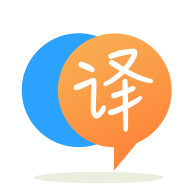
[英]ASP.NET Core MVC model validation in viewmodel with base model
[英]Skip all the validation of a model base on one of its property (ASP.Net Core 2.0)
我如何可以跳過基於其屬性之一的模型的所有驗證。 例如,如果對象的WillBeDeleted
屬性為 true,驗證器將跳過所有驗證規則。
namespace ViewModel
{
public class ContactForm : IViewModel
{
[ValidateNever]
public Contact Item { get; set; }
public Category Category { get; set; }
[Required, DisplayName("First name")]
[StringLength(200, ErrorMessage = "First name should not exceed 200 characters.")]
public string FirstName { get; set; }
[Required, DisplayName("Last name")]
[StringLength(200, ErrorMessage = "Last name should not exceed 200 characters.")]
public string LastName { get; set; }
public List<TelephonesSubForm> Telephones { get; set; } = new List<TelephonesSubForm>();
public List<EmailsSubForm> Emails { get; set; } = new List<EmailsSubForm>();
public class TelephonesSubForm : IViewModel
{
public int Id { get; set; }
public bool MustBeDeleted { get; set; }
[Required]
public TelephoneAndEmailType Type { get; set; }
[Required]
[StringLength(200, MinimumLength = 10, ErrorMessage = "Telephone should not exceed 200 characters.")]
public string Telephone { get; set; }
}
public class EmailsSubForm
{
public int Id { get; set; }
public bool MustBeDeleted { get; set; }
[Required]
public TelephoneAndEmailType Type { get; set; }
[Required]
[StringLength(200, MinimumLength = 10, ErrorMessage = "Email should not exceed 200 characters.")]
public string Email { get; set; }
}
}
}
在給定的示例模型中,當MustBeDeleted
為true
,表示將在保存操作中刪除電子郵件或電話項。
我搜索並發現了幾個關於條件(自定義)驗證的問題,他們建議在檢查ModelState
的驗證狀態之前從ModelState
刪除特定的鍵。 像這個。
我正在添加一個新答案並保留舊答案,因為它可能對某人有用
因此,創建一個自定義屬性:
public class CustomShouldValidateAttribute : Attribute, IPropertyValidationFilter
{
public CustomShouldValidateAttribute(){
}
public bool ShouldValidateEntry(ValidationEntry entry, ValidationEntry parentEntry)
{
//Here you change this verification to Wether it should verificate or not, In this case we are checking if the property is ReadOnly (Only a get method)
return !entry.Metadata.IsReadOnly;
}
}
和他們在你的班級上你用你的自定義屬性裝飾它
[CustomShouldValidateAttribute]
public class MyClass{
public string Stuff{get;set;}
public string Name {get{return "Furlano";}}
}
盡管這不是問題的完整答案,但可以繼承驗證屬性( RequiredAttribute
、 StringLength
等)並覆蓋IsValid
方法。 作為示例,我提供其中之一。
public class RequiredWhenItIsNotDeletingAttribute : RequiredAttribute
{
string DeletingProperty;
/// <summary>
/// Check if the object is going to be deleted skip the validation.
/// </summary>
/// <param name="deletingProperty">The boolean property which shows the object will be deleted.</param>
public RequiredWhenItIsNotDeletingAttribute(string deletingProperty = "MustBeDeleted") =>
DeletingProperty = deletingProperty;
protected override ValidationResult IsValid(object value, ValidationContext validationContext)
{
var property = validationContext.ObjectType.GetProperty(DeletingProperty);
if((bool)property.GetValue(validationContext.ObjectInstance))
return ValidationResult.Success;
return base.IsValid(value, validationContext);
}
}
從 IValidatableObject 繼承您的模型
並實現接口方法:
public virtual IEnumerable<ValidationResult> Validate(ValidationContext validationContext)
{
if (YourCondition==false){
yield return new ValidationResult(
$"This is wrong",
new[] { nameof(Email) });
}
else
yield return ValidationResult.Success
}
但是您必須為您的每個屬性實現驗證並排除您已經編寫的裝飾!
您可以調用該方法:
ModelState.Clear()
當 MustBeDeleted 為真時
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.