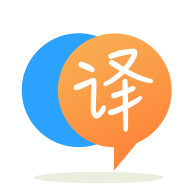
[英]Read in a text file 1 line at a time and split the words into an Array Java
[英]In Java I need to read a text file and put each line in a separate array. But every time I read the text file I cannot split the lines
在Java中,我需要讀取一個文本文件(該文件包含三行,並且需要將每行放在一個雙行進位中)。 但是我不能分界線。 這是我到目前為止的代碼。 但我不知道如何進行:
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
public class FedEx {
public static void main(String[] args) throws Exception
{
//Read the file
FileReader file = new FileReader("info.txt");
BufferedReader br = new BufferedReader(file);
String text = " ";
String line = br.readLine();
while(line != null)
{
text += line;
line = br.readLine();
}
//Print the text file.
System.out.println(text);
}
}
我認為您不希望讀取數字文件並將其放入雙精度數組中,如果那樣,則可以看到以下代碼:
注意:我使用
try-with-resource
來防止源泄漏。
public class FedEx {
public static void main(String[] args) throws Exception {
// Read the file
try (BufferedReader br = new BufferedReader(new FileReader("info.txt"))) {
String line;
List<Double> doubles = new ArrayList<>();
while ((line = br.readLine()) != null) {
doubles.add(Double.parseDouble(line));
}
System.out.println(doubles);
}
}
}
文件樣本:
343
6787
19
22
58
0
輸出樣本:
[343.0, 6787.0, 19.0, 22.0, 58.0, 0.0]
無需一起添加文本,因此,如果您只想逐行顯示文件的內容,請嘗試以下操作:
public class FedEx {
public static void main(String[] args) throws Exception {
// Read the file
try (BufferedReader br = new BufferedReader(new FileReader("info.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
}
}
}
文件樣本:
343
6787
19
22
58
0
輸出樣本:
343
6787
19
22
58
0
使用Java 8功能的方法如下:
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class FileLineByLine {
private static List<Double> file2doubles(String fileName) throws IOException {
try (Stream<String> stream = Files.lines(Paths.get(fileName))) {
return stream.map(Double::parseDouble).collect(Collectors.toList());
}
}
public static void main(String args[]) throws Exception {
List<Double> doubles = file2doubles(args[0]);
System.out.println(doubles);
}
}
您可以通過參數化線轉換使它更通用,請參見此處https://gist.github.com/sorokod/818ccd65cad967cdecb7dbf4006e4fa0
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.