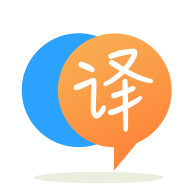
[英]“Incorrect Content-Type: image/png” when trying to upload png with Ajax to Asp.net Core server
[英]InvalidOperationException: Incorrect Content-Type: ASP.NET Core
我有兩種形式,一種用於登錄,一種用於注冊。 這些都在同一視圖上並且使用相同的模型。 我正在使用控制器處理表單提交。
訪問登錄頁面時出現以下錯誤
InvalidOperationException: Incorrect Content-Type:
完整的錯誤日志: https : //pastebin.com/JjfDtr6q
我已經上網瀏覽了,找不到與我的問題直接相關的任何東西,並且在C#/。NET Core中經驗不足,無法理解導致問題的原因。
我的觀點
@model PastPapers.Models.LoginModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Login</title>
</head>
<body>
<!-- Register form inside modal -->
<div id="registerModal" class="">
<div class="">
<header class="">
<span onclick="document.getElementById('registerModal').style.display = 'none'" class="">×</span>
<h2>Register</h2>
</header>
<div class="">
<form asp-controller="Login" asp-action="AttemptRegister" method="post">
<input asp-for="newUsername" type="text" placeholder="Username" />
<input asp-for="newEmail" type="email" placeholder="Email" />
<input asp-for="newPassword" type="password" placeholder="Password" />
<input type="submit" value="Register" />
</form>
</div>
</div>
</div>
<!-- Login form -->
<form asp-controller="Login" asp-action="AttemptLogin" method="post">
<input asp-for="username" type="text" placeholder="Username" />
<input asp-for="password" type="password" placeholder="Password" />
<input type="submit" value="Login" />
</form>
<button onclick="document.getElementById('registerModal').style.display='block'" class="">Register</button>
</body>
</html>
我的控制器
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using PastPapers.Models;
namespace PastPapers.Controllers
{
public class LoginController : Controller
{
public IActionResult Index(LoginModel loginModel = null)
{
if (loginModel == null)
{
return View();
} else
{
return View(loginModel);
}
}
[HttpPost]
public IActionResult AttemptLogin(LoginModel loginModel = null)
{
if (loginModel != null)
{
loginModel.AttemptLogin();
if (loginModel.loginSuccess)
{
return RedirectToAction("Index", "Home", null);
} else
{
return RedirectToAction("Index", loginModel);
}
} else
{
return RedirectToAction("Index");
}
}
[HttpPost]
public IActionResult AttemptRegister(LoginModel loginModel = null)
{
if (loginModel != null)
{
loginModel.AttemptRegister();
if (loginModel.registerSuccess)
{
return RedirectToAction("Index");
} else
{
return RedirectToAction("Index", loginModel);
}
} else
{
return RedirectToAction("Index");
}
}
}
}
我的模特
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using MySql.Data.MySqlClient;
using Newtonsoft.Json;
using PastPapers.Helpers;
using Microsoft.AspNetCore.Http;
namespace PastPapers.Models
{
public class LoginModel : HttpContextAccessor
{
public string username { get; set; }
public string password { get; set; }
public bool loginSuccess { get; set; }
public string loginErrorMessage { get; set; }
public string newUsername { get; set; }
public string newPassword { get; set; }
public string newEmail { get; set; }
public bool registerSuccess { get; set; }
public string registerErrorMessage { get; set; }
[JsonIgnore]
public AppDb Db { get; set; }
public LoginModel(AppDb db = null)
{
Db = db;
loginSuccess = false;
registerSuccess = false;
}
public LoginModel()
{
}
public void AttemptRegister()
{
try
{
Db.Connection.Open();
string sql = "INSERT INTO users (id, username, password, email) VALUES (DEFAULT, @username, @password, @email)";
MySqlCommand cmd = new MySqlCommand(sql, Db.Connection);
cmd.Prepare();
cmd.Parameters.AddWithValue("@username", newUsername);
cmd.Parameters.AddWithValue("@password", SecurePasswordHasher.Hash(newPassword));
cmd.Parameters.AddWithValue("@email", newEmail);
if (cmd.ExecuteNonQuery() == 0)
{
System.Diagnostics.Debug.WriteLine("Account failed to create!");
registerSuccess = false;
} else
{
registerSuccess = true;
}
} catch (Exception ex)
{
registerErrorMessage = "Error connecting to database";
System.Diagnostics.Debug.WriteLine(ex.Message);
}
}
public void AttemptLogin()
{
try
{
Db.Connection.Open();
string sql = "SELECT password FROM users WHERE username=@username";
MySqlCommand cmd = new MySqlCommand(sql, Db.Connection);
cmd.Prepare();
cmd.Parameters.AddWithValue("@username", username);
MySqlDataReader reader = cmd.ExecuteReader();
if (reader.Read())
{
string dbPassword = reader.GetString(0);
if (SecurePasswordHasher.Verify(password, dbPassword))
{
loginSuccess = true;
HttpContext.Session.SetString("username", username);
} else
{
loginSuccess = false;
loginErrorMessage = "Incorrect password";
}
} else
{
loginErrorMessage = "Unknown username";
}
} catch (Exception ex)
{
loginErrorMessage = "Error connecting to database";
System.Diagnostics.Debug.WriteLine(ex.Message);
}
}
}
}
我放棄了HttpContextAccessor(或IHttpContextAccessor)的整個繼承,因為您將失去具體HttpContextAccessor提供的功能。 而是將HttpContextAccessor注入到控制器中,然后使用對Login模型的操作結果來設置會話值。 像這樣:
在您的Startup.ConfigureServices
添加以下行:
services.AddSingleton<IHttpContextAccessor, HttpContextAccessor>();
這樣可以將HttpContext注入到控制器中。
然后在您的控制器中,添加一個接受IHttpContextAccessor的構造函數:
public LoginController(IHttpContextAccessor contextAccessor)
{
// save to a field, like _httpContext = contextAccessor.HttpContext;
}
然后將該HttpContext作為參數傳遞給LoginModel.AttemptLogin
。 這樣,您仍然可以滿足訪問HTTP變量的要求,並且代碼更具可維護性和可預測性。
需要注意的是-通過將HttpContextAccessor添加到服務中,對性能的影響很小。 但總體來說更好。
用IHttpContextAccessor替換HttpContextAccessor的繼承,並添加一個公共HttpContext屬性。 下面的新模型。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using MySql.Data.MySqlClient;
using Newtonsoft.Json;
using PastPapers.Helpers;
using Microsoft.AspNetCore.Http;
namespace PastPapers.Models
{
public class LoginModel : IHttpContextAccessor
{
public string username { get; set; }
public string password { get; set; }
public bool loginSuccess { get; set; }
public string loginErrorMessage { get; set; }
public string newUsername { get; set; }
public string newPassword { get; set; }
public string newEmail { get; set; }
public bool registerSuccess { get; set; }
public string registerErrorMessage { get; set; }
[JsonIgnore]
public AppDb Db { get; set; }
public HttpContext HttpContext { get; set; }
public LoginModel(AppDb db = null)
{
Db = db;
loginSuccess = false;
registerSuccess = false;
}
public LoginModel()
{
}
public void AttemptRegister()
{
try
{
Db.Connection.Open();
string sql = "INSERT INTO users (id, username, password, email) VALUES (DEFAULT, @username, @password, @email)";
MySqlCommand cmd = new MySqlCommand(sql, Db.Connection);
cmd.Prepare();
cmd.Parameters.AddWithValue("@username", newUsername);
cmd.Parameters.AddWithValue("@password", SecurePasswordHasher.Hash(newPassword));
cmd.Parameters.AddWithValue("@email", newEmail);
if (cmd.ExecuteNonQuery() == 0)
{
System.Diagnostics.Debug.WriteLine("Account failed to create!");
registerSuccess = false;
} else
{
registerSuccess = true;
}
} catch (Exception ex)
{
registerErrorMessage = "Error connecting to database";
System.Diagnostics.Debug.WriteLine(ex.Message);
}
}
public void AttemptLogin()
{
try
{
Db.Connection.Open();
string sql = "SELECT password FROM users WHERE username=@username";
MySqlCommand cmd = new MySqlCommand(sql, Db.Connection);
cmd.Prepare();
cmd.Parameters.AddWithValue("@username", username);
MySqlDataReader reader = cmd.ExecuteReader();
if (reader.Read())
{
string dbPassword = reader.GetString(0);
if (SecurePasswordHasher.Verify(password, dbPassword))
{
loginSuccess = true;
HttpContext.Session.SetString("username", username);
} else
{
loginSuccess = false;
loginErrorMessage = "Incorrect password";
}
} else
{
loginErrorMessage = "Unknown username";
}
} catch (Exception ex)
{
loginErrorMessage = "Error connecting to database";
System.Diagnostics.Debug.WriteLine(ex.Message);
}
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.