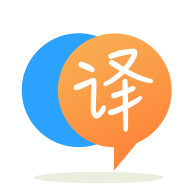
[英]How can I get the resolution of an embeded image in a PDF using ITextSharp
[英]How can I change background image color in PDF using itextsharp
我正在使用 itextsharp 來創建 pdf 並且它工作得很好,但是現在當我嘗試添加背景圖像(作為水印)時,我想將圖像顏色更改為黑白,但不知道該怎么做。 我正在發布屏幕截圖以及用於添加背景圖像的代碼。
代碼 :
public class PdfWriterEvents : IPdfPageEvent
{
string watermarkText = string.Empty;
public PdfWriterEvents(string watermark)
{
watermarkText = watermark;
}
public void OnStartPage(PdfWriter writer, Document document)
{
float width = document.PageSize.Width;
float height = document.PageSize.Height;
try
{
PdfContentByte under = writer.DirectContentUnder;
Image image = Image.GetInstance(watermarkText);
image.ScaleToFit(275f, 275f);
image.SetAbsolutePosition(150, 300);
under.AddImage(image);
}
catch (Exception ex)
{
Console.Error.WriteLine(ex.Message);
}
}
public void OnEndPage(PdfWriter writer, Document document) { }
public void OnParagraph(PdfWriter writer, Document document, float paragraphPosition) { }
public void OnParagraphEnd(PdfWriter writer, Document document, float paragraphPosition) { }
public void OnChapter(PdfWriter writer, Document document, float paragraphPosition, Paragraph title) { }
public void OnChapterEnd(PdfWriter writer, Document document, float paragraphPosition) { }
public void OnSection(PdfWriter writer, Document document, float paragraphPosition, int depth, Paragraph title) { }
public void OnSectionEnd(PdfWriter writer, Document document, float paragraphPosition) { }
public void OnGenericTag(PdfWriter writer, Document document, Rectangle rect, String text) { }
public void OnOpenDocument(PdfWriter writer, Document document) { }
public void OnCloseDocument(PdfWriter writer, Document document) { }
}
對於調用這個:
writer.PageEvent = new PdfWriterEvents(LogoImage);
那么如何像普通水印圖像一樣將顏色更改為黑白。
謝謝 !
您可以通過兩種方式更改圖像顏色:
顯然是最簡單的一種:使用像 MS Paint 或 Adobe Photoshop 這樣的圖像編輯器來更改圖像內容顏色。
在運行時,正如您在評論中所述:“我想知道是否還有其他選項可以通過后端代碼使用 itextsharp 更改圖像的顏色”。 您可以嘗試以下代碼,而不是使用itextsharp
:
static void Main(string[] args)
{
try
{
Bitmap bmp = null;
//The Source Directory in debug\bin\Big\
string[] files = Directory.GetFiles("Big\\");
foreach (string filename in files)
{
bmp = (Bitmap)Image.FromFile(filename);
bmp = ChangeColor(bmp);
string[] spliter = filename.Split('\\');
//Destination Directory debug\bin\BigGreen\
bmp.Save("BigGreen\\" + spliter[1]);
}
}
catch (System.Exception ex)
{
Console.WriteLine(ex.ToString());
}
}
public static Bitmap ChangeColor(Bitmap scrBitmap)
{
//You can change your new color here. Red,Green,LawnGreen any..
Color newColor = Color.Red;
Color actualColor;
//make an empty bitmap the same size as scrBitmap
Bitmap newBitmap = new Bitmap(scrBitmap.Width, scrBitmap.Height);
for (int i = 0; i < scrBitmap.Width; i++)
{
for (int j = 0; j < scrBitmap.Height; j++)
{
//get the pixel from the scrBitmap image
actualColor = scrBitmap.GetPixel(i, j);
// > 150 because.. Images edges can be of low pixel color. if we set all pixel color to new then there will be no smoothness left.
if (actualColor.A > 150)
newBitmap.SetPixel(i, j, newColor);
else
newBitmap.SetPixel(i, j, actualColor);
}
}
return newBitmap;
}
積分: DareDevil 的回答
您可以使用提供的方法編輯圖像。
PS:當您完成並對結果感到滿意時,請為@DareDevil 的回答點贊,這是一個絕妙的發現!
首先,您使用OnStartPage
創建背景。 這實際上是錯誤的做法。 iText 開發者一再強調,在OnStartPage
不得向文檔中添加任何內容。 相反,應該使用OnEndPage
,在您的情況下它不會強加任何問題,因此您真的應該這樣做。
如果您只有一個位圖,最好的方法當然是在某些圖像處理軟件中打開該位圖,然后更改顏色以使圖像最佳作為水印背景。
另一方面,如果您有許多可能的圖像用作背景,可能您甚至為每個新文檔都獲得了一個單獨的圖像,那么您就無法再手動將每個圖像調整到最佳狀態。 相反,您可以通過某些服務操作位圖本身,也可以使用 PDF 特定功能來操作圖像外觀。
例如,使用您的頁面事件偵聽器,我得到了以下信息:
使用混合模式色相用白色覆蓋背景變成:
這看起來很暗,但我們可以在混合模式Screen中用淺灰色將其照亮,覆蓋背景:
為此,我將代碼從您的PdfWriterEvents
方法OnStartPage
移至OnEndPage
(請參閱我的答案的開頭)並將其更改如下:
public void OnEndPage(PdfWriter writer, Document document)
{
float width = document.PageSize.Width;
float height = document.PageSize.Height;
try
{
iTextSharp.text.Image image = iTextSharp.text.Image.GetInstance(watermarkText);
image.ScaleToFit(275f, 275f);
image.SetAbsolutePosition(150, 300);
PdfGState gStateHue = new PdfGState();
gStateHue.BlendMode = new PdfName("Hue");
PdfGState gStateScreen = new PdfGState();
gStateScreen.BlendMode = new PdfName("Screen");
PdfContentByte under = writer.DirectContentUnder;
under.SaveState();
under.SetColorFill(BaseColor.WHITE);
under.Rectangle(document.PageSize.Left, document.PageSize.Bottom, document.PageSize.Width, document.PageSize.Height);
under.Fill();
under.AddImage(image);
under.SetGState(gStateHue);
under.SetColorFill(BaseColor.WHITE);
under.Rectangle(document.PageSize.Left, document.PageSize.Bottom, document.PageSize.Width, document.PageSize.Height);
under.Fill();
under.SetGState(gStateScreen);
under.SetColorFill(BaseColor.LIGHT_GRAY);
under.Rectangle(document.PageSize.Left, document.PageSize.Bottom, document.PageSize.Width, document.PageSize.Height);
under.Fill();
under.RestoreState();
}
catch (Exception ex)
{
Console.Error.WriteLine(ex.Message);
}
}
我從IconArchive復制了圖像。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.