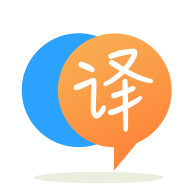
[英]How can I create a file from a byte array and send it to the stream as a file without creating this file on the disk?
[英]How can i fill a byte array with a string without creating a new array?
我正在嘗試打開多個websocket,我需要以某種方式在每個套接字上使用相同的緩沖區,或者在發送/接收新消息之前清除它們。 接收方法很好,因為我可以傳遞字節數組的參數,它將填充該參數而無需創建新的字節數組實例。
我該如何使用BitConverter.GetBytes
方法?我需要開始使用不安全的上下文並使用帶有指針參數的重載GetBytes
嗎?還有其他方法嗎? 我需要它來填充將在構造函數中定義的outBytes
變量。
public class Client:IDisposable
{
//Fields
public char[] innerData { get; private set; }
private byte[] inBytes;
private byte[] outBytes;
private ArraySegment<byte> inSegment;
private ArraySegment<byte> outSegment;
private WebSocket webSocket;
public WebSocket Socket => this.webSocket;
public readonly string clientID;
//Auxiliary
private const int BufferSize = 1024;
public static Client CreateClient(WebSocket socket, string id)
{
Client client = new Client(socket, id);
return client;
}
public Client(WebSocket socket, string id)
{
this.inBytes = new byte[BufferSize];
this.inSegment = new ArraySegment<byte>(inBytes);
this.outBytes = new byte[BufferSize];
this.outSegment = new ArraySegment<byte>(outBytes);
this.webSocket = socket;
this.clientID = id;
this.innerData = new char[BufferSize];
}
public async Task<WebSocketReceiveResult> ReceiveResult()
{
if(this.webSocket.State!=WebSocketState.Open)
{
return null;
}
WebSocketReceiveResult result = await this.webSocket.ReceiveAsync(this.inSegment, CancellationToken.None);
Encoding.UTF8.GetChars(this.inSegment.Array, 0, BufferSize, this.innerData, 0);
return result;
}
public async Task SendMessage(string message)
{
if(this.webSocket.State==WebSocketState.Open)
{
this.outBytes = Encoding.UTF8.GetBytes(message, 0, message.Length); //How can i fill the already existing outBytes?
await this.webSocket.SendAsync(this.outSegment, WebSocketMessageType.Text, true, CancellationToken.None);
}
}
public void Dispose()
{
if(this.webSocket.State!=WebSocketState.Closed)
{
this.webSocket.Dispose();
this.webSocket = null;
}
}
}
我需要以某種方式利用現有outBytes
當我轉換的消息,我將send.At此刻outBytes
的行為就像一個指針,並在每一個方法的SendMessage的每一次迭代GetBytes
將產生一個新的字節數組。
您顯然對GetBytes的工作方式有誤解,它不會每次都生成新的數組,此重載:
Encoding.GetBytes方法(字符串,Int32,Int32,Byte [],Int32)
將
將指定字符串中的一組字符編碼為指定字節數組(來自MSDN)
所以你的線應該是
Encoding.UTF8.GetBytes(message, 0, message.Length, this.outBytes, 0);
該函數將把使用UTF8編碼轉換為字節的字符串填充到數組中,然后您可以使用返回值(它是整數)來檢查已將多少字節寫入到數組中。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.