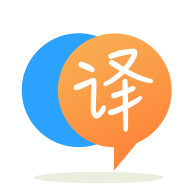
[英]Get selected checkboxes values from table and pass those values into ajax
[英]Table with Checkboxes - selected all Checkboxes and pass values to AJAX script
我在第一列中有一個帶有復選框的表-選中后,它將執行AJAX腳本,該腳本將使用所選值更新PHP會話變量。 一切正常,但我現在需要擴展此功能,以便在第一列的頂部具有一個復選框,以允許用戶選擇表中的所有項目,並將所選項目的值(例如,逗號分隔)作為AJAX腳本的參數-假設我為此需要一個新腳本。
這是我到目前為止的內容:
$(document).ready(function() { $("input.select-item").click(function() { var productID = $(this).val(); // Create a reference to $(this) here: $this = $(this); $.post('productSelections.php', { type: 'updateSelections', productID: productID, selectionType: 'single' }, function(data) { data = JSON.parse(data); if (data.error) { var ajaxError = (data.text); var errorAlert = 'There was an error updating the Product Selections'; $this.closest('td').addClass("has-error"); $("#updateSelectionsErrorMessage").html(errorAlert); $("#updateSelectionsError").show(); return; // stop executing this function any further } else { $this.closest('td').addClass("success") $this.closest('td').removeClass("danger"); } }).fail(function(xhr) { var httpStatus = (xhr.status); var ajaxError = 'There was an error updating the Product Selections'; $this.closest('td').addClass("danger"); $("#updateSelectionsErrorMessage").html(ajaxError); $("#updateSelectionsError").show(); }); }); });
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <table class="table table-condensed table-striped table-bordered"> <thead> <th><input type="checkbox" class="select-all checkbox" name="select-all" /></th> <th class="text-center" scope="col">Product ID</th> <th class="text-center" scope="col">Description</th> </thead> <tbody> <tr class="" id="85799"> <td id="AT36288"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36288" /></td> <td>AT36288</td> <td>Apples</td> <td></td> </tr> <tr class="" id="85800"> <td id="AT36289"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36289" /></td> <td>AT36289</td> <td>Bananas</td> <td></td> </tr> <tr class="" id="85801"> <td id="AT36290"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36290" /></td> <td>AT36290</td> <td>Oranges</td> <td></td> </tr> <tr class="" id="85803"> <td id="AT36292"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36292" /></td> <td>AT36292</td> <td>Grapes</td> <td></td> </tr> </tbody> </table>
我在第一行中添加了一個復選框,該復選框可用於選擇所有項目-本質上等同於單擊每個復選框。 不確定如何擴展或創建一個新腳本,當單擊該腳本時會選中每個復選框,並將ID值傳遞給我可以包含在AJAX腳本中的變量?
$('.select-all').on('click', function(){
var values = []; // will contain all checkbox values that you can send via ajax
$('table > tbody input[type="checkbox"]').each(function(i, el) {
$(el).prop('checked', true);
values.push(el.value);
});
});
在下面的代碼中添加了此功能:
$("input.select-all").click(function() {
$("input.select-item").each(function() {
$(this).trigger('click');
});
});
$(document).ready(function() { $("input.select-all").click(function() { $("input.select-item").each(function() { $(this).trigger('click'); }); }); $("input.select-item").click(function() { var productID = $(this).val(); // Create a reference to $(this) here: $this = $(this); $.post('productSelections.php', { type: 'updateSelections', productID: productID, selectionType: 'single' }, function(data) { data = JSON.parse(data); if (data.error) { var ajaxError = (data.text); var errorAlert = 'There was an error updating the Product Selections'; $this.closest('td').addClass("has-error"); $("#updateSelectionsErrorMessage").html(errorAlert); $("#updateSelectionsError").show(); return; // stop executing this function any further } else { $this.closest('td').addClass("success") $this.closest('td').removeClass("danger"); } }).fail(function(xhr) { var httpStatus = (xhr.status); var ajaxError = 'There was an error updating the Product Selections'; $this.closest('td').addClass("danger"); $("#updateSelectionsErrorMessage").html(ajaxError); $("#updateSelectionsError").show(); }); }); });
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <table class="table table-condensed table-striped table-bordered"> <thead> <th><input type="checkbox" class="select-all checkbox" name="select-all" /></th> <th class="text-center" scope="col">Product ID</th> <th class="text-center" scope="col">Description</th> </thead> <tbody> <tr class="" id="85799"> <td id="AT36288"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36288" /></td> <td>AT36288</td> <td>Apples</td> <td></td> </tr> <tr class="" id="85800"> <td id="AT36289"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36289" /></td> <td>AT36289</td> <td>Bananas</td> <td></td> </tr> <tr class="" id="85801"> <td id="AT36290"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36290" /></td> <td>AT36290</td> <td>Oranges</td> <td></td> </tr> <tr class="" id="85803"> <td id="AT36292"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36292" /></td> <td>AT36292</td> <td>Grapes</td> <td></td> </tr> </tbody> </table>
這與選擇所有復選框的多個table
一起使用效果很好。
特色:
打電話給你的AJAX從callUpdate
// This will work with multiple table items // on page $(document).ready(function(){ function callUpdate( ids, isChecked ){ alert( 'Check status is: ' + isChecked + '\\nids:\\n' + ids.join(', ') ); } var allSelectAllCheckboxes = $('thead th:first .select-all'); // On related checkbox clicked individually allSelectAllCheckboxes.closest('table').find('tbody .select-item').click(function(){ var $el = $(this); callUpdate( [ $el.val() ], $el.prop('checked') ); }); // Look for master checkbox within table thead allSelectAllCheckboxes.click(function(){ // Get clicked checkbox var $clickedCheckbox = $(this); isSelectAllChecked = $clickedCheckbox.prop('checked'), $targetCheckboxes = $clickedCheckbox.closest('table').find('[name=select-item]'), ids = []; // Enumerate through each target checkbx $targetCheckboxes.each( function(){ // Set checkbox check/uncheck // according to 'select all' status this.checked = isSelectAllChecked; // Push product id to collection ids.push( this.value ); }); // Call update using our proxy function callUpdate( ids, isSelectAllChecked ); }); });
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <table class="table table-condensed table-striped table-bordered"> <thead> <th><input type="checkbox" class="select-all checkbox" name="select-all" /></th> <th class="text-center" scope="col">Product ID</th> <th class="text-center" scope="col">Description</th> </thead> <tbody> <tr class="" id="85799"> <td id="AT36288"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36288" /></td> <td>AT36288</td> <td>Apples</td> <td></td> </tr> <tr class="" id="85800"> <td id="AT36289"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36289" /></td> <td>AT36289</td> <td>Bananas</td> <td></td> </tr> <tr class="" id="85801"> <td id="AT36290"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36290" /></td> <td>AT36290</td> <td>Oranges</td> <td></td> </tr> <tr class="" id="85803"> <td id="AT36292"><input type="checkbox" class="select-item checkbox" name="select-item" value="AT36292" /></td> <td>AT36292</td> <td>Grapes</td> <td></td> </tr> </tbody> </table>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.