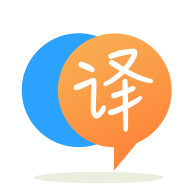
[英]Java: Using 2 or more Classes for Freemarker Data Model processing
[英]Java File Classes Processing Numeric Data
public class AvgScore {
public static void main(String[] args) {
File dataFile = new File("scores.dat");
FileReader in;
BufferedReader readFile;
String score;
double avgScore;
double totalScores = 0;
int numScores = 0;
try {
in = new FileReader(dataFile);
readFile = new BufferedReader(in);
while ((score = readFile.readLine()) != null) {
numScores += 1;
System.out.println(score);
totalScores += Double.parseDouble(score);
}
avgScore = totalScores / numScores;
System.out.println("Average = " + avgScore);
readFile.close();
in.close();
} catch (FileNotFoundException e) {
System.out.println("File does not exist or could not be found");
System.err.println("FileNotFoundException: "
+ e.getMessage());
} catch (IOException e) {
System.out.println("Problem reading file.");
System.err.println("IOException: " + e.getMessage());
}
}
}
當我運行它時,我得到了Tia
Exception in thread "main" java.lang.NumberFormatException: For input
string: "Tia"
at sun.misc.FloatingDecimal.readJavaFormatString(Unknown Source)
at sun.misc.FloatingDecimal.parseDouble(Unknown Source)
at java.lang.Double.parseDouble(Unknown Source)
at AvgScore.main(AvgScore.java:18)
//My desired output I want is
//Tia
//88
//Ben
//55
//Derek
//77
//John
//65
我犯什么錯誤? 該代碼對我來說似乎很牢固。 另外,我該如何編碼程序,以便能夠識別最高和最低分數,因為我想輸出該分數。 任何幫助將不勝感激。
確保分數變量實際上包含數值的字符串表示形式,換句話說,除了小數點的句點外,沒有空格,制表符,空白行(空字符串)或任何字母字符。 由於明顯的原因, Double.parseDouble()方法無法將這些字符轉換為雙精度數據類型,因此會引發您收到的異常。 僅允許使用數值。 您可以使用String.matches()方法和正則表達式進行檢查 :
在您的while循環中:
// If 'score' is NOT a numerical value (contains alpha char's)
if (!score.matches("-?\\d+(\\.\\d+)?") {
// Skip code below and continue 'while' loop.
// If it's not numerical value then it must
// be a name or a blank line
continue;
}
else {
// 'score' DOES contain a numerical value...
numScores++; // same as: numScores += 1; OR numScores = numScores + 1;
System.out.println(score);
totalScores += Double.parseDouble(score);
}
這是String.matches()方法中使用的正則表達式的解釋:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.