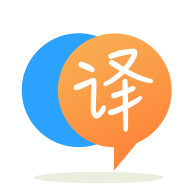
[英]What is the most pythonic way to split a string into contiguous, overlapping list of words
[英]What's the pythonic way to split a string using a list of sizes?
實現這個的pythonic方法是什么:
s = "thisismystring"
keys = [4, 2, 2, 6]
new = []
i = 0
for k in keys:
new.append(s[i:i+k])
i = i+k
這確實給了我['this', 'is', 'my', 'string']
,但是我覺得這是一種更優雅的方式。 建議?
您可以使用itertools.accumulate()
,也許:
from itertools import accumulate
s = "thisismystring"
keys = [4, 2, 2, 6]
new = []
start = 0
for end in accumulate(keys):
new.append(s[start:end])
start = end
你可以內聯start
通過增加另一個值accumulate()
從零開始電話:
for start, end in zip(accumulate([0] + keys), accumulate(keys)):
new.append(s[start:end])
這個版本可以制成列表理解:
[s[a:b] for a, b in zip(accumulate([0] + keys), accumulate(keys))]
演示后者版本:
>>> from itertools import accumulate
>>> s = "thisismystring"
>>> keys = [4, 2, 2, 6]
>>> [s[a:b] for a, b in zip(accumulate([0] + keys), accumulate(keys))]
['this', 'is', 'my', 'string']
double accumulate可以替換為tee()
,包含在itertools
文檔中的itertools
pairwise()
函數中 :
from itertools import accumulate, chain, tee
def pairwise(iterable):
"s -> (s0,s1), (s1,s2), (s2, s3), ..."
a, b = tee(iterable)
next(b, None)
return zip(a, b)
[s[a:b] for a, b in pairwise(accumulate(chain([0], keys)))]
我在一個itertools.chain()
調用中輸入前綴為0的起始位置,而不是創建一個帶有連接的新列表對象。
我會使用enumerate
,累積:
[s[sum(keys[:i]): sum(keys[:i]) + k] for i, k in enumerate(keys)]
用你的例子:
>>> s = "thisismystring"
>>> keys = [4, 2, 2, 6]
>>> new = [s[sum(keys[:i]): sum(keys[:i]) + k] for i, k in enumerate(keys)]
>>> new
['this', 'is', 'my', 'string']
可以使用islice
。 可能效率不高,但可能至少有趣而且簡單。
>>> from itertools import islice
>>> s = 'thisismystring'
>>> keys = [4, 2, 2, 6]
>>> it = iter(s)
>>> [''.join(islice(it, k)) for k in keys]
['this', 'is', 'my', 'string']
僅僅因為我認為必須有辦法在沒有顯式循環的情況下做到這一點:
import re
s = "thisismystring"
keys = [4, 2, 2, 6]
new = re.findall((r"(.{{{}}})" * len(keys)).format(*keys), s)[0]
print(new)
OUTPUT
('this', 'is', 'my', 'string')
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.