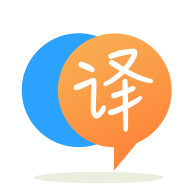
[英]Delete a specific selected row in a table when deleting from a pop-up modal button using angular
[英]Angular2: Delete selected table row on clicking button in modal window
我試圖在表格的任何行上單擊“刪除”按鈕時實現模態,其中它彈出一個模態窗口,要求確認。
當我只執行不帶模式的刪除操作時,它似乎工作正常並且僅刪除該特定行,但是在使用模式時,它僅刪除第一行,而不刪除所選行。
user.component.html
<h1>{{welcome}}</h1>
<table class="table table-bordered">
<tr>
<th>#</th>
<th>Game</th>
<th>Platform</th>
<th>Release</th>
<th>Actions</th>
</tr>
<tr *ngFor="let game of games | paginate: {itemsPerPage: 5, currentPage:page, id: '1'}; let i = index">
<td>{{i + 1}}</td>
<td>{{game.game}}</td>
<td>{{game.platform}}</td>
<td>{{game.release}}</td>
<td><button data-title="title" data-id="2" data-toggle="modal" data-target="#deleteModal" class="confirm-delete"> Delete</button>
</tr>
</table>
<pagination-controls (pageChange)="page = $event" id="1" maxSize="5" directionLinks="true" autoHide="true">
</pagination-controls>
<div class="modal fade" id="deleteModal" tabindex="-1" role="dialog" aria-labelledby="edit" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true"><span class="glyphicon glyphicon-remove" aria-hidden="true"></span></button>
<h4 class="modal-title custom_align" id="Heading">Delete this entry</h4>
</div>
<div class="modal-body">
<div class="alert alert-danger">Are you sure you want to delete this Record?</div>
</div>
<div class="modal-footer ">
<button type="button" class="btn btn-success danger" (click)="deleteGame(i)"><span class="glyphicon glyphicon-ok-sign"></span> Yes</button>
<button type="button" class="btn btn-default" data-dismiss="modal"><span class="glyphicon glyphicon-remove"></span> No</button>
</div>
</div>
<!-- /.modal-content -->
</div>
<!-- /.modal-dialog -->
user.component.ts
import { Component } from '@angular/core';
@Component({
moduleId: module.id,
selector: 'user',
templateUrl: 'user.component.html',
})
export class UserComponent {
welcome : string;
games : [{
game: string,
platform : string,
release : string
}];
constructor(){
this.welcome = "Display List using ngFor in Angular 2";
this.games = [{
game : "Deus Ex: Mankind Divided",
platform: " Xbox One, PS4, PC",
release : "August 23"
},
{
game : "Hue",
platform: " Xbox One, PS4, Vita, PC",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
},
{
game : "Deus Ex: Mankind Divided",
platform: " Xbox One, PS4, PC",
release : "August 23"
},
{
game : "Hue",
platform: " Xbox One, PS4, Vita, PC",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
},
{
game : "Deus Ex: Mankind Divided",
platform: " Xbox One, PS4, PC",
release : "August 23"
},
{
game : "Hue",
platform: " Xbox One, PS4, Vita, PC",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
},
{
game : "Deus Ex: Mankind Divided",
platform: " Xbox One, PS4, PC",
release : "August 23"
},
{
game : "Hue",
platform: " Xbox One, PS4, Vita, PC",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
},
{
game : "Deus Ex: Mankind Divided",
platform: " Xbox One, PS4, PC",
release : "August 23"
},
{
game : "Hue",
platform: " Xbox One, PS4, Vita, PC",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
},
{
game : "The Huntsman: Winter's Curse",
platform: "PS4",
release : "August 23"
}];
};
deleteGame(i){
this.games.splice(i,1);
}
};
您正在遍歷表中的游戲數組,並在每次迭代中給出一個i。 我在ngFor中是已知的,但在循環之外卻不是。 在模式中,您調用deleteGame(i),並且在循環外不知道i。
您可以使用變量“ selectedGame”,並在單擊的行中引用該屬性,如果按“取消”,則將其設置為null。 在deleteGame方法中,您現在可以從數組中刪除對象,因為現在有對選定游戲對象的引用。
也許更好的方法是為模態制作一個單獨的組件,並通過模態組件中的Input()傳遞游戲對象,並使用eventEmitter將對象發回到父組件中,您可以在父組件中調用deleteGame方法。發射器與游戲對象一起發射。
它可能看起來像:
<game-delete-modal [selectedGame]="selectedGame" (deleteGame)="deleteGame($event)"></game-delete-modal>
在模態組件中使用輸入
@Input() selectedGame;
並輸出
@Output() deleteGame = new EventEmitter<Game>();
模態中的delete方法發出deleteGame。
onDeleteGame() {
this.deleteGame.emit(this.selectedGame);
};
希望對您有幫助。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.