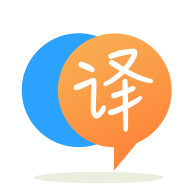
[英]DataTrigger binded to property in ViewModel is not fired in the Button of DataGrid
[英]Multiselect Listview with select all check box. Datatrigger on binded property
我有一個帶有兩列的網格視圖的列表視圖。 第一列包含綁定到listviewitem selected屬性的復選框,第二列是文本。 在復選框列的標題中,我有一個復選框,我想用作全選/取消全選按鈕。 我已經使用數據觸發器來執行此操作,但是僅當我刪除復選框和selected屬性之間的綁定時,它才起作用。 我應該能夠使用數據觸發器來設置綁定屬性嗎?
代碼隱藏
namespace ListviewWCheckboxes
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
List<string> listItems = new List<string>() { "foo", "bar", "blah" };
public MainWindow()
{
InitializeComponent();
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
pdflistView.ItemsSource = listItems;
}
}
}
XAML
<Window x:Class="ListviewWCheckboxes.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:ListviewWCheckboxes"
mc:Ignorable="d"
Title="MainWindow" Height="350" Width="525"
Loaded="Window_Loaded">
<Grid>
<ListView x:Name="pdflistView" HorizontalAlignment="Left" Height="300" Margin="5" VerticalAlignment="Top" Width="240"
SelectionMode="Extended"
>
<ListView.View>
<GridView>
<GridViewColumn>
<GridViewColumn.Header>
<CheckBox x:Name="ckbxSelectAll"/>
</GridViewColumn.Header>
<GridViewColumn.CellTemplate>
<DataTemplate>
<CheckBox IsChecked="{Binding Path=IsSelected,
RelativeSource={RelativeSource AncestorType={x:Type ListViewItem}, Mode=FindAncestor},Mode=TwoWay,UpdateSourceTrigger=PropertyChanged}">
<CheckBox.Style>
<Style TargetType="CheckBox">
<Style.Triggers>
<DataTrigger Binding="{Binding ElementName=ckbxSelectAll, Path=IsChecked}" Value="True">
<Setter Property="IsChecked" Value="True" />
</DataTrigger>
<DataTrigger Binding="{Binding ElementName=ckbxSelectAll, Path=IsChecked}" Value="False">
<Setter Property="IsChecked" Value="False" />
</DataTrigger>
</Style.Triggers>
</Style>
</CheckBox.Style>
</CheckBox>
<DataTemplate.Triggers>
</DataTemplate.Triggers>
</DataTemplate>
</GridViewColumn.CellTemplate>
</GridViewColumn>
<GridViewColumn Header="Pdf" DisplayMemberBinding="{Binding}"/>
</GridView>
</ListView.View>
</ListView>
</Grid>
</Window>
根據AjS的建議,我提出了此解決方案。
我還能夠提出一種解決方案,該解決方案可以深入挖掘列表框的可視樹,直到獲得包含所有ListviewItems的VirtualizingStackPanel並通過設置IsSelected屬性遍歷它們為止,但這似乎更干凈。
XAML
<Window x:Class="ListviewWCheckboxes.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:ListviewWCheckboxes"
mc:Ignorable="d"
Title="MainWindow" Height="350" Width="525">
<Grid>
<ListView x:Name="pdflistView" HorizontalAlignment="Left" Height="300" Margin="5" VerticalAlignment="Top" Width="240"
SelectionMode="Extended" ItemsSource="{Binding Path=listItems}">
<ListView.ItemContainerStyle>
<Style TargetType="ListViewItem">
<Setter Property="IsSelected" Value="{Binding is_Selected}"/>
</Style>
</ListView.ItemContainerStyle>
<ListView.View>
<GridView>
<GridViewColumn>
<GridViewColumn.Header>
<CheckBox x:Name="ckbxSelectAll" Checked="ckbxSelectAll_Checked" Unchecked="ckbxSelectAll_Checked"/>
</GridViewColumn.Header>
<GridViewColumn.CellTemplate>
<DataTemplate>
<CheckBox IsChecked="{Binding is_Selected}"/>
</DataTemplate>
</GridViewColumn.CellTemplate>
</GridViewColumn>
<GridViewColumn Header="Pdf" DisplayMemberBinding="{Binding Path=text}"/>
</GridView>
</ListView.View>
</ListView>
</Grid>
</Window>
碼
using System.Collections.ObjectModel;
using System.ComponentModel;
using System.Runtime.CompilerServices;
using System.Windows;
using System.Windows.Controls;
namespace ListviewWCheckboxes
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public ObservableCollection<someobjects> listItems { get; set; } = new ObservableCollection<someobjects>();
public MainWindow()
{
InitializeComponent();
this.DataContext = this;
listItems.Add(new someobjects("foo"));
listItems.Add(new someobjects("bar"));
listItems.Add(new someobjects("blah"));
}
private void ckbxSelectAll_Checked(object sender, RoutedEventArgs e)
{
foreach (someobjects item in listItems)
{
item.is_Selected = (bool)((CheckBox)sender).IsChecked;
}
}
public class someobjects : INotifyPropertyChanged
{
private string _text;
public string text
{
get { return _text; }
set { _text = value; OnPropertyChanged(); }
}
private bool _is_Selected;
public bool is_Selected
{
get { return _is_Selected; }
set { _is_Selected = value; OnPropertyChanged(); }
}
public someobjects(string t)
{
text = t;
is_Selected = false;
}
public event PropertyChangedEventHandler PropertyChanged;
protected void OnPropertyChanged([CallerMemberName] string propertyName = "")
{
var handler = PropertyChanged;
if (handler != null)
handler(this, new PropertyChangedEventArgs(propertyName));
}
}
}
}
對於想知道我提到的第二個解決方案的任何人,此事件都必須附加到selectall復選框的選中和未選中事件
private void ckbxSelectAll_Checked(object sender, RoutedEventArgs e)
{
DockPanel dp = FindVisualChild<DockPanel>(pdflistView, 0);
ScrollContentPresenter scp = FindVisualChild<ScrollContentPresenter>(dp, 1);
VirtualizingStackPanel vsp = FindVisualChild<VirtualizingStackPanel>(scp, 0);
foreach (ListViewItem item in vsp.Children)
{
item.IsSelected = (bool)((CheckBox)sender).IsChecked;
}
}
private static T FindVisualChild<T>(UIElement element, int childindex) where T : UIElement
{
UIElement child = element;
while (child != null)
{
T correctlyTyped = child as T;
if (correctlyTyped != null)
{
return correctlyTyped;
}
child = VisualTreeHelper.GetChild(child, childindex) as UIElement;
}
return null;
}
我相信實現此目的的最佳方法是在類上創建一個屬性“ IsSelected”,並將其綁定到復選框(IsChecked)和列表視圖(isSelected)。 處理標題復選框的選中事件,並手動切換集合中各項的IsSelected屬性,以便更新復選框和listviewitems。 如果您還有其他疑問,請告訴我
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.