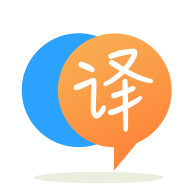
[英]Simple Calculator with all buttons created and actions added in JButton array
[英]How to add two actions to a JButton? Tip calculator program
我正在嘗試創建一個簡單的小費計算器程序,但是,對於一般的動作偵聽器來說,我是新手,所以我不確定自己做錯了什么。 我希望按鈕更新num的值,將其格式化為字符串,然后更改標簽的文本(顯示用戶輸入的內容(即帳單金額))。
這是我的代碼:
package calculatorCW;
import java.awt.Color;
import java.awt.event.*;
import java.text.DecimalFormat;
import java.text.ParseException;
import javax.swing.*;
public class calGui {
double tip = 0;
String num = "000.00", output1 = "00.00", output2 = "00.00";
JFrame frame = new JFrame();
JPanel myPanel = new JPanel();
JLabel label1, label2, label3, label4;
JTextField myText1, myText2;
JButton buttons, tBtn1, tBtn2, tBtn3, calc, btn0;
DecimalFormat formatter = new DecimalFormat();
private int i = 1;
public calGui() {
initializeLabels();
initializeTextFields();
initializeButtons();
setPanels();
setBounds();
guiExtras();
setActionListeners();
}
public static void main(String[] args) {
new calGui();
}
public void initializeLabels() {
label1 = new JLabel("<html> <p style='font-size: 15px; color: red;'> TIP CALCULATOR </p></html>");
label2 = new JLabel("<html> <p style='color: white;'> Bill Amount:</p></html> ");
label3 = new JLabel("<html> <p style='color: white;'>Tip Percentage: </p></html>");
label4 = new JLabel("<html> <p style='color: white;'>Total Cost: </p></html>");
}
public void initializeTextFields() {
myText1 = new JTextField(output1);
myText2 = new JTextField(output2);
}
public void initializeButtons() {
tBtn1 = new JButton("<html> <p style='color: blue;'>15 %</p></html>");
tBtn2 = new JButton("<html> <p style='color: blue;'>18 %</p></html>");
tBtn3 = new JButton("<html> <p style='color: blue;'>20 %</p></html>");
calc = new JButton("<html> <p style='color: black;'>CALC</p></html>");
btn0 = new JButton("0");
//Buttons Loop
for (int col = 50; col <= 190; col = col + 70) {
for (int row = 253; row <= 393; row = row + 70) {
buttons = new JButton("" + i);
myPanel.add(buttons);
buttons.setBounds(row, col, 60, 50);
buttons.setActionCommand(String.valueOf(i));
i++;
}
}
}
public void setPanels() {
myPanel.add(tBtn1);
myPanel.add(tBtn2);
myPanel.add(tBtn3);
myPanel.add(calc);
myPanel.add(btn0);
myPanel.add(label1);
myPanel.add(label2);
myPanel.add(label3);
myPanel.add(label4);
myPanel.add(myText1);
myPanel.add(myText2);
}
public void setBounds() {
tBtn1.setBounds(23, 90, 60, 50);
tBtn2.setBounds(93, 90, 60, 50);
tBtn3.setBounds(163, 90, 60, 50);
calc.setBounds(13, 190, 100, 50);
btn0.setBounds(323, 260, 60, 50);
label1.setBounds(155, -5, 200, 50);
label2.setBounds(13, 50, 100, 20);
label3.setBounds(13, 70, 200, 20);
label4.setBounds(13, 160, 100, 20);
myText1.setBounds(96, 50, 100, 20);
myText2.setBounds(96, 160, 100, 20);
}
public void guiExtras() {
myPanel.setLayout(null);
myPanel.setSize(500, 400);
frame.add(myPanel);
frame.setBounds(600, 50, 500, 400);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
myText1.setEditable(false);
myText2.setEditable(false);
//myPanel.setOpaque(true);
myPanel.setBackground(Color.black);
}
public void setActionListeners() {
//bill amounts
buttons.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
num = num + (e.getActionCommand());
}
});
buttons.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
output1 = formatter.format(formatter.parse(num));
myText1.setText(output1);
} catch (ParseException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
});
//tips
tBtn1.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
tip = .15;
}
});
tBtn2.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
tip = .18;
}
});
tBtn3.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
tip = .20;
}
});
}
}
當前,您將ActionListener添加到“按鈕”中,這只是對在“ initializeButtons()”中創建的按鈕的臨時引用。 隨后調用“ setActionListeners”,然后將ActionListener添加到初始化按鈕時創建的最后一個按鈕。
我建議不要對按鈕使用類變量,而應使用局部變量按鈕。
您可以通過在初始化按鈕時添加ActionListener來解決此問題:
public void initializeButtons() {
...
//Buttons Loop
for (int col = 50; col <= 190; col = col + 70) {
for (int row = 253; row <= 393; row = row + 70) {
JButton button = new JButton("" + i);
// bill amounts
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
num = num + (e.getActionCommand());
try {
output1 = formatter.format(formatter.parse(num));
myText1.setText(output1);
} catch (ParseException e1) {
e1.printStackTrace();
}
}
});
...
}
}
}
你可以在同一個監聽器上做
button.addActionListener(new Actionlistener{
public void actionPerformed(ActionEvent ae){
//add code to update value of num
//change text of Jlabel
}
});
我的新問題是:我的代碼現在有兩個問題。 第一個問題是,當我按任意數字按鈕添加帳單金額時,它不會在帳單金額文本中顯示。 如果用戶輸入9。我希望它顯示“ 000.09”。 如果現在用戶輸入5,我希望帳單金額現在顯示為“ 000.95”。 我了解我的數學運算方式是否錯誤(我在Button動作偵聽器中進行設置的方式)。 我遇到的第二個問題是我添加了一個重置按鈕,並希望它將textFields中的值重置為原始值。 在setResetListener()方法中,我為reset按鈕創建了一個動作偵聽器,以便將double的值轉換回其原始值。 我希望它再顯示在textField上。 但是,它不會這樣做。 我還希望它可以將myPanel的背景重新着色為原始的黑色。我猜這是由於線程引起的嗎? 這是我到目前為止的代碼。
package calculatorCW;
import java.awt.Color;
import java.awt.event.*;
import java.text.DecimalFormat;
import java.text.ParseException;
import javax.swing.*;
public class calGui
{
private int i=1;
double tip=0,finalAmount=0,amountTaxed=0;
String num="000.00",output1="000.00",output2="000.00";
JFrame frame = new JFrame();
JPanel myPanel = new JPanel();
JLabel label1, label2, label3, label4;
JTextField myText1, myText2;
JButton buttons,tBtn1,tBtn2, tBtn3,calc,btn0,reset;
DecimalFormat formatter = new DecimalFormat();
public calGui()
{
initializeLabels();
initializeTextFields();
initializeButtons();
setPanels();
setBounds();
guiExtras();
setTipListeners();
setCalcListener();
}
public void initializeLabels()
{
label1 = new JLabel("<html> <p style='font-size: 15px; color: red;'> TIP CALCULATOR </p></html>");
label2 = new JLabel("<html> <p style='color: white;'> Bill Amount:</p></html> ");
label3 = new JLabel("<html> <p style='color: white;'>Tip Percentage: </p></html>");
label4 = new JLabel("<html> <p style='color: white;'>Total Cost: </p></html>");
}
public void initializeTextFields()
{
myText1 = new JTextField(output1);
myText2 = new JTextField(output2);
}
public void initializeButtons()
{
tBtn1 = new JButton("<html> <p style='color: blue;'>15 %</p></html>");
tBtn2 = new JButton("<html> <p style='color: blue;'>18 %</p></html>");
tBtn3 = new JButton("<html> <p style='color: blue;'>20 %</p></html>");
calc = new JButton("<html> <p style='color: black;'>CALC</p></html>");
reset = new JButton("<html> <p style='color: black;'>Reset</p></html>");
btn0 = new JButton("0");
//Buttons Loop
for (int col = 50; col<=190; col=col+70)
{
for(int row =253; row<=393; row=row+70)
{
buttons = new JButton(""+i);
myPanel.add(buttons);
buttons.setBounds(row, col, 60, 50);
buttons.setActionCommand(String.valueOf(i));
setButtonsListeners();
i++;
}
}
}
public void setPanels()
{
myPanel.add(tBtn1);
myPanel.add(tBtn2);
myPanel.add(tBtn3);
myPanel.add(calc);
myPanel.add(reset);
myPanel.add(btn0);
myPanel.add(label1);
myPanel.add(label2);
myPanel.add(label3);
myPanel.add(label4);
myPanel.add(myText1);
myPanel.add(myText2);
}
public void setBounds()
{
tBtn1.setBounds(23, 90, 60, 50);
tBtn2.setBounds(93, 90, 60, 50);
tBtn3.setBounds(163, 90, 60, 50);
calc.setBounds(13, 190, 100, 50);
reset.setBounds(13, 260, 100, 50);
btn0.setBounds(323, 260, 60, 50);
label1.setBounds(155,-5,200, 50);
label2.setBounds(13, 50, 100, 20);
label3.setBounds(13, 70, 200, 20);
label4.setBounds(13, 160, 100, 20);
myText1.setBounds(96, 50, 100, 20);
myText2.setBounds(96, 160, 100, 20);
}
public void guiExtras()
{
myPanel.setLayout(null);
myPanel.setSize(500,400);
frame.add(myPanel);
frame.setBounds(600,50,500,400);
frame.setVisible(true);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
myText1.setEditable(false);
myText2.setEditable(false);
//myPanel.setOpaque(true);
myPanel.setBackground(Color.black);
}
public void setButtonsListeners()
{
//bill amounts
buttons.addActionListener(new ActionListener()
{@Override public void actionPerformed(ActionEvent e)
{
num=num+(e.getActionCommand());
//buttons.setEnabled(true);
try {
output1=formatter.format(formatter.parse(num));
} catch (ParseException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
myText1.setText(output1);
}});
}
public void setTipListeners()
{
//tips
tBtn1.addActionListener(new ActionListener() {@Override public void actionPerformed(ActionEvent e) {tip=.15;}});
tBtn2.addActionListener(new ActionListener() {@Override public void actionPerformed(ActionEvent e) {tip=.18;}});
tBtn3.addActionListener(new ActionListener() {@Override public void actionPerformed(ActionEvent e) {tip=.20;}});
}
public void setCalcListener()
{
calc.addActionListener(new ActionListener() {@Override public void actionPerformed(ActionEvent e)
{
amountTaxed=(Double.parseDouble(output1)*tip)+Double.parseDouble(output1);
finalAmount=(amountTaxed*8.25)+amountTaxed;
try {
output2=formatter.format(formatter.parse(Double.toString(finalAmount)));
} catch (ParseException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
myText2.setText(output2);
myPanel.setBackground(Color.gray);
label1.setText(("<html> <p style='font-size: 15px; color: white;'> TIP CALCULATOR </p></html>"));
}});
}
public void setResetListerner()
{
reset.addActionListener(new ActionListener() {@Override public void actionPerformed(ActionEvent e)
{
tip=0;
finalAmount=0;
amountTaxed=0;
num="000.00";
output1="000.00";
output2="000.00";
myText2.setText(output2);
myText1.setText(output1);
myPanel.setBackground(Color.BLACK);
//myPanel.repaint();
}});
}
public static void main(String[] args)
{
new calGui();
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.