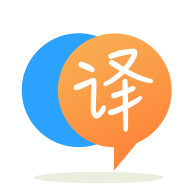
[英]Java: is there more elegant way to extract a new list from an existing list using reduce?
[英]Is there a more elegant way to get random not used item from list using java 8?
要重構的功能......
<T> T notUsedRandomItem(List<T> allItems, List<T> usedItems) {
return allItems.stream()
.filter(item -> !usedItems.contains(item))
.sorted((o1, o2) -> new Random().nextInt(2) - 1)
.findFirst()
.orElseThrow(() -> new RuntimeException("Did not find item!"));
}
功能可能會像這樣使用......
System.out.println(
notUsedRandomItem(
Arrays.asList(1, 2, 3, 4),
Arrays.asList(1, 2)
)
); // Should print either 3 or 4
編輯:通過針對人員列表運行它們來收集建議的實現和測試效率。
edit2:為Person類添加了缺少的equals方法。
import java.util.*;
import java.util.concurrent.TimeUnit;
import java.util.function.BiFunction;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import static java.util.stream.Collectors.toList;
class Functions {
<T> T notUsedRandomItemOriginal(List<T> allItems, List<T> usedItems) {
return allItems.stream()
.filter(item -> !usedItems.contains(item))
.sorted((o1, o2) -> new Random().nextInt(2) - 1)
.findFirst()
.orElseThrow(() -> new RuntimeException("Did not find item!"));
}
<T> T notUsedRandomItemByAominè(List<T> allItems, List<T> usedItems) {
List<T> distinctItems = allItems.stream()
.filter(item -> !usedItems.contains(item))
.collect(toList());
if (distinctItems.size() == 0) throw new RuntimeException("Did not find item!");
return distinctItems.get(new Random().nextInt(distinctItems.size()));
}
<T> T notUsedRandomItemByEugene(List<T> allItems, List<T> usedItems) {
// this is only needed because your input List might not support removeAll
List<T> left = new ArrayList<>(allItems);
List<T> right = new ArrayList<>(usedItems);
left.removeAll(right);
return left.get(new Random().nextInt(left.size()));
}
<T> T notUsedRandomItemBySchaffner(List<T> allItems, List<T> usedItems) {
Set<T> used = new HashSet<>(usedItems);
List<T> all = new ArrayList<>(allItems);
Collections.shuffle(all);
for (T item : all) if (!used.contains(item)) return item;
throw new RuntimeException("Did not find item!");
}
}
public class ComparingSpeedOfNotUsedRandomItemFunctions {
public static void main(String[] plaa) {
runFunctionsWith(100);
runFunctionsWith(1000);
runFunctionsWith(10000);
runFunctionsWith(100000);
runFunctionsWith(200000);
}
static void runFunctionsWith(int itemCount) {
TestConfiguration testConfiguration = new TestConfiguration();
Functions functions = new Functions();
System.out.println("Function execution time with " + itemCount + " items...");
System.out.println("Schaffner: " +
testConfiguration.timeSpentForFindingNotUsedPeople(
itemCount, (allPeople, usedPeople) ->
functions.notUsedRandomItemBySchaffner(allPeople, usedPeople)
));
System.out.println("Eugene: " +
testConfiguration.timeSpentForFindingNotUsedPeople(
itemCount, (allPeople, usedPeople) ->
functions.notUsedRandomItemByEugene(allPeople, usedPeople)
));
System.out.println("Aominè: " +
testConfiguration.timeSpentForFindingNotUsedPeople(
itemCount, (allPeople, usedPeople) ->
functions.notUsedRandomItemByAominè(allPeople, usedPeople)
));
System.out.println("Original: " +
testConfiguration.timeSpentForFindingNotUsedPeople(
itemCount, (allPeople, usedPeople) ->
functions.notUsedRandomItemOriginal(allPeople, usedPeople)
));
}
}
class TestConfiguration {
Long timeSpentForFindingNotUsedPeople(int numberOfPeople, BiFunction<List<Person>, List<Person>, Person> function) {
ArrayList<Person> people = new ArrayList<>();
IntStream.range(1, numberOfPeople).forEach(i -> people.add(new Person()));
Collections.shuffle(people);
List<Person> halfOfPeople =
people.stream()
.limit(numberOfPeople / 2)
.collect(Collectors.toList());
Collections.shuffle(halfOfPeople);
long before = System.nanoTime();
Person foundItem = function.apply(people, halfOfPeople);
long after = System.nanoTime();
// Return -1 if function do not return valid answer
if (halfOfPeople.contains(foundItem))
return (long) -1;
return TimeUnit.MILLISECONDS.convert(after - before, TimeUnit.NANOSECONDS);
}
class Person {
public final String name = UUID.randomUUID().toString();
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Person person = (Person) o;
return name != null ? name.equals(person.name) : person.name == null;
}
@Override
public int hashCode() {
return name != null ? name.hashCode() : 0;
}
}
}
結果:
Function execution time with 100 items...
Schaffner: 0
Eugene: 1
Aominè: 2
Original: 5
Function execution time with 1000 items...
Schaffner: 0
Eugene: 14
Aominè: 13
Original: 5
Function execution time with 10000 items...
Schaffner: 2
Eugene: 564
Aominè: 325
Original: 348
Function execution time with 20000 items...
Schaffner: 3
Eugene: 1461
Aominè: 1418
Original: 1433
Function execution time with 30000 items...
Schaffner: 3
Eugene: 4616
Aominè: 2832
Original: 4567
Function execution time with 40000 items...
Schaffner: 4
Eugene: 10889
Aominè: 4903
Original: 10394
結論
當列表大小達到10000個項目時,到目前為止只有Schaffner的實現可用。
而且因為閱讀相當簡單,我會選擇它作為最優雅的解決方案。
我可以想到這一點,但不知道它與現有解決方案相比如何擴展:
<T> T notUsedRandomItem(List<T> allItems, List<T> usedItems) {
// this is only needed because your input List might not support removeAll
List<T> left = new ArrayList<>(allItems);
List<T> right = new ArrayList<>(usedItems);
left.removeAll(right);
return left.get(new Random().nextInt(left.size()));
}
要記住的一件事是, sorted
是一個有狀態操作,因此它會對整個“diff”進行排序,但是你只能從中檢索一個元素。 你的Comparator
也是錯誤的,對於相同的兩個值o1
和o2
你可能會說它們是不同的 - 這可能會以神秘的方式打破。
您應該使用HashSet
來提高性能:
<T> T notUsedRandomItem(List<T> allItems, List<T> usedItems) {
Set<T> used = new HashSet<>(usedItems);
Set<T> all = new HashSet<>(allItems);
all.removeIf(used::contains); // or all.removeAll(used)
if (all.isEmpty()) throw new RuntimeException("Did not find item!");
int skip = new Random().nextInt(all.size());
Iterator<T> it = all.iterator();
for (int i = 0; i < skip; i++) it.next();
return it.next();
}
如果元素屬於used
集合,則會從all
集合中刪除元素。 在使用Set.removeIf
和Set.contains
,元素的刪除是最佳的性能。 然后,在結果集中跳過隨機數量的元素,最后,返回該集合的下一個元素。
另一種方法是首先對all
列表進行混洗,然后簡單地迭代並返回不屬於已used
集合的第一個元素:
<T> T notUsedRandomItem(List<T> allItems, List<T> usedItems) {
Set<T> used = new HashSet<>(usedItems);
List<T> all = new ArrayList<>(allItems);
Collections.shuffle(all);
for (T item : all) if (!used.contains(item)) return item;
throw new RuntimeException("Did not find item!");
}
編輯:檢查最后一段代碼,我現在意識到沒有必要洗牌整個列表。 相反,您可以隨機化allItems
列表的索引並返回不屬於已used
集的第一個元素:
<T> T notUsedRandomItem(List<T> allItems, List<T> usedItems) {
Set<T> used = new HashSet<>(usedItems);
return new Random().ints(allItems.size(), 0, allItems.size())
.mapToObj(allItems::get)
.filter(item -> !used.contains(item))
.findAny()
.orElseThrow(() -> new RuntimeException("Did not find item!"));
}
在Comparator
你傳遞到sorted
中間操作似乎是錯誤的和奇怪的方式使用Comparator
我的眼睛無妨; 這與@Eugene在帖子中提到的內容有關。
因此,我建議你避免任何類型的陷阱,並始終按照預期的方式使用API; 而已。
如果你真的想要一個來自上述列表的隨機元素; 唯一可能的方法是找到兩個列表的所有不同元素。 所以我們無法提高這方面的速度。
一旦完成,我們只需要在包含不同元素的列表范圍內生成一個隨機整數,並在其中包含至少一個元素的情況下為其索引。
雖然我不得不承認,在沒有使用流的情況下,可能有更好的方法來完成手頭的任務; 這里是我如何稍微修改你的代碼以消除.sorted((o1, o2) -> new Random().nextInt(2) - 1)
。
<T> T notUsedRandomItem(List<T> allItems, List<T> usedItems) {
List<T> distinctItems = allItems.stream()
.filter(item -> !usedItems.contains(item))
.collect(toList());
if(distinctItems.size() == 0) throw new RuntimeException("Did not find item!");
return distinctItems.get(new Random().nextInt(distinctItems.size()));
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.