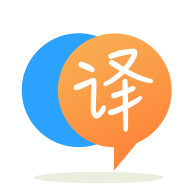
[英]undefined reference to 'std::string Helper::ToString<int>(int const&)'
[英]Undefined reference to 'PreconditionViolatedException::PreconditionViolatedException(std::string const&)'
我在尋找一個包含四個文件的代碼項目中遇到了很多麻煩:
GiftRegistry.cpp:
#include "GiftRegistry.h"
#include "LinkList/LinkedList.h"
#include "LinkList/LinkedList.cpp"
#include <iostream>
template<class ItemType>
GiftRegistry<ItemType>::GiftRegistry() : giftRegistry(new LinkedList<ItemType>())
{
}
template<class ItemType>
bool GiftRegistry<ItemType>::addGift(const ItemType& gift, int desiredRank)
{
if(giftRegistry->insert(desiredRank, gift))
{
return true;
}
else{
return false;
}
}
template<class ItemType>
void GiftRegistry<ItemType>::moveGift(int curPos, int newPos)
{
if(giftRegistry->isEmpty())
{
ItemType itemToMove = giftRegistry->getEntry(curPos);
if(giftRegistry->insert(newPos, itemToMove))
{
cout << "Gift moved successfully!";
}
if(giftRegistry->remove(curPos))
{
cout << "Gift removed successfully";
}
else{
cout << "Operation failed. Please examine input.";
}
}
}
template<class ItemType>
bool GiftRegistry<ItemType>::removeGift(int position)
{
if(giftRegistry->isEmpty())
{
if(giftRegistry->remove(position))
{
return true;
}
}
else
{
return false;
}
}
template<class ItemType>
void GiftRegistry<ItemType>::showRegistry()
{
for(int i = 1; i < giftRegistry->getLength(); i++)
{
ItemType itemToPrint = giftRegistry->getEntry(i);
std::cout << i + "." + itemToPrint;
}
}
main.cpp:
#include "GiftRegistry.cpp"
//#include "LinkList/LinkedList.cpp"
#include <iostream>
#include <string>
using namespace std;
int main()
{
ListInterface<string>* listPtr = new LinkedList<string>();
//RegistryInterface<string>* weddingRegistry = new GiftRegistry<string>();
string gift = "hello there";
listPtr->insert(1, gift);
cout << listPtr->getEntry(1);
// string gift = "Box of rocks";
//
// RegistryInterface<string>* weddingRegistry = new GiftRegistry<string>();
// if(weddingRegistry->addGift(gift, 1))
// {
// cout << "Added item " + gift;
// weddingRegistry->showRegistry();
// }
// else
// {
// cout << "Failed to add item. Error.";
// }
//weddingRegistry->showRegistry();
return 0;
}
GiftRegistry.h:
#ifndef Gift_Registry_
#define Gift_Registry_
#include "RegistryInterface.h"
#include "C:\Users\Austin\Documents\LinkList\LinkedList.h"
using namespace std;
template<class ItemType>
class GiftRegistry : public RegistryInterface<ItemType>
{
private:
LinkedList<ItemType>* giftRegistry;
public:
string CustomerName;
string EventName;
GiftRegistry();
bool addGift(const ItemType& gift, int desiredRank);
void moveGift(int curPos, int newPos);
bool removeGift(int position);
void showRegistry();
virtual ~GiftRegistry();
};
#endif // _GiftRegistry_
RegistryInterface.h:
#ifndef REGISTRY_INTERFACE_
#define REGISTRY_INTERFACE_
template<class ItemType>
class RegistryInterface
{
public:
/** Adds gift to the gift registry
@post Add was successful
@param gift The gift to be added to the registry.
@param giftRank The rank to be attributed to the gift. Also determines the point of insertion for the gift.
@return 'True' if addition was successful, 'false' if item already exists in the list
**/
virtual bool addGift(const ItemType& gift, int giftRank) = 0;
/** Moves the gift to the user-desired location / priority
@pre List is not empty
@param currentPosition The current position of the gift to be moved.
@param desiredPosition The new position
**/
virtual void moveGift(int currentPosition, int desiredPosition) = 0;
/** Removes the specified item from the List
@pre List is not empty
@post Item was removed
@param position The index of the item to be removed
@return 'True' if gift was removed, 'False' if not
**/
virtual bool removeGift(int position) = 0;
/** Prints the registry contents and displays them by most wanted to least wanted
@pre List is not empty
**/
virtual void showRegistry() = 0;
/** Destroys this registry and frees its assigned memory.
**/
virtual ~RegistryInterface() { }
};
#endif // REGISTRY_INTERFACE_
我正在使用Code :: Blocks 16.01並遵循C ++ 11標准。 運行“ main.cpp”文件時出現問題,導致出現以下錯誤:
|| ===構建:在GiftList中調試(編譯器:GNU GCC編譯器)=== | obj \\ Debug \\ main.o ||在函數ZNK10LinkedListISsE8getEntryEi':| C:\\Users\\Arralan\\Documents\\LinkList\\LinkedList.cpp|121|undefined reference to
ZNK10LinkedListISsE8getEntryEi':| C:\\Users\\Arralan\\Documents\\LinkList\\LinkedList.cpp|121|undefined reference to
PreconditionViolatedException :: PreconditionViolatedException(std :: string const&)'|的ZNK10LinkedListISsE8getEntryEi':| C:\\Users\\Arralan\\Documents\\LinkList\\LinkedList.cpp|121|undefined reference to
obj \\ Debug \\ main.o ||在函數ZN10LinkedListISsE7replaceEiRKSs':| C:\\Users\\Arralan\\Documents\\LinkList\\LinkedList.cpp|140|undefined reference to
ZN10LinkedListISsE7replaceEiRKSs':| C:\\Users\\Arralan\\Documents\\LinkList\\LinkedList.cpp|140|undefined reference to
PreconditionViolatedException :: PreconditionViolatedException(std :: string const&)'|的ZN10LinkedListISsE7replaceEiRKSs':| C:\\Users\\Arralan\\Documents\\LinkList\\LinkedList.cpp|140|undefined reference to
||錯誤:ld返回1個退出狀態| || ===構建失敗:3個錯誤,0個警告(0分鍾,0秒)=== |
我對數據結構不熟悉,這個問題使我掛斷了兩天。 任何幫助或指導將不勝感激,但請記住,這是一項家庭作業。
“ LinkList”中的代碼沒有錯誤,並且可以在其單獨的項目中按預期運行,但是當被另一個項目引用時,其行為會異常。 我認為問題可能出在我的“ #include”語句上。
編輯:問題是由在此唯一實例中必需的'#include'引起的。 我找到的解決方案,無論是否最佳,都是通過'#include'添加此路徑:“ LinkList / PreconditionViolatedException.cpp”。 將此行添加到我的“ main.cpp”后,錯誤已不再出現。
該問題是由此唯一實例中必需的'#include'引起的。 我找到的解決方案,無論是否最佳,都是通過'#include'添加此路徑:“ LinkList / PreconditionViolatedException.cpp”。 將此行添加到我的“ main.cpp”后,錯誤已不再出現。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.