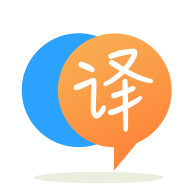
[英]Dot appended to the end of MQ message when passing it from C# .Net application to IBM MQ
[英]C#: IBM MQ 'unlock' message when using Read() method
我的代碼:
//Initialize MQMessage
MQMessage message = new MQMessage();
//Initialize WebMQConnection
WebSphereMQConnection mqRequestConnection = new WebSphereMQConnection(initQMName, initQChannel, initQConnection, initQName, string.Empty, string.Empty);
mqRequestConnection.Open();
mqRequestConnection.Read(message);
//Get the contents as a string
string body = message.ReadString(message.MessageLength);
return body;
此代碼是控制台應用程序的一部分,可按預期順序瀏覽隊列並讀取每條消息。 它從平面文件中解析字符串內容。
但是,似乎Read()方法也會鎖定消息,直到程序關閉。 即使我在一個循環中運行程序來順序讀取所有消息,它似乎也沒有“釋放”消息,直到程序完全關閉。
我已經嘗試了Get and Puts,Dispose,Backout等等,除了停止整個控制台應用程序的執行之外,似乎沒有任何工作。
//Initialize WebMQConnection
WebSphereMQConnection mqRequestConnection = new WebSphereMQConnection(initQMName, initQChannel, initQConnection, initQName, string.Empty, string.Empty);
mqRequestConnection.Open();
mqRequestConnection.Read(message);
這是世界上的什么? 它當然不是IBM MQ類和方法。 所以,這是一個本土的班級。
因此,Read()方法實際上執行'瀏覽'。 命名不好的方法。 我敢打賭,在你的Read()方法中,它使用MQGET上的MQGMO_LOCK選項。
為什么不擺脫WebSphereMQConnection類並只編寫純IBM MQ .NET代碼?
這是一個MQ CS .NET示例程序,用於在沒有任何消息鎖定的情況下瀏覽隊列:
using System;
using IBM.WMQ;
/// <summary> Program Name
/// MQTest62B
///
/// Description
/// This C# class will connect to a remote queue manager
/// and get (browse) a message from a queue using a managed .NET environment.
///
/// Sample Command Line Parameters
/// -h 127.0.0.1 -p 1415 -c TEST.CHL -m MQWT1 -q TEST.Q1
/// </summary>
/// <author> Roger Lacroix, Capitalware Inc.
/// </author>
namespace MQTest62
{
public class MQTest62B
{
private System.Collections.Hashtable inParms = null;
private System.String qManager;
private System.String outputQName;
private System.String userID = "tester";
private System.String password = "barney";
/*
* The constructor
*/
public MQTest62B()
: base()
{
}
/// <summary> Make sure the required parameters are present.</summary>
/// <returns> true/false
/// </returns>
private bool allParamsPresent()
{
bool b = inParms.ContainsKey("-h") && inParms.ContainsKey("-p") &&
inParms.ContainsKey("-c") && inParms.ContainsKey("-m") &&
inParms.ContainsKey("-q");
if (b)
{
try
{
System.Int32.Parse((System.String)inParms["-p"]);
}
catch (System.FormatException e)
{
b = false;
}
}
return b;
}
/// <summary> Extract the command-line parameters and initialize the MQ variables.</summary>
/// <param name="args">
/// </param>
/// <throws> IllegalArgumentException </throws>
private void init(System.String[] args)
{
inParms = System.Collections.Hashtable.Synchronized(new System.Collections.Hashtable(14));
if (args.Length > 0 && (args.Length % 2) == 0)
{
for (int i = 0; i < args.Length; i += 2)
{
inParms[args[i]] = args[i + 1];
}
}
else
{
throw new System.ArgumentException();
}
if (allParamsPresent())
{
qManager = ((System.String)inParms["-m"]);
outputQName = ((System.String)inParms["-q"]);
// Set up MQ environment
MQEnvironment.Hostname = ((System.String)inParms["-h"]);
MQEnvironment.Channel = ((System.String)inParms["-c"]);
try
{
MQEnvironment.Port = System.Int32.Parse((System.String)inParms["-p"]);
}
catch (System.FormatException e)
{
MQEnvironment.Port = 1414;
}
if (userID != null)
MQEnvironment.UserId = userID;
if (password != null)
MQEnvironment.Password = password;
}
else
{
throw new System.ArgumentException();
}
}
/// <summary> Connect, open queue, read (browse) a message, close queue and disconnect. </summary>
///
private void testReceive()
{
MQQueueManager qMgr = null;
MQQueue inQ = null;
int openOptions = MQC.MQOO_BROWSE + MQC.MQOO_FAIL_IF_QUIESCING;
try
{
qMgr = new MQQueueManager(qManager);
System.Console.Out.WriteLine("MQTest62B successfully connected to " + qManager);
inQ = qMgr.AccessQueue(outputQName, openOptions, null, null, null); // no alternate user id
System.Console.Out.WriteLine("MQTest62B successfully opened " + outputQName);
testLoop(inQ);
}
catch (MQException mqex)
{
System.Console.Out.WriteLine("MQTest62B cc=" + mqex.CompletionCode + " : rc=" + mqex.ReasonCode);
}
catch (System.IO.IOException ioex)
{
System.Console.Out.WriteLine("MQTest62B ioex=" + ioex);
}
finally
{
try
{
if (inQ != null)
inQ.Close();
System.Console.Out.WriteLine("MQTest62B closed: " + outputQName);
}
catch (MQException mqex)
{
System.Console.Out.WriteLine("MQTest62B cc=" + mqex.CompletionCode + " : rc=" + mqex.ReasonCode);
}
try
{
if (qMgr != null)
qMgr.Disconnect();
System.Console.Out.WriteLine("MQTest62B disconnected from " + qManager);
}
catch (MQException mqex)
{
System.Console.Out.WriteLine("MQTest62B cc=" + mqex.CompletionCode + " : rc=" + mqex.ReasonCode);
}
}
}
private void testLoop(MQQueue inQ)
{
bool flag = true;
MQMessage msg = new MQMessage();
MQGetMessageOptions gmo = new MQGetMessageOptions();
gmo.Options |= MQC.MQGMO_BROWSE_NEXT | MQC.MQGMO_WAIT | MQC.MQGMO_FAIL_IF_QUIESCING;
gmo.WaitInterval = 500; // 1/2 second wait time or MQC.MQEI_UNLIMITED
while (flag)
{
try
{
msg = new MQMessage();
inQ.Get(msg, gmo);
System.Console.Out.WriteLine("Message Data: " + msg.ReadString(msg.MessageLength));
}
catch (MQException mqex)
{
System.Console.Out.WriteLine("MQTest62B CC=" + mqex.CompletionCode + " : RC=" + mqex.ReasonCode);
if (mqex.Reason == MQC.MQRC_NO_MSG_AVAILABLE)
{
// no meesage - life is good - loop again
}
else
{
flag = false; // severe error - time to exit
}
}
catch (System.IO.IOException ioex)
{
System.Console.Out.WriteLine("MQTest62B ioex=" + ioex);
}
}
}
/// <summary> main line</summary>
/// <param name="args">
/// </param>
// [STAThread]
public static void Main(System.String[] args)
{
MQTest62B write = new MQTest62B();
try
{
write.init(args);
write.testReceive();
}
catch (System.ArgumentException e)
{
System.Console.Out.WriteLine("Usage: MQTest62B -h host -p port -c channel -m QueueManagerName -q QueueName");
System.Environment.Exit(1);
}
catch (MQException e)
{
System.Console.Out.WriteLine(e);
System.Environment.Exit(1);
}
System.Environment.Exit(0);
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.