization dataWithContacts:array error:&error];
[data writeToFile:VCardPath atomically:YES];
return VCardPath;
}
CNContactVCardSerialization
類方法dataWithContacts:error:
獲取聯系人對象數組( CNContact
類Object)。
saveContactToDocumentDirectory
方法將返回Vcard的文件路徑。 使用文件路徑,您可以將聯系人導出到任何需要的地方。
來源: Contacts UI,Contacts Framework和在Objective-C中創建VCard(VCF)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.