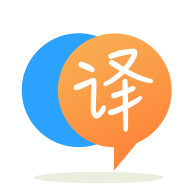
[英]Grouping with PersistedFaceIds in Microsoft Cognitive Services Face API
[英]Microsoft Cognitive Face API- how can i get Face Attributes in video feed from Kinect?
我正在探索 Microsoft Cognitive Face API,我對它很陌生。 我能夠通過一個簡單的圖像實現人臉屬性,但是我的問題是如何在 WPF c# 中從 Kinect 的實時視頻源中獲取一個人的人臉屬性。 如果有人可以幫助我,那就太好了。 提前致謝!
我曾嘗試每隔 2 秒從 Kinect 顏色饋送中將幀捕獲到某個文件位置,並使用該文件路徑並將其轉換為 Stream,然后將其傳遞給 Face-API 函數,然后就成功了。 以下是我試過的代碼。
namespace CognitiveFaceAPISample
{
public partial class MainWindow : Window
{
private readonly IFaceServiceClient faceServiceClient = new FaceServiceClient("c2446f84b1eb486ca11e2f5d6e670878");
KinectSensor ks;
ColorFrameReader cfr;
byte[] colorData;
ColorImageFormat format;
WriteableBitmap wbmp;
BitmapSource bmpSource;
int imageSerial;
DispatcherTimer timer,timer2;
string streamF = "Frames//frame.jpg";
public MainWindow()
{
InitializeComponent();
ks = KinectSensor.GetDefault();
ks.Open();
var fd = ks.ColorFrameSource.CreateFrameDescription(ColorImageFormat.Bgra);
uint frameSize = fd.BytesPerPixel * fd.LengthInPixels;
colorData = new byte[frameSize];
format = ColorImageFormat.Bgra;
imageSerial = 0;
cfr = ks.ColorFrameSource.OpenReader();
cfr.FrameArrived += cfr_FrameArrived;
}
void cfr_FrameArrived(object sender, ColorFrameArrivedEventArgs e)
{
if (e.FrameReference == null) return;
using (ColorFrame cf = e.FrameReference.AcquireFrame())
{
if (cf == null) return;
cf.CopyConvertedFrameDataToArray(colorData, format);
var fd = cf.FrameDescription;
// Creating BitmapSource
var bytesPerPixel = (PixelFormats.Bgr32.BitsPerPixel) / 8;
var stride = bytesPerPixel * cf.FrameDescription.Width;
bmpSource = BitmapSource.Create(fd.Width, fd.Height, 96.0, 96.0, PixelFormats.Bgr32, null, colorData, stride);
// WritableBitmap to show on UI
wbmp = new WriteableBitmap(bmpSource);
FacePhoto.Source = wbmp;
}
}
private void SaveImage(BitmapSource image)
{
try
{
FileStream stream = new System.IO.FileStream(@"Frames\frame.jpg", System.IO.FileMode.OpenOrCreate);
JpegBitmapEncoder encoder = new JpegBitmapEncoder();
encoder.FlipHorizontal = true;
encoder.FlipVertical = false;
encoder.QualityLevel = 30;
encoder.Frames.Add(BitmapFrame.Create(image));
encoder.Save(stream);
stream.Close();
}
catch (Exception)
{
}
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
timer = new DispatcherTimer { Interval = TimeSpan.FromSeconds(2) };
timer.Tick += Timer_Tick;
timer.Start();
timer2 = new DispatcherTimer { Interval = TimeSpan.FromSeconds(5) };
timer2.Tick += Timer2_Tick;
timer2.Start();
}
private void Timer_Tick(object sender, EventArgs e)
{
SaveImage(bmpSource);
}
private async void Timer2_Tick(object sender, EventArgs e)
{
Title = "Detecting...";
FaceRectangle[] faceRects = await UploadAndDetectFaces(streamF);
Face[] faceAttributes = await UploadAndDetectFaceAttributes(streamF);
Title = String.Format("Detection Finished. {0} face(s) detected", faceRects.Length);
if (faceRects.Length > 0)
{
DrawingVisual visual = new DrawingVisual();
DrawingContext drawingContext = visual.RenderOpen();
drawingContext.DrawImage(bmpSource,
new Rect(0, 0, bmpSource.Width, bmpSource.Height));
double dpi = bmpSource.DpiX;
double resizeFactor = 96 / dpi;
foreach (var faceRect in faceRects)
{
drawingContext.DrawRectangle(
Brushes.Transparent,
new Pen(Brushes.Red, 2),
new Rect(
faceRect.Left * resizeFactor,
faceRect.Top * resizeFactor,
faceRect.Width * resizeFactor,
faceRect.Height * resizeFactor
)
);
}
drawingContext.Close();
RenderTargetBitmap faceWithRectBitmap = new RenderTargetBitmap(
(int)(bmpSource.PixelWidth * resizeFactor),
(int)(bmpSource.PixelHeight * resizeFactor),
96,
96,
PixelFormats.Pbgra32);
faceWithRectBitmap.Render(visual);
FacePhoto.Source = faceWithRectBitmap;
}
if (faceAttributes.Length > 0)
{
foreach (var faceAttr in faceAttributes)
{
Label lb = new Label();
//Canvas.SetLeft(lb, lb.Width);
lb.Content = faceAttr.FaceAttributes.Gender;// + " " + faceAttr.Gender + " " + faceAttr.FacialHair + " " + faceAttr.Glasses + " " + faceAttr.HeadPose + " " + faceAttr.Smile;
lb.FontSize = 50;
lb.Width = 200;
lb.Height = 100;
stack.Children.Add(lb);
}
}
}
private async Task<FaceRectangle[]> UploadAndDetectFaces(string imageFilePath)
{
try
{
using (Stream imageFileStream = File.OpenRead(imageFilePath))
{
var faces = await faceServiceClient.DetectAsync(imageFilePath);
var faceRects = faces.Select(face => face.FaceRectangle);
var faceAttrib = faces.Select(face => face.FaceAttributes);
return faceRects.ToArray();
}
}
catch (Exception)
{
return new FaceRectangle[0];
}
}
private async Task<Face[]> UploadAndDetectFaceAttributes(string imageFilePath)
{
try
{
using (Stream imageFileStream = File.Open(imageFilePath, FileMode.Open, FileAccess.Read, FileShare.ReadWrite))
{
var faces = await faceServiceClient.DetectAsync(imageFileStream, true, true, new FaceAttributeType[] { FaceAttributeType.Gender, FaceAttributeType.Age, FaceAttributeType.Smile, FaceAttributeType.Glasses, FaceAttributeType.HeadPose, FaceAttributeType.FacialHair });
return faces.ToArray();
}
}
catch (Exception)
{
return new Face[0];
}
}
}
上面的代碼運行良好。 但是,我想將 Kinect Color Feed 的每一幀直接轉換為 Stream,雖然我搜索過但我不知道該怎么做,但沒有任何效果。 如果有人可以幫助我,那就太好了。 謝謝!
您可以將幀持久化到MemoryStream
,倒帶它(通過調用Position = 0
),然后將該流發送到DetectAsync()
,而不是將幀持久化到SaveImage
的文件。
另請注意,在UploadAndDetectFaces
,您應該將imageFileStream
而不是imageFilePath
發送到DetectAsync()
。 無論如何,您可能不想同時調用UploadAndDetectFaces
和UploadAndDetectFaceAttributes
,因為您只是將工作翻了一番(並且達到了配額/速率限制。)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.