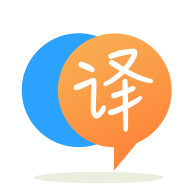
[英]Angular 2 Perform Login Authentication (i am getting into infinite loop.)
[英]How do I perform infinite animations in Angular 2?
基本上,我想使用 angular 中的 web-animations-api polyfill(當前為 4)來對元素執行無限動畫。
讓我們看一個基本的非角度示例:
var ball = document.getElementById('ball'); ball.animate([ { transform: 'scale(0.5)' }, { transform: 'scale(1)' } ], { duration: 1000, iterations: Infinity, direction: 'alternate', easing: 'ease-in-out' });
.container { position: relative; width: 100%; height: 200px; } .ball { position: absolute; width: 80px; height: 80px; border-radius: 50%; border: 2px solid #E57373; background: #F06292; box-shadow: 0px 0px 15px #F06292; top: 0; left: 0; bottom: 0; right: 0; margin: auto; }
<script src="https://cdnjs.cloudflare.com/ajax/libs/web-animations/2.2.5/web-animations.min.js"></script> <div class="container"> <div class="ball" id="ball"><div> </div>
我如何將其翻譯成 Angular?
這是我到目前為止嘗試過但只工作一次:
animations: [
trigger('scale', [
transition('* <=> *', animate('1s ease-in-out', keyframes([
style({ transform: 'scale(0.5)' }),
style({ transform: 'scale(1)' })
]))) // How do I specify the iterations, or the direction?
])
]
有沒有辦法用@angular/animation 插件來做到這一點,而不是存儲一個 ElementRef 並像上面的例子那樣做? 或者我誤解了這個插件的用途?
提前致謝。
我正在為我的項目尋找無限動畫。 angular.io
沒有這方面的angular.io
。 所以我嘗試了這種方式並花費了我很多時間來實現這一目標。 希望它能幫助別人。 首先在class
定義animation
。
animations: [
trigger('move', [
state('in', style({transform: 'translateX(0)'})),
state('out', style({transform: 'translateX(100%)'})),
transition('in => out', animate('5s linear')),
transition('out => in', animate('5s linear'))
]),
]
然后將其插入到您的html
<div [@move]="state" (@move.done)="onEnd($event)"></div>
然后設置state = 'in'
並在ngAfterViewInit()
生命周期鈎子中,使用setTimeout
函數更新您的state
屬性值'out'
,並在結束回調函數后,將您的state
屬性值更新為'in'
並檢查您的state
屬性值,如果in
然后像這樣通過setTimeout
函數更新為out
export class AppComponent implements AfterViewInit {
state = 'in';
ngAfterViewInit() {
setTimeout(() => {
this.state = 'out';
}, 0);
}
onEnd(event) {
this.state = 'in';
if (event.toState === 'in') {
setTimeout(() => {
this.state = 'out';
}, 0);
}
}
}
快樂編碼:)
可以在 Angular 中實現無限動畫,這方面的內容不多,但這對我來說非常適合 onenter 和 onleave 動畫。
當“mouseenter”動畫循環播放時,它會在“mouseleave”處停止。
我正在使用 Angular 6。
Angular CLI:6.0.8 節點:8.9.3 操作系統:linux x64 Angular:6.1.4 ... 動畫、通用、編譯器、編譯器 cli、核心、表單 ... http、語言服務、平台瀏覽器 .. . 平台瀏覽器動態,路由器
rxjs 6.2.2 打字稿 2.7.2 網絡包 4.8.3
import {
trigger,
state,
style,
animate,
transition
} from '@angular/animations';
@Component({
selector: 'hot-spot',
templateUrl: './myComp.component.html',
styleUrls: ['./myComp.component.css'],
animations: [
trigger('compState', [
state('small', style({
opacity: '0.5',
transform: 'scale(0.8)'
})),
state('large', style({
opacity: '1',
transform: 'scale(1.2)'
})),
transition('small => large', animate('0.6s 100ms ease-in')),
transition('large => small', animate('0.7s 100ms ease-out'))
]),
]
})
export class MyComponent {
state: string = 'none';
isEnter: boolean = false;
onMouseEnter(evt: MouseEvent) {
console.log('onMouseEnter()');
this.state = 'small';
this.isEnter = true;
}
onMouseLeave(evt: MouseEvent) {
console.log('onMouseLeave()');
this.state = 'large';
this.isEnter = false;
}
onEnd(event) {
if (this.isEnter) {
if (event.toState === 'small') {
this.state = 'large';
}
else {
this.state = 'small';
}
}
}
}
和 HTML
<div
(mouseenter)="onMouseEnter($event)"
(mouseleave)="onMouseLeave($event)">
<div>
<div
[@compState]="state"
(@compState.done)="onEnd($event)">
<button
(click)="onSceneClick($event,data.sceneId)"
type="button"
class="btn btn-link" >
<i class="fa fa-angle-double-up" style="font-size:16pt;color:red">
<br><span style="font-size:12pt">{{data.text}}</span>
</i>
</button>
</div>
</div>
您可以嘗試使用WebComponents以聲明方式使用Web Animations API (通過使用組件對任何 html 元素進行動畫處理):
<!-- Add Web Animations Polyfill :) --> <script src="https://cdnjs.cloudflare.com/ajax/libs/web-animations/2.3.2/web-animations.min.js"></script> <script type="module" src="https://unpkg.com/@proyecto26/animatable-component@1.0.0/dist/animatable-component/animatable-component.esm.js"></script> <script nomodule="" src="https://unpkg.com/@proyecto26/animatable-component@1.0.0/dist/animatable-component/animatable-component.js"></script> <animatable-component autoplay easing="ease-in-out" duration="800" delay="300" animation="zoomIn" iterations="Infinity" direction="alternate" style="display: flex;align-self: center;" > <h1>Hello World</h1> </animatable-component>
有很多定義的動畫,但您也可以使用關鍵幀創建自定義動畫。
在此處檢查以將其與Angular 、 React 、 VueJS等一起使用: https : //github.com/proyecto26/animatable-component#framework-integrations
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.