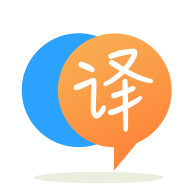
[英]FileHelpers: How to skip first and last line reading fixed width text
[英]Reading the 2 last line from a text
我是c#的新手,我正在開發一個應用程序,它顯示文本文件最后兩行上兩個日期的時差。
我想從文件文本中讀取前一行,我已經知道如何讀取最后一行,但我需要閱讀前一行。
這是我的代碼:
var lastLine = File.ReadAllLines("C:\\test.log").Last();
richTextBox1.Text = lastLine.ToString();
以來
File.ReadAllLines("C:\\test.log");
返回一個數組,你可以采取數組的最后兩項:
var data = File.ReadAllLines("C:\\test.log");
string last = data[data.Length - 1];
string lastButOne = data[data.Length - 2];
在一般情況下,使用長文件(這就是為什么ReadAllLines
是一個糟糕的選擇),你可以實現
public static partial class EnumerableExtensions {
public static IEnumerable<T> Tail<T>(this IEnumerable<T> source, int count) {
if (null == source)
throw new ArgumentNullException("source");
else if (count < 0)
throw new ArgumentOutOfRangeException("count");
else if (0 == count)
yield break;
Queue<T> queue = new Queue<T>(count + 1);
foreach (var item in source) {
queue.Enqueue(item);
if (queue.Count > count)
queue.Dequeue();
}
foreach (var item in queue)
yield return item;
}
}
...
var lastTwolines = File
.ReadLines("C:\\test.log") // Not all lines
.Tail(2);
你可以嘗試這樣做
var lastLines = File.ReadAllLines("C:\\test.log").Reverse().Take(2).Reverse();
但是根據文件的大小,有可能比一次讀取所有行更有效的方法來處理它。 請參閱獲取最后10行非常大的文本文件> 10GB以及如何讀取日志文件的最后“n”行
只需將ReadAllLines
的結果存儲到一個變量中,然后取兩個最后的變量:
var allText = File.ReadAllLines("C:\\test.log");
var lastLines = allText.Skip(allText.Length - 2);
在返回請求的最后一行之前,所有先前的答案都急切地將所有文件加載到內存中。 如果文件很大,這可能是一個問題。 幸運的是,它很容易避免。
public static IEnumerable<string> ReadLastLines(string path, int count)
{
if (count < 1)
return Enumerable.Empty<string>();
var queue = new Queue<string>(count);
foreach (var line in File.ReadLines(path))
{
if (queue.Count == count)
queue.Dequeue();
queue.Enqueue(line);
}
return queue;
}
這將只保留內存中最后n
讀取行,避免了大文件的內存問題。
您可以使用Skip()
和Take()
類的
var lastLine = File.ReadAllLines("C:\\test.log");
var data = lastLine.Skip(lastLine.Length - 2);
richTextBox1.Text = lastLine.ToString();
您可以在Queue<string>
的組合中使用StreamReader
,因為您必須以任一方式讀取整個文件。
// if you want to read more lines change this to the ammount of lines you want
const int LINES_KEPT = 2;
Queue<string> meQueue = new Queue<string>();
using ( StreamReader reader = new StreamReader(File.OpenRead("C:\\test.log")) )
{
string line = string.Empty;
while ( ( line = reader.ReadLine() ) != null )
{
if ( meQueue.Count == LINES_KEPT )
meQueue.Dequeue();
meQueue.Enqueue(line);
}
}
現在你可以像這樣使用這兩行:
string line1 = meQueue.Dequeue();
string line2 = meQueue.Dequeue(); // <-- this is the last line.
或者將其添加到RichTextBox
:
richTextBox1.Text = string.Empty; // clear the text
while ( meQueue.Count != 0 )
{
richTextBox1.Text += meQueue.Dequeue(); // add all lines in the same order as they were in file
}
使用File.ReadAllLines
將讀取整個文本,然后使用Linq
將迭代已經的紅線。 此方法一次完成所有操作。
string line;
string[] lines = new string[]{"",""};
int index = 0;
using ( StreamReader reader = new StreamReader(File.OpenRead("C:\\test.log")) )
{
while ( ( line = reader.ReadLine() ) != null )
{
lines[index] = line;
index = 1-index;
}
}
// Last Line -1 = lines[index]
// Last line = lines[1-index]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.