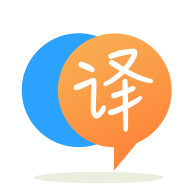
[英]How can i create a class with a property for each country and it's items?
[英]How can i create a directory for each name that it's code belong to?
我正在從鏈接下載文件,到目前為止將它們保存在一個目錄中。 但是現在我需要將每組文件下載到特定目錄。
這是我作為現有項目添加到項目中的第一個文本文件的內容。 國家名稱。
Europe
Alps
Benelux
Germany
Spain & Portugal
France
Greece
Italy
Poland
Scandinavia
Turkey
UK & Ireland
Russia
Baltic
Balkan
Romania & Bulgaria
Hungary
Africa
Algeria
Cameroon
CanaryIslands
Congo
CentralAfrica
Nigeria
Chad
Egypt
Ethiopia
Israel
Libya
Madagascar
Morocco
Namibia
SaudiArabia
Somalia
SouthAfrica
Sudan
Tanzania
Tunesia
WestAfrica
Zambia
我還有另一個文本文件,顯示每個國家/地區代碼:
eu
alps
nl
de
sp
fr
gr
it
pl
scan
tu
gb
ru
bc
ba
se
hu
af
dz
cm
ce
cg
caf
ng
td
eg
et
is
ly
mg
mo
bw
sa
so
za
sd
tz
tn
wa
zm
為什么代碼很重要? 因為每個鏈接都是使用國家/地區代碼(而非名稱)構建的。 例如:
http://www.sat24.com/image2.ashx?region=is&time=201612271600&ir=true
因此,我知道鏈接中的region = is部分意味着在這種情況下,國家/地區代碼為:“ is”(以色列)。
因此,現在我需要找到所有具有代碼“ is”的鏈接,這些鏈接應下載到以色列目錄中。
下一步是鏈接與其他區域的連接時,例如:
http://www.sat24.com/image2.ashx?region=tu&time=201612271600&ir=true
因此,現在代碼tu適用於土耳其國家/地區,因此現在每個帶有代碼tu的鏈接都應下載到土耳其目錄。
因此,第一個問題是如何在指向國家名稱的鏈接中的國家代碼之間進行連接,然后將其下載到我已經在構造函數中創建的正確國家名稱目錄中? 我已經准備好所有國家名稱目錄,但是我需要某種方式在國家名稱目錄鏈接中的代碼(區域)之間進行連接。
第二個問題是我每15分鍾下載一次。 稍后我將使用計時器。 每15分鍾重新下載一次圖像。 但是我不想刪除或覆蓋舊圖像,主要思想是保存和保留圖像。 問題是每15分鍾在每個國家/地區我應創建哪些子路徑? 我的意思是每15分鍾給每個國家子路徑一個名稱?
我想為每個國家/地區創建一個目錄,其中包含下載圖像的日期和時間范圍,但是我不確定這是否是一個好主意。
稍后,我將希望能夠在每個國家/地區的此目錄之間移動,並將圖像加載到pictureBox。 問題是我將如何識別每15分鍾下載一次的每個目錄?
問題不在於下載程序是否正常。 目錄存在兩個問題。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using System.Diagnostics;
using System.IO;
using System.Net;
using System.Text.RegularExpressions;
namespace Downloader
{
public partial class Form1 : Form
{
int countCompleted = 0;
ExtractImages ei = new ExtractImages();
List<string> newList = new List<string>();
List<string> countryList = new List<string>();
List<string> countriesPaths = new List<string>();
private Queue<string> _downloadUrls = new Queue<string>();
public Form1()
{
InitializeComponent();
ManageDirectories();
lblDownloads.Text = "0";
ei.Init();
foreach (ExtractImages.Continent continent in ei.world.continents)
{
foreach (ExtractImages.Country country in continent.countries)
{
if (country.name == "Israel")
{
foreach (string imageUri in country.imageUrls)
{
countryList.Add(imageUri);
}
}
else
{
foreach (string imageUri in country.imageUrls)
{
newList.Add(imageUri);
}
}
}
}
}
private void ManageDirectories()
{
string savedImagesPath = Path.GetDirectoryName(Application.LocalUserAppDataPath);
string mainPath = "Countries";
mainPath = Path.Combine(savedImagesPath, mainPath);
string[] lines = File.ReadAllLines("CountriesNames.txt");
foreach(string path in lines)
{
string countryPath = Path.Combine(mainPath, path);
if (!Directory.Exists(countryPath))
{
Directory.CreateDirectory(countryPath);
}
countriesPaths.Add(countryPath);
}
string[] countriesCodes = File.ReadAllLines("CountriesCodes.txt");
}
private void downloadFile(IEnumerable<string> urls)
{
foreach (var url in urls)
{
_downloadUrls.Enqueue(url);
}
// Starts the download
btnStart.Text = "Downloading...";
btnStart.Enabled = false;
pbStatus.Visible = true;
DownloadFile();
}
int count = 0;
private void DownloadFile()
{
if (_downloadUrls.Any())
{
WebClient client = new WebClient();
client.DownloadProgressChanged += client_DownloadProgressChanged;
client.DownloadFileCompleted += client_DownloadFileCompleted;
var url = _downloadUrls.Dequeue();
//string FileName = url.Substring(url.LastIndexOf("/") + 1,
// (url.Length - url.LastIndexOf("/") - 1));
client.DownloadFileAsync(new Uri(url), countriesPaths[count] + ".gif");
RichTextBoxExtensions.AppendText(richTextBox1, "Downloading: ", Color.Red);
RichTextBoxExtensions.AppendText(richTextBox1, url, Color.Green);
richTextBox1.AppendText(Environment.NewLine);
count++;
return;
}
// End of the download
btnStart.Text = "Download Complete";
countCompleted = newList.Count;
lblDownloads.Text = countCompleted.ToString();
timer1.Enabled = true;
downloadFile(newList);
}
private void client_DownloadFileCompleted(object sender, AsyncCompletedEventArgs e)
{
if (e.Error != null)
{
RichTextBoxExtensions.UpdateText(richTextBox1, "Downloading: ", "Downloaded: ", Color.Red);
// handle error scenario
throw e.Error;
}
else
{
countCompleted--;
lblDownloads.Text = countCompleted.ToString();
RichTextBoxExtensions.UpdateText(richTextBox1, "Downloading: ", "Downloaded: ", Color.Green);
}
if (e.Cancelled)
{
// handle cancelled scenario
}
DownloadFile();
}
void client_DownloadProgressChanged(object sender, DownloadProgressChangedEventArgs e)
{
double bytesIn = double.Parse(e.BytesReceived.ToString());
double totalBytes = double.Parse(e.TotalBytesToReceive.ToString());
double percentage = bytesIn / totalBytes * 100;
pbStatus.Value = int.Parse(Math.Truncate(percentage).ToString());
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void btnStart_Click(object sender, EventArgs e)
{
countCompleted = countryList.Count;
lblDownloads.Text = countCompleted.ToString();
downloadFile(countryList);
}
public class RichTextBoxExtensions
{
public static void AppendText(RichTextBox box, string text, Color color)
{
box.SelectionStart = box.TextLength;
box.SelectionLength = 0;
box.SelectionColor = color;
box.AppendText(text);
box.SelectionColor = box.ForeColor;
}
public static void UpdateText(RichTextBox box, string find, string replace, Color? color)
{
box.SelectionStart = box.Find(find, RichTextBoxFinds.Reverse);
box.SelectionLength = find.Length;
box.SelectionColor = color ?? box.SelectionColor;
box.SelectedText = replace;
}
}
private void timer1_Tick(object sender, EventArgs e)
{
}
private void SortList()
{
}
}
}
只需使用通用詞典將國家/地區代碼綁定到目錄路徑中使用的全名即可
Dictionary<string, string> countryCodeMapping = new Dictionary<string, string>() {
{"us", "United States"},
{"is", "Isreal"}
...
};
如果按照示例中的順序對這兩個文件進行了排序,則可以創建字典形式的代碼來全稱。
var codes = new List<string>() { ..... }
var countries = new List<string> () { ....}
創建字典將如下所示
var codeToFullNameMap = codes
.Select((code, index) => index)
.ToDictionary(
keySelector: index => codes[index].
elementSelector: index => cointires[index]);
然后創建字典,就可以通過其代碼訪問完整的國家/地區名稱。
var countryName = codeToFullNameMap["tu"];
關於第二個問題。 如果命名為您提供了足夠的信息,並且使用了它認為的當前日期時間,那就應該可以了。
我的建議是為每個下載開始時間的國家代碼和子文件夾創建目錄(我猜包括小時,分鍾和秒就足夠了)。
TU // (main folder)
-> 2017-01-26-18-50-10 // (subfolder 1)
-> img#1
-> img#2
-> 2017-01-26-18-50-10 // (subfolder 2)
我不確定第三個問題。 如果您的文件夾名為TU,這足以說明這些圖像是用於土耳其的,還是我缺少什么? 每個子文件夾都包含從上一個文件夾開始每15分鍾下載一次的圖像。
也許,如果您分享有關第三個問題的更多細節,您究竟在哪里看到問題,例如,您需要按國家形象化桌面應用程序中的圖像?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.