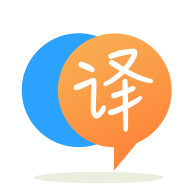
[英]printf() within a C-function outputs wrong result in Python when using ctypes
[英]Wrapping c-function in Python with ctypes
我有一個帶有以下簽名的C函數
__declspec( dllexport ) void* setup(int c_force, int c_stepping, int c_iteration, int c_roots,
struct para* c_userdata, double* c_y0,
double c_reltol, double c_abstol)
{
....
return "a pointer";
}
其中para定義如下。 我想用此函數(和其他函數)構建一個dll並從Python訪問它。 重要的Python行是
import ctypes as ct
lib = ct.cdll.LoadLibrary('lib_path.dll')
getattr(lib, 'setup')
lib.setup.restype = ct.c_void_p
# pystruct as defined below to avoid clogging code
lib.setup.argtypes = [ct.c_int, ct.c_int, ct.c_int, ct.c_int,
ct.POINTER(para), ct.POINTER(ct.c_double),
ct.c_double, ct.c_double]
ptr = lib.setup(cf, cs, ci, cr, ct.byref(para), c_y0, c_reltol, c_abstol)
myobj = ct.c_void_p(ptr)
其中cf
, cs
, ci
, cr
是(python)ints, para
是如下定義的struct-type, c_y0=(ct_c_double * 2)()
(從python端和c端都應為長度2, )和c_reltol
和c_abstol
為ct.c_double,因為c_reltol = ct.c_double(reltol)
。
當我嘗試運行我的主應用程序時,出現WindowsError: exception: access violation writing xxx
在lib.setup
調用中WindowsError: exception: access violation writing xxx
,我看不到原因...在調用lib.setup
之前進行打印lib.setup
給出以下輸出值和類型傳遞給函數的參數(順序)
1 2 2 1 <cparam 'P' (0000000003C84EB0)> <cvode_library.c_double_Array_2 object at 0x0000000003F95BC8> c_double(1e-06) c_double(1e-08)
<type 'int'> <type 'int'> <type 'int'> <type 'int'> <type 'CArgObject'> <class 'cvode_library.c_double_Array_2'> <class 'ctypes.c_double'> <class 'ctypes.c_double'>
我一直在試圖與調試這個和這個問題,但沒有成功。 由於C函數的調用簽名非常簡單,所以我看不到它為什么會中斷。
PS它可以在Ubuntu上完美運行,用__declspec...
替換為extern
c結構定義為
typedef struct para PARA;
struct para
{
double a;
double b;
double c;
};
和相應的pystruct為
class para(ct.Structure):
_fields_ = [('a', ct.c_double),
('b', ct.c_double),
('c', ct.c_double)]
編輯 c_y0
定義為
y0 = np.array([0., 0.])
c_y0 = (ct.c_double * 2)()
c_y0[0] = y0[0]
c_y0[1] = y0[1]
所有“ cvode”函數和N_Vector
都是Sundials套件的一部分, 用於求解非線性方程
__declspec( dllexport ) void* setup(int c_force, int c_stepping, int c_iteration, int c_roots,
struct para* c_userdata, double* c_y0,
double c_reltol, double c_abstol)
{
int flag;
N_Vector y;
void* cvode_mem;
PARA* ptr_para;
ptr_para = c_userdata;
// ****** Set up vector with initial conditions ******
y = N_VNew_Serial(2);
NV_Ith_S(y,0) = c_y0[0];
NV_Ith_S(y,1) = c_y0[1];
// ****** Create cvode object with stepping and iteration method ******
if(c_iteration==CV_FUNCTIONAL)
cvode_mem = CVodeCreate(c_stepping, 1); // Functional iteration
else
cvode_mem = CVodeCreate(c_stepping, 2); // Newton interation
if(check_flag((void *)cvode_mem, "CVodeCreate", 0)) return(NULL);
flag = CVodeInit(cvode_mem, ode, 0, y);
if(check_flag(&flag, "CVodeInit", 1)) return(NULL);
// ****** Specify integration tolerances ******
flag = CVodeSStolerances(cvode_mem, c_reltol, c_abstol);
if(check_flag(&flag, "CVodeSStolerances", 1)) return(NULL);
// ****** Set up linear solver module if required ******
if(c_iteration==CV_DENSE_USER)
{
printf("Dense user supplied Jacobian\n");
// Dense user-supplied Jacobian
flag = CVDense(cvode_mem, 2);
if(check_flag(&flag, "CVDense", 1)) return(NULL);
flag = CVDlsSetDenseJacFn(cvode_mem, jac);
if(check_flag(&flag, "CVDlsSetDenseJacFn", 1)) return(NULL);
}
else if(c_iteration==CV_DENSE_DQ)
{
// Dense difference quotient Jacobian
flag = CVDlsSetDenseJacFn(cvode_mem, NULL);
if(check_flag(&flag, "CVDlsSetDenseJacFn", 1)) return(NULL);
}
// Set optional inputs
flag = CVodeSetUserData(cvode_mem, c_userdata);
if(check_flag(&flag, "CVodeSetUserData", 1)) return(NULL);
// Attach linear solver module
// Specify rootfinding problem
if(c_roots!=ROOTS_OFF)
{
flag = CVodeRootInit(cvode_mem, 1, root_func);
}
return cvode_mem;
}
這是MCVE。 它表明您的聲明是正確的,因此問題可能出在函數實現中。 如果以下方法對您不起作用,請使用類似的MCVE更新您的問題,以重現您的失敗。
測試
#include <stdio.h>
typedef struct para PARA;
struct para
{
double a;
double b;
double c;
};
__declspec(dllexport) void* setup(int c_force, int c_stepping, int c_iteration, int c_roots,
struct para* c_userdata, double* c_y0,
double c_reltol, double c_abstol)
{
printf("%d %d %d %d %lf %lf %lf %lf %lf %lf %lf\n",c_force,c_stepping,c_iteration,c_roots,c_userdata->a,c_userdata->b,c_userda
ta->c,c_y0[0],c_y0[1],c_reltol,c_abstol);
return NULL;
}
test.py
import ctypes as ct
class para(ct.Structure):
_fields_ = [('a', ct.c_double),
('b', ct.c_double),
('c', ct.c_double)]
lib = ct.CDLL('test')
lib.setup.restype = ct.c_void_p
# pystruct as defined below to avoid clogging code
lib.setup.argtypes = [ct.c_int, ct.c_int, ct.c_int, ct.c_int,
ct.POINTER(para), ct.POINTER(ct.c_double),
ct.c_double, ct.c_double]
p = para(1.5,2.5,3.5)
c_y0 = (ct.c_double * 2)(4.5,5.5)
ptr = lib.setup(1,2,3,4,p, c_y0,6.5,7.5)
myobj = ct.c_void_p(ptr)
輸出量
1 2 3 4 1.500000 2.500000 3.500000 4.500000 5.500000 6.500000 7.500000
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.