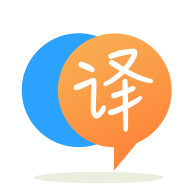
[英]Entity Framework On Update : Store update, insert, or delete statement affected an unexpected number of rows
[英]Entity Framework: “Store update, insert, or delete statement affected an unexpected number of rows (0).”
首先使用MVC5代碼,嘗試編輯任何模型都會給我以下錯誤:實體框架:“存儲更新,插入或刪除語句影響了意外的行數(0)。”這是我的控制器,調試器正在調用db.SaveChanges();
錯誤db.SaveChanges();
,但我不知道為什么。
// GET: Artists/Edit/5
public ActionResult Edit(int? id)
{
if (id == null)
{
return new HttpStatusCodeResult(HttpStatusCode.BadRequest);
}
Artist artist = db.Artists.Find(id);
if (artist == null)
{
return HttpNotFound();
}
return View(artist);
}
// POST: Artists/Edit/5
// To protect from overposting attacks, please enable the specific properties you want to bind to, for
// more details see http://go.microsoft.com/fwlink/?LinkId=317598.
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Edit([Bind(Include = "ID,Name,Picture,BirthDate,Nationality,ArtStyle,Info,Rating,Artwork1,Artwork2,Artwork3")] Artist artist)
{
if (ModelState.IsValid)
{
db.Entry(artist).State = EntityState.Modified;
db.SaveChanges();
return RedirectToAction("Index");
}
return View(artist);
}
藝術家模型:
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Data.Entity;
namespace ArtistDatabase.Models
{
public class Artist
{
[Key]
public int ArtistID { get; set; }
//----------------------------------------------------------------------------------------------
[Required,StringLength(60, MinimumLength = 3), Display(Name = "Artist")]
public string Name { get; set; }
//----------------------------------------------------------------------------------------------
[DataType(DataType.ImageUrl)]
public string Picture { get; set; }
//----------------------------------------------------------------------------------------------
[System.ComponentModel.DataAnnotations.Schema.Column(TypeName = "datetime2")]
[Display(Name = "Date of Birth"),DataType(DataType.Date),DisplayFormat(DataFormatString = "{0:dd-MM-yyyy}", ApplyFormatInEditMode = true)]
public DateTime BirthDate { get; set; }
//----------------------------------------------------------------------------------------------
[Required,StringLength(30)]
public string Nationality { get; set; }
//----------------------------------------------------------------------------------------------
[Display(Name = "Style/Movement")]
public string ArtStyle { get; set; }
//----------------------------------------------------------------------------------------------
[DataType(DataType.MultilineText)]
public string Info { get; set; }
//----------------------------------------------------------------------------------------------
[RegularExpression(@"^[A-Z]+[a-zA-Z''-'\s]*$")]
[StringLength(5)]
public string Rating { get; set; }
//----------------------------------------------------------------------------------------------
[Display(Name = "Famous work: "),DataType(DataType.ImageUrl)]
public string Artwork1 { get; set; }
//----------------------------------------------------------------------------------------------
[Display(Name = " "), DataType(DataType.ImageUrl)]
public string Artwork2 { get; set; }
//----------------------------------------------------------------------------------------------
[Display(Name = " "), DataType(DataType.ImageUrl)]
public string Artwork3 { get; set; }
//----------------------------------------------------------------------------------------------
public virtual ICollection<Artwork> Artworks { get; set; }
}
public class ArtistDBContext : DbContext
{
public DbSet<Artist> Artists { get; set; }
public DbSet<Artwork> Artworks { get; set; }
}
}
編輯視圖:
@model ArtistDatabase.Models.Artist
@{
ViewBag.Title = "Edit";
}
<h2>Edit</h2>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<h4>@Html.DisplayFor(model => model.Name)</h4>
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
@Html.HiddenFor(model => model.ArtistID)
<div class="form-group">
@Html.LabelFor(model => model.Name, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Name, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Name, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Picture, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Picture, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Picture, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.BirthDate, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.BirthDate, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.BirthDate, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Nationality, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Nationality, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Nationality, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.ArtStyle, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.ArtStyle, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.ArtStyle, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Info, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Info, new { htmlAttributes = new { @class = "form-control", @rows = 10, @cols = 80 } })
@Html.ValidationMessageFor(model => model.Info, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Artwork1, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Artwork1, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Artwork1, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Artwork2, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Artwork2, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Artwork2, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Artwork3, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Artwork3, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Artwork3, "", new { @class = "text-danger" })
</div>
</div>
</div>
<hr />
<div class="row">
<div class="col-sm-6 text-left">
@using (Html.BeginForm())
{
<div class="form-actions">
<input type="submit" value="Save" class="btn btn-default" />
@Html.ActionLink("Cancel", "Details", new { id = Model.ArtistID }, new { @class = "btn btn-default" })
</div>
}
</div>
<div class="col-sm-6 text-right">
@Html.ActionLink("Profile", "Details", new { id = Model.ArtistID }, new { @class = "btn btn-default" })
@Html.ActionLink("Artists", "Index", null, new { @class = "btn btn-default" })
</div>
</div>
}
@section Scripts {
@Scripts.Render("~/bundles/jqueryval")
}
您可以在這里進行測試: http : //www.artistdb.willcardoso.net/
您可以通過以下方法解決此問題:A)修改保存調用以添加對象,或B)僅在編輯時不更改主鍵。 我做了B)。
您的綁定不正確。 您正在使用:
public ActionResult Edit([Bind(Include = "ID,Name,Picture,BirthDate,Nationality,ArtStyle,Info,Rating,Artwork1,Artwork2,Artwork3")] Artist artist)
但關鍵是ArtistID
綁定中的ID
應該是ArtistID
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.