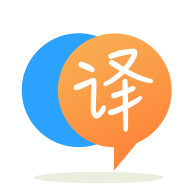
[英]How do I make a border-box get bigger when I hover over something on top of it?
[英]How can I add alert box that says “game over” when the ball touches the top border?
我正在用JavaScript創建一個2人網球類型的游戲,我得到了一個警告框彈出窗口,當它擊中頂部時說“gameover”,但我似乎無法讓它在頂部邊界上工作。 如果有人能提前告訴我我做錯了什么。
我在下面輸入了我的游戲代碼:
var canvas = document.getElementById("gameCanvas"); var ctx = canvas.getContext("2d"); var x = canvas.width / 2; var y = canvas.height - 30; var dx = 2; var dy = -2; var ballRadius = 10; var paddleHeight = 10; var paddleWidth = 75; var paddle2Height = 10; var paddle2Width = 75; var paddleX = (canvas.width - paddleWidth) / 2; var paddle2X = (canvas.width - paddleWidth) / 2; var rightPressed = false; var leftPressed = false; var rightPressed2 = false; var leftPressed2 = false; function drawBall() { ctx.beginPath(); ctx.arc(x, y, ballRadius, 0, Math.PI * 2); ctx.fillStyle = "#0095DD"; //color of ball ctx.fill(); ctx.closePath(); } //Draws the bottom paddle function drawPaddle1() { ctx.beginPath(); ctx.rect(paddleX, canvas.height - paddleHeight, paddleWidth, paddleHeight); ctx.fillStyle = "#0095DD"; //color of paddle ctx.fill(); ctx.closePath(); } //Draws the top paddle function drawPaddle2() { ctx.beginPath(); ctx.rect(paddle2X, 0, paddle2Width, paddle2Height); ctx.fillStyle = "#0095DD"; //color of paddle ctx.fill(); ctx.closePath(); } function draw() { //Checks collison for left and right if (x + dx > canvas.width || x + dx < 0) { dx = -dx; } //Paddle collison for top and down "still fixing" if (y + dy < ballRadius || y + dy < 0) { dy = -dy; } else if (y + dy > canvas.height - ballRadius) { if (x > paddleX && x < paddleX + paddleWidth) { dy = -dy; } else { alert("GAME OVER"); document.location.reload(); } } //Clears canvas so the ball won't leave a trail ctx.clearRect(0, 0, canvas.width, canvas.height); drawBall(); //Draws the ball //Draws the paddle drawPaddle1(); drawPaddle2(); //Makes the ball move x += dx; y += dy; //Moves the paddle if (rightPressed && paddleX < canvas.width - paddleWidth) { paddleX += 7; } else if (leftPressed && paddleX > 0) { paddleX -= 7; } else if (rightPressed2 && paddle2X < canvas.width - paddleWidth) { paddle2X += 7; } else if (leftPressed2 && paddle2X > 0) { paddle2X -= 7; } } //Handles the keyboard commands document.addEventListener("keydown", keyDownHandler, false); document.addEventListener("keyup", keyUpHandler, false); function keyDownHandler(e) { if (e.keyCode == 39) { rightPressed = true; } else if (e.keyCode == 68) { rightPressed2 = true; } else if (e.keyCode == 37) { leftPressed = true; } else if (e.keyCode == 65) { leftPressed2 = true; } } function keyUpHandler(e) { if (e.keyCode == 39) { rightPressed = false; } else if (e.keyCode == 68) { rightPressed2 = false; } else if (e.keyCode == 37) { leftPressed = false; } else if (e.keyCode == 65) { leftPressed2 = false; } } //Refreshes screen every 10 seconds setInterval(draw, 10);
* { padding: 0; margin: 0; } canvas { background: #eee; display: block; margin: 0 auto; }
<canvas id="gameCanvas" width="480" height="320"></canvas>
你錯過了paddle2的一個塊。
//Paddle collison for top and down "still fixing"
if (y + dy < ballRadius || y + dy < 0) {
if (x > paddle2X && x < paddle2X + paddleWidth) {
dy = -dy;
} else {
alert("GAME OVER");
document.location.reload();
}
} else if (y + dy > canvas.height - ballRadius) {
if (x > paddleX && x < paddleX + paddleWidth) {
dy = -dy;
} else {
alert("GAME OVER");
document.location.reload();
}
}
你的游戲很酷。 我只是想完成它。 的jsfiddle
var canvas = document.getElementById("gameCanvas"); var ctx = canvas.getContext("2d"); var x = canvas.width / 2; var y = canvas.height - 30; var dx = 2; var dy = -2; var ballRadius = 10; var paddleHeight = 10; var paddleWidth = 75; var paddle2Height = 10; var paddle2Width = 75; var paddleX = (canvas.width - paddleWidth) / 2; var paddle2X = (canvas.width - paddleWidth) / 2; var rightPressed = false; var leftPressed = false; var rightPressed2 = false; var leftPressed2 = false; var isStart = false; var interval; function drawBall() { ctx.beginPath(); ctx.arc(x, y, ballRadius, 0, Math.PI * 2); ctx.fillStyle = "#0095DD"; //color of ball ctx.fill(); ctx.closePath(); } //Draws the bottom paddle function drawPaddle1() { ctx.beginPath(); ctx.rect(paddleX, canvas.height - paddleHeight, paddleWidth, paddleHeight); ctx.fillStyle = "#0095DD"; //color of paddle ctx.fill(); ctx.closePath(); } //Draws the top paddle function drawPaddle2() { ctx.beginPath(); ctx.rect(paddle2X, 0, paddle2Width, paddle2Height); ctx.fillStyle = "#0095DD"; //color of paddle ctx.fill(); ctx.closePath(); } function draw() { //Checks collison for left and right if (x + dx > canvas.width || x + dx < 0) { dx = -dx; } //Paddle collison for top and down "still fixing" if (y + dy < ballRadius) { console.log("touch border top - Game over"); start(); } if (y + dy >= ballRadius && y + dy <= ballRadius + paddle2Height && x > paddle2X && x < paddle2X + paddleWidth) { console.log("touch paddle top"); dy = -dy; } if (y + dy > canvas.height - ballRadius) { if (x > paddleX && x < paddleX + paddleWidth) { console.log("touch paddle bot"); dy = -dy; } else { console.log("touch border bot - Game over"); start(); } } //Clears canvas so the ball won't leave a trail ctx.clearRect(0, 0, canvas.width, canvas.height); drawBall(); //Draws the ball //Draws the paddle drawPaddle1(); drawPaddle2(); //Makes the ball move x += dx; y += dy; //Moves the paddle if (rightPressed && paddleX < canvas.width - paddleWidth) { paddleX += 7; }; if (leftPressed && paddleX > 0) { paddleX -= 7; }; if (rightPressed2 && paddle2X < canvas.width - paddleWidth) { paddle2X += 7; }; if (leftPressed2 && paddle2X > 0) { paddle2X -= 7; } } //Handles the keyboard commands document.addEventListener("keydown", keyDownHandler, false); document.addEventListener("keyup", keyUpHandler, false); document.getElementById("start-button").addEventListener("click", start); function keyDownHandler(e) { if (e.keyCode == 39) { rightPressed = true; }; if (e.keyCode == 68) { rightPressed2 = true; }; if (e.keyCode == 37) { leftPressed = true; }; if (e.keyCode == 65) { leftPressed2 = true; } } function keyUpHandler(e) { if (e.keyCode == 39) { rightPressed = false; }; if (e.keyCode == 68) { rightPressed2 = false; }; if (e.keyCode == 37) { leftPressed = false; }; if (e.keyCode == 65) { leftPressed2 = false; } } function start() { isStart = !isStart; if (isStart) { x = canvas.width / 2; y = canvas.height - 30; paddleX = (canvas.width - paddleWidth) / 2; paddle2X = (canvas.width - paddleWidth) / 2; //Refreshes screen every 10 seconds interval = setInterval(draw, 10); } else clearInterval(interval); }
* { padding: 0; margin: 0; } canvas { background: #eee; display: block; margin: 0 auto; } button { display: block; margin: 10px auto; padding: 10px; }
<canvas id="gameCanvas" width="480" height="320"></canvas> <button id="start-button">Start/Pause</button>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.