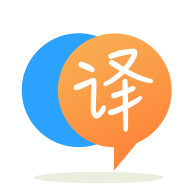
[英]I need to get a file reader to read a text file line by line and format them in Java
[英]I need to take an array of three lines in a text file and sort them base on the first line in Java
我需要在文本文件中使用三行數組,並根據Java中的第一行對其進行排序。 我還需要操縱它,然后打印到屏幕上。
我有一個格式如下的測試文件:
10
Michael
Jackson
12
Richard
Woolsey
我需要從文本文件中輸入此內容,然后根據與名稱關聯的數字重新排列它。 那時,我需要使用隨機數生成器,並根據隨機數為每個名稱分配一個變量。 然后,我需要打印以不同的格式來篩選添加的變量和名稱。 這是輸出示例:
12:
Woolsey, Richard
Variable assigned
10:
Jackson, Michael
Other variable assigned
我非常感謝您的幫助。 我問是因為我真的不知道如何將三行作為一個變量輸入,然后在程序中稍后進行操作。 謝謝,科里
我不確定我是否理解您的問題。 除了排序之外,下面的類似內容可能會有所幫助。 請注意,這是一個不完整的解決方案,其中不存儲任何輸入,並且僅將輸出打印一次(然后丟棄)。
Random generator = new Random();
try { BufferedReader in = new BufferedReader(new FileReader("filename"));
String str;
String firstName = null:
while ((str = in.readLine()) != null) {
// try to create an Integer out of the "str"-variable
Integer nr = null;
try{
nr = Integer.parseInt(str);
firstName = null;
}
catch (NumberFormatException e) {
//line was not a number
if (firstName != null) {
// str is the last name
System.out.println(nr);
System.out.println(str + ", " firstName + " " + generator.nextInt());
}
else {
firstName = str;
}
}
}
in.close();
}
catch (IOException e) { }
請注意,此操作不會進行任何排序。 看一下該部分的比較器。 您也可以使用java.util.Scanner
從命令行讀取輸入(如果它不在文件中)。
Scanner
讀取輸入中的行 Random
生成隨機數 Entry
類以封裝每個條目的數據 Comparator<Entry>
和Collections.sort
List<Entry>
此代碼段應具有指導意義:
import java.util.*;
import java.io.*;
public class Entry implements Comparable<Entry> {
final int id;
final int whatever;
final String firstName;
final String lastName;
Entry(int id , String firstName, String lastName, int whatever) {
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.whatever = whatever;
}
@Override public String toString() {
return String.format(
"%d:%n%s, %s%n(%d)%n",
id, lastName, firstName, whatever);
}
@Override public int compareTo(Entry other) {
return
(this.id < other.id) ? +1 :
(this.id > other.id) ? -1 :
0;
}
}
在這里,我們將每個Entry
封裝在其自己的不可變值類型類中。 它還implements Comparable<Entry>
,以降序比較id
(假定為唯一)。
現在,我們可以使用SortedSet<Entry>
進行其余操作:
public static void main(String[] args) throws FileNotFoundException {
SortedSet<Entry> entries = new TreeSet<Entry>();
Scanner sc = new Scanner(new File("sample.txt"));
Random r = new Random();
while (sc.hasNextInt()) {
int id = sc.nextInt();
sc.nextLine();
String firstName = sc.nextLine();
String lastName = sc.nextLine();
int whatever = r.nextInt();
entries.add(new Entry(id, firstName, lastName, whatever));
}
for (Entry e : entries) {
System.out.println(e);
}
}
如果sample.txt
包含以下內容:
10
Michael
Jackson
12
Richard
Woolsey
那么輸出可能是:
12:
Woolsey, Richard
(-1279824163)
10:
Jackson, Michael
(320574093)
@Override
我看到了您的帖子,並想給您一個快速的答案。
檢查此鏈接: 逐行讀取文件
您也應該閱讀Java教程。
每行之后,您可以使用以下內容將其保存到二維數組中:
String str;
i = 0;
j = 0;
while ((str = in.readLine()) != null) {
array[i][j] = str;
j++;
if (j == 3){
j = 0;
i++;
}
}
in.close();
然后,您可以按自己喜歡的任何方式使用數組值。
祝好運。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.