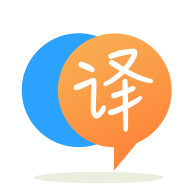
[英]how to clean datagridview in C# - but stay with the originals columns header
[英]C# DataGridView: combine multiple rows to one but keep originals
首先:一旦我完成,我將自己提供這個問題的答案。
但是你可以在我的路上幫助我,我會很感激你的所有建議。
我有一個DataGridView與不同的行屬於一起。 我的問題是找到一種合適的方式來顯示和使用這些連接的行。
我的第一個想法是保持每一行的個性,但這有一些缺點:
如何清楚地顯示行屬於一起?
我可以添加另一個列,其單元格顯示連接行的相同數字,但這不容易看到,需要另一列。
我的解決方案是所有連接的行具有相同的背景顏色,並且對於每組連接的行,顏色例如在白色和淺灰色之間變化。
如何使用連接的行? 一旦我選擇了一行的一行,我就必須通過提取哪些行屬於一起的信息(保存在單元格的標簽或隱藏的單元格中)來分析這一行,並選擇它們。 在DataGridView中向上/向下移動行的工作量更大:我還需要分析相鄰的行集以查看我必須移動多遠。
因此我決定創建一個DataGridViewMultiRow
。
完成之后,我將在這里發布該類的完整代碼作為答案。
它將繼承DataGridViewRow
(“DGVR”)並存儲單個DGVR或其他多行的列表,並通過自己的代碼繪制行的單元格將它們顯示為一個。 但是,我仍然需要找出用於此目的的事件。 MSDN建議使用DataGridView.RowPrePaint
,但我更想使用綁定到DGVR本身的事件。 也許我會分析DataGridViewRow.Paint()的源代碼並編寫我自己的方法......
當添加到多行時,單行將變為不可見(它們可以通過濫用概念再次切換到可見,但.net本身有很多不受濫用的保護;也許我甚至不切換看不見,所以這是用戶的責任)。
通過強制每個DGVR成為與多行相同的DGV的一部分,可以簡單地避免多行中的遞歸,並且因為每行只能添加到一個DGV並且只能添加一次,所以我不必檢查遞歸了。
現在我正在努力如何實現內部行列表。 我正在考慮使用.net DataGridViewRowCollection
,但我發現它的操作與DataGridView
本身緊密綁定:DGV只能有一個DGVRColl,但每個DGVRColl都指的是DGV。 因此,我的每個DGVMultiRow中都會有半連接的DGVRColl。
我打算問這是否會引起問題,但我已經發現在實例化DGVRColl時我必須提供一個DGV,這是DGVMultiRow ctor被調用的那一刻我沒有的。 此外,當使用DGVRColl並為其提供公共get屬性時,我只能掛鈎'CollectionChanged'事件並且無法控制各個操作,如Add()
和Remove()
。 所以我將使用一個簡單的私人列表。
繼續#1
我完成了主要工作,看起來已經很不錯了:
我仍然需要修復細節,比如在移動滾動條和其他小東西時正確放置文本。
我決定不覆蓋DataGridViewRow.Paint()
因為那個內部連接太多了。 所以我第一次使用CellPainting
事件,這是一個良好的開端。 但我需要同時擁有該行的所有單元格的信息,所以我按照MSDN的建議繼續覆蓋DataGridView.RowPrePaint()
,請參閱上面的鏈接。 這非常有效。
未完待續。
經過各種弊端,我終於創造了一個解決方案。
它是用C ++ / CLI編寫的,因此大多數人都必須調整它以便在C#中使用。
此解決方案包含一些不屬於解決方案的用戶功能,但其目的應該易於通過其名稱進行猜測。
#pragma once
using namespace System;
using namespace System::Collections::Generic;
using namespace System::Drawing;
using namespace System::Windows::Forms;
using namespace System::ComponentModel;
public ref class CDataGridViewMultiRow : DataGridViewRow
{
public:
//----------------------------------------------------------------------------
// constructor
//----------------------------------------------------------------------------
CDataGridViewMultiRow ();
CDataGridViewMultiRow (bool i_bHideRows);
CDataGridViewMultiRow (bool i_bHideRows, ::DataGridView^ i_dgv);
//----------------------------------------------------------------------------
// Clone
//----------------------------------------------------------------------------
virtual Object^ Clone () override;
//----------------------------------------------------------------------------
// Clear
//----------------------------------------------------------------------------
void Clear ();
//----------------------------------------------------------------------------
// Add, Insert
//----------------------------------------------------------------------------
bool Add (DataGridViewRow^ i_dgvr);
bool Insert (int i_ixRow, DataGridViewRow^ i_dgvr);
//----------------------------------------------------------------------------
// Remove
//----------------------------------------------------------------------------
bool Remove (int i_ixRow);
bool Remove (DataGridViewRow^ i_dgvr);
//----------------------------------------------------------------------------
// Update
//----------------------------------------------------------------------------
void Update ();
//----------------------------------------------------------------------------
// PaintRow
//
// description: manually paints the row.
//
// !!! IMPORTANT NOTICE: !!!
// This method must be attached to the DataGridView's RowPrePaint event.
//----------------------------------------------------------------------------
static void PaintRow (Object^ sender, DataGridViewRowPrePaintEventArgs^ e);
//----------------------------------------------------------------------------
// properties
//----------------------------------------------------------------------------
property DataGridViewRow^ Rows[int] { DataGridViewRow^ get (int i_ixRow);
void set (int i_ixRow, DataGridViewRow^ i_dgvr); }
property int RowCount { int get() { return m_listdgvr->Count;} }
property bool HideRows { bool get() { return m_bHideRows;}
void set(bool i_bHideRows); }
public:
protected:
List<DataGridViewRow^>^ m_listdgvr;
bool m_bHideRows;
private:
protected:
virtual void OnDataGridViewChanged () override;
private:
void CommonConstructor (bool i_bHideRows,
::DataGridView^ i_dgv);
};
#include "CDataGridViewMultiRow.h"
using namespace Schmoll_SwCore;
//----------------------------------------------------------------------------
// constructor
//----------------------------------------------------------------------------
CDataGridViewMultiRow::CDataGridViewMultiRow () : DataGridViewRow ()
{
CommonConstructor (false, nullptr);
}
//----------------------------------------------------------------------------
CDataGridViewMultiRow::CDataGridViewMultiRow (bool i_bHideRows) : DataGridViewRow ()
{
CommonConstructor (i_bHideRows, nullptr);
}
//----------------------------------------------------------------------------
CDataGridViewMultiRow::CDataGridViewMultiRow (bool i_bHideRows, ::DataGridView^ i_dgv) : DataGridViewRow ()
{
CommonConstructor (i_bHideRows, i_dgv);
}
//----------------------------------------------------------------------------
// property: Rows
//----------------------------------------------------------------------------
DataGridViewRow^ CDataGridViewMultiRow::Rows::get (int i_ixRow)
{
if (i_ixRow < 0 || i_ixRow >= m_listdgvr->Count)
return nullptr;
return m_listdgvr[i_ixRow];
}
//----------------------------------------------------------------------------
void CDataGridViewMultiRow::Rows::set (int i_ixRow, DataGridViewRow^ i_dgvr)
{
if (!i_dgvr)
return;
if (i_ixRow < 0 || i_ixRow >= m_listdgvr->Count)
return;
int ixDgvr = -1;
DataGridViewRow^ dgvr = m_listdgvr[i_ixRow];
if (dgvr->DataGridView
&& dgvr->DataGridView == this->DataGridView)
{
ixDgvr = dgvr->Index;
dgvr->DataGridView->Rows->Remove (dgvr);
}
m_listdgvr[i_ixRow] = i_dgvr;
if (this->DataGridView)
{
if (ixDgvr < 0)
ixDgvr = this->DataGridView->Rows->IndexOf (this) + 1 + i_ixRow;
this->DataGridView->Rows->Insert (ixDgvr, i_dgvr);
i_dgvr->Visible = !m_bHideRows;
}
Update();
}
//----------------------------------------------------------------------------
// property: HideRows
//----------------------------------------------------------------------------
void CDataGridViewMultiRow::HideRows::set (bool i_bHideRows)
{
m_bHideRows = i_bHideRows;
for (int ixRow = 0; ixRow < m_listdgvr->Count; ixRow++)
m_listdgvr[ixRow]->Visible = !m_bHideRows;
}
//----------------------------------------------------------------------------
// Clone
//----------------------------------------------------------------------------
Object^ CDataGridViewMultiRow::Clone ()
{
CDataGridViewMultiRow^ dgvr = (CDataGridViewMultiRow^)DataGridViewRow::Clone();
if (dgvr)
{
dgvr->m_bHideRows = this->m_bHideRows;
dgvr->m_listdgvr->Clear();
dgvr->m_listdgvr->AddRange (this->m_listdgvr);
}
return dgvr;
}
//----------------------------------------------------------------------------
// Clear
//----------------------------------------------------------------------------
void CDataGridViewMultiRow::Clear ()
{
for (int ixRow = 0; ixRow < m_listdgvr->Count; ixRow++)
{
if (m_listdgvr[ixRow]->DataGridView
&& m_listdgvr[ixRow]->DataGridView == this->DataGridView)
m_listdgvr[ixRow]->DataGridView->Rows->Remove (m_listdgvr[ixRow]);
m_listdgvr[ixRow]->Visible = true;
}
m_listdgvr->Clear();
Update();
}
//----------------------------------------------------------------------------
// Add
//----------------------------------------------------------------------------
bool CDataGridViewMultiRow::Add (DataGridViewRow^ i_dgvr)
{
return Insert (m_listdgvr->Count, i_dgvr);
}
//----------------------------------------------------------------------------
// Insert
//----------------------------------------------------------------------------
bool CDataGridViewMultiRow::Insert (int i_ixRow, DataGridViewRow^ i_dgvr)
{
if (!i_dgvr)
return false;
if (i_dgvr->Index < 0)
return false; // block shared rows and rows that are not part of a DGV
if (i_ixRow < 0)
return false;
else if (i_ixRow > m_listdgvr->Count)
i_ixRow = m_listdgvr->Count;
m_listdgvr->Insert (i_ixRow, i_dgvr);
if (i_dgvr->DataGridView
&& i_dgvr->DataGridView != this->DataGridView)
i_dgvr->DataGridView->Rows->Remove (i_dgvr);
if (this->DataGridView)
{
int ixDgvr = this->DataGridView->Rows->IndexOf (this) + 1 + i_ixRow;
if (i_dgvr->DataGridView == this->DataGridView
&& i_dgvr->Index != ixDgvr)
i_dgvr->DataGridView->Rows->Remove (i_dgvr);
ixDgvr = this->DataGridView->Rows->IndexOf (this) + 1 + i_ixRow;
if (i_dgvr->DataGridView != this->DataGridView)
this->DataGridView->Rows->Insert (ixDgvr, i_dgvr);
}
i_dgvr->Visible = !m_bHideRows;
Update();
return true;
}
//----------------------------------------------------------------------------
// Remove
//----------------------------------------------------------------------------
bool CDataGridViewMultiRow::Remove (int i_ixRow)
{
return Remove (Rows[i_ixRow]);
}
//----------------------------------------------------------------------------
// Remove
//----------------------------------------------------------------------------
bool CDataGridViewMultiRow::Remove (DataGridViewRow^ i_dgvr)
{
bool bResult = m_listdgvr->Remove (i_dgvr);
if (i_dgvr)
{
if (i_dgvr->DataGridView
&& i_dgvr->DataGridView == this->DataGridView)
i_dgvr->DataGridView->Rows->Remove (i_dgvr);
i_dgvr->Visible = true;
}
Update();
return bResult;
}
//----------------------------------------------------------------------------
// Update
//----------------------------------------------------------------------------
void CDataGridViewMultiRow::Update ()
{
if (!this->DataGridView)
return;
if (this->Index < 0)
throw gcnew InvalidOperationException ("Index is < 0. This may happen if the row was created by CreateCells(), then added to a DGV, which made a previously shared row become unshared, and then being accessed by the same invalidated object. Get the updated row object from the DGV.");
array<int>^ aiNewLines = gcnew array<int>(m_listdgvr->Count);
array<String^, 2>^ a2sValue = gcnew array<String^, 2>(this->Cells->Count, m_listdgvr->Count);
for (int ixCell = 0; ixCell < Cells->Count; ixCell++)
{
for (int ixRow = 0; ixRow < m_listdgvr->Count; ixRow++)
{
if (m_listdgvr[ixRow]->Index < 0)
continue;
Object^ oValue = m_listdgvr[ixRow]->Cells[ixCell]->Value;
if (oValue)
{
a2sValue[ixCell, ixRow] = oValue->ToString();
int iNewLines = CString::Count (a2sValue[ixCell, ixRow], CONSTS::CRLF, StringComparison::InvariantCultureIgnoreCase);
aiNewLines[ixRow] = Math::Max (aiNewLines[ixRow], iNewLines);
}
}
}
for (int ixCell = 0; ixCell < Cells->Count; ixCell++)
{
String^ sText = nullptr;
for (int ixRow = 0; ixRow < m_listdgvr->Count; ixRow++)
{
if (ixRow > 0)
sText += CONSTS::CRLF;
sText += a2sValue[ixCell, ixRow];
int iNewLines = CString::Count (a2sValue[ixCell, ixRow], CONSTS::CRLF, StringComparison::InvariantCultureIgnoreCase);
sText += CString::Repeat (CONSTS::CRLF, aiNewLines[ixRow] - iNewLines);
}
this->Cells[ixCell]->Value = sText;
}
}
//----------------------------------------------------------------------------
// OnDataGridViewChanged
//----------------------------------------------------------------------------
void CDataGridViewMultiRow::OnDataGridViewChanged ()
{
try
{
if (this->DataGridView)
{
int ixDgvr = this->DataGridView->Rows->IndexOf (this) + 1;
for (int ixCnt = 0; ixCnt < m_listdgvr->Count; ixCnt++)
DataGridView->Rows->Insert (ixDgvr + ixCnt, m_listdgvr[ixCnt]);
}
else
{
for (int ixCnt = 0; ixCnt < m_listdgvr->Count; ixCnt++)
m_listdgvr[ixCnt]->DataGridView->Rows->Remove (m_listdgvr[ixCnt]);
}
}
finally
{
DataGridViewRow::OnDataGridViewChanged();
}
}
//----------------------------------------------------------------------------
// PaintRow
//----------------------------------------------------------------------------
void CDataGridViewMultiRow::PaintRow (Object^ sender, DataGridViewRowPrePaintEventArgs^ e)
{
::DataGridView^ dgv = dynamic_cast<::DataGridView^>(sender);
if (!dgv)
return;
if (e->RowIndex < 0 || e->RowIndex >= dgv->RowCount)
return;
CDataGridViewMultiRow^ dgvmr = dynamic_cast<CDataGridViewMultiRow^>(dgv->Rows->SharedRow(e->RowIndex));
if (!dgvmr)
return;
if (dgvmr->DataGridView != dgv)
return;
bool bAutoHeight = dgv->AutoSizeRowsMode == DataGridViewAutoSizeRowsMode::AllCells
|| dgv->AutoSizeRowsMode == DataGridViewAutoSizeRowsMode::AllCellsExceptHeaders
|| dgv->AutoSizeRowsMode == DataGridViewAutoSizeRowsMode::DisplayedCells
|| dgv->AutoSizeRowsMode == DataGridViewAutoSizeRowsMode::DisplayedCellsExceptHeaders;
Graphics^ g = e->Graphics;
StringFormatFlags enFlags = (StringFormatFlags)0;
if (dgvmr->InheritedStyle->WrapMode != DataGridViewTriState::True)
enFlags = enFlags | StringFormatFlags::NoWrap;
StringFormat^ oStringFormat = gcnew StringFormat(enFlags);
array<float>^ afRowHeight = gcnew array<float>(dgvmr->RowCount);
array<int>^ aiLines = gcnew array<int> (dgvmr->RowCount);
array<int, 2>^ a2iLines = gcnew array<int, 2>(dgvmr->Cells->Count, dgvmr->RowCount);
array<String^, 2>^ a2sValue = gcnew array<String^, 2>(dgvmr->Cells->Count, dgvmr->RowCount);
for (int ixRow = 0; ixRow < dgvmr->RowCount; ixRow++)
{
DataGridViewRow^ dgvr = dgvmr->Rows[ixRow];
for (int ixCell = 0; ixCell < dgvmr->Cells->Count; ixCell++)
{
if (dgvr->Index < 0)
continue;
Object^ oValue = dgvr->Cells[ixCell]->Value;
if (!oValue)
continue;
a2sValue[ixCell, ixRow] = oValue->ToString();
int iCharacters = 0, iLines = 0;
SizeF oLayoutArea ((float)dgvmr->Cells[ixCell]->Size.Width, 0);
SizeF oTextSize = g->MeasureString (a2sValue[ixCell, ixRow],
dgvmr->Cells[ixCell]->InheritedStyle->Font,
oLayoutArea,
oStringFormat,
iCharacters,
iLines);
float fHeight = oTextSize.Height;
if (!bAutoHeight)
fHeight += 4;
afRowHeight[ixRow] = Math::Max (afRowHeight[ixRow], fHeight);
a2iLines[ixCell, ixRow] = iLines;
aiLines[ixRow] = Math::Max (aiLines[ixRow], iLines);
}
}
int iLength = dgv->Columns->GetColumnsWidth(DataGridViewElementStates::Visible);
int iHeight = (int)Math::Ceiling(CMath::Sum (afRowHeight));
dgvmr->Height = iHeight;
e->PaintCellsBackground (e->ClipBounds, true);
int iPositionX = e->RowBounds.X + dgvmr->HeaderCell->Size.Width - dgv->HorizontalScrollingOffset;
int iPositionY = 0;
for (int ixCell = 0; ixCell < dgvmr->Cells->Count; ixCell++)
{
String^ sText = nullptr;
DataGridViewCell^ oCell = dgvmr->Cells[ixCell];
Color oTextColor = oCell->Selected ? oCell->InheritedStyle->SelectionForeColor : oCell->InheritedStyle->ForeColor;
Drawing::Brush^ oBrush = gcnew Drawing::SolidBrush (oTextColor);
iPositionY = e->RowBounds.Y;
if (!bAutoHeight)
iPositionY += 2;
for (int ixRow = 0; ixRow < dgvmr->RowCount; ixRow++)
{
if (ixRow > 0)
sText += CONSTS::CRLF;
sText += a2sValue[ixCell, ixRow];
sText += CString::Repeat (CONSTS::CRLF, aiLines[ixRow] - a2iLines[ixCell, ixRow]);
Rectangle oRectText (iPositionX, iPositionY, oCell->Size.Width, oCell->Size.Height);
g->DrawString (a2sValue[ixCell, ixRow], oCell->InheritedStyle->Font, oBrush, oRectText, oStringFormat);
iPositionY += (int)afRowHeight[ixRow];
}
dgvmr->Cells[ixCell]->Value = sText;
iPositionX += oCell->Size.Width;
}
Color oLineColor = dgvmr->Selected ? dgvmr->InheritedStyle->SelectionForeColor : dgvmr->InheritedStyle->ForeColor;
Pen^ oPen = gcnew Pen(oLineColor , 1);
oPen->DashPattern = gcnew array<float>{5, 15};
iPositionX = e->RowBounds.X + dgvmr->HeaderCell->Size.Width - dgv->HorizontalScrollingOffset;
iPositionY = e->RowBounds.Y;
for (int ixRow = 0; ixRow < dgvmr->RowCount - 1; ixRow++)
{
iPositionY += (int)afRowHeight[ixRow];
g->DrawLine (oPen, iPositionX, iPositionY,
iPositionX + iLength, iPositionY);
}
e->PaintHeader (true);
e->Handled = true;
}
//----------------------------------------------------------------------------
// CommonConstructor
//----------------------------------------------------------------------------
void CDataGridViewMultiRow::CommonConstructor ( bool i_bHideRows,
::DataGridView^ i_dgv)
{
m_bHideRows = i_bHideRows;
if (i_dgv)
this->CreateCells(i_dgv);
m_listdgvr = gcnew List<DataGridViewRow^>;
this->ReadOnly = true;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.