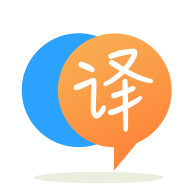
[英]How to calculate the average and max/min of an array of calculations from input?
[英]How to exclude numbers from array sum/average/min/max?
我正在為班級分配作業,我試圖弄清楚如何獲取數組中數字的平均值,同時排除數字-999(用於指示缺少的數據),我想出了如何獲取總和/平均/最低/最高等,但我無法弄清楚如何從搜索范圍中排除-999。 您可以在前幾種方法中看到到目前為止我已經嘗試解決的一些事情。 我什至在Google上都找不到任何東西,甚至可能無法開始解釋我現在應該做什么。 這些是我要遵循的說明,以下是我當前的代碼,感謝您的輸入。
/**
* WeatherComputation.java
*/
//Put any imports below this line.
import java.util.*;
/**
* Static methods library which compute averages and other
* computations on integer arrays of temperatures.
*
* @author Joel Swanson
* @version 03.29.2014
*/
public class WeatherComputation
{
/**
* Average an array of temperatures.
* @param temperatures An array storing temperatures as ints.
* @return Returns the average of the array of ints.
*/
public static double averageTemperature(int[] temperatures)
{
int sum = 0;
for (int i = 0; i < temperatures.length; i++)
{
sum += temperatures[i];
}
double average = sum / temperatures.length;
return average;
}
/**
* Find the highest in an array of temperatures.
* @param temperatures An array storing temperatures as ints.
* @return The largest value from the the array of ints.
*/
public static int highestTemperature(int[] temperatures)
{
int max = temperatures[0];
for (int i = 1; i < temperatures.length; i++)
{
if(temperatures[i] > max)
{
max = temperatures[i];
}
}
return max;
}
/**
* Find the lowest in an array of temperatures.
* @param temperatures An array storing temperatures as ints.
* @return The lowest value from the the array of ints.
*/
public static int lowestTemperature(int[] temperatures)
{
int min = 0;
Arrays.sort(temperatures);
while (true)
{
if (min == -999)
{
break;
}
if(min > -999)
{
min = temperatures[0];
}
}
for (int i = 1; i < temperatures.length; i++)
{
if (min < -999)
{
if (temperatures[i] < min)
{
min = temperatures[i];
}
}
}
return min;
}
/**
* Return the total number of missing days. That is days with
* -999 recorded as the temperatures.
* @param temperatures An array storing temperatures as ints.
* @return The number of -999s found.
*/
public static int numberMissing(int[] temperatures)
{
int count = 0;
return count;
}
/**
* Calculate heating degree day.
* @param max The highest temperature for a given day.
* @param min The lowest temperature for a given day.
* @return heating degree day data for this day.
*/
public static double hdd(int max, int min)
{
double hdd = 0.0;
return hdd;
}
/**
* Calculate cooling degree day.
* @param max The highest temperature for a given day.
* @param min The lowest temperature for a given day.
* @return cooling degree day data for this day.
*/
public static double cdd(int max, int min)
{
double cdd = 0.0;
return cdd;
}
/**
* Sum monthly heating degree days.
* @param max An array with the highest temperatures for each day
* in a given month.
* @param min An array with the lowest temperatures for each day
* in a given month.
* @return The sum of the heating degree days.
*/
public static double monthHdd(int[] max, int[] min)
{
double sum = 0.0;
return sum;
}
/**
* Sum monthly cooling degree days.
* @param max An array with the highest temperatures for each day
* in a given month.
* @param min An array with the lowest temperatures for each day
* in a given month.
* @return The sum of the cooling degree days.
*/
public static double monthCdd(int[] max, int[] min)
{
double sum = 0.0;
return sum;
}
}
您確實想為此使用Java 8的流運算符。 如果我采用您的代碼,而忽略了未實現的方法,並且沒有描述實際計算的內容,那么我會得到:
/**
* WeatherComputation.java
*/
import java.util.*;
import java.util.stream.IntStream;
/**
* Static methods library which compute averages and other
* computations on integer arrays of temperatures.
*
* @author Joel Swanson
* @version 03.29.2014
*/
public class WeatherComputation {
/**
* Filters out all missing values (represented by -999).
*
* @param temperaturs an array storing temperatures as ints.
* @return the list with the missing values removed
*/
private static IntStream getFilteredArray(final int[] temperaturs) {
return IntStream.of(temperaturs)
.filter(i -> i != -999);
}
/**
* Average an array of temperatures.
*
* @param temperatures an array storing temperatures as ints.
* @return the average of the array of ints.
*/
public static double averageTemperature(int[] temperatures) {
// Returns 0 on an empty list. Your code will throw an exception
return getFilteredArray(temperatures).average().orElse(0d);
}
/**
* Find the highest in an array of temperatures.
*
* @param temperatures an array storing temperatures as ints.
* @return the largest value from the the array of ints.
*/
public static int highestTemperature(int[] temperatures) {
return getFilteredArray(temperatures).max().orElse(0);
}
/**
* Find the lowest in an array of temperatures.
*
* @param temperatures an array storing temperatures as ints.
* @return the lowest value from the the array of ints.
*/
public static int lowestTemperature(int[] temperatures) {
return getFilteredArray(temperatures).min().orElse(0);
}
/**
* Return the total number of missing days. That is days with
* -999 recorded as the temperatures.
*
* @param temperatures an array storing temperatures as ints.
* @return the number of -999s found.
*/
public static int numberMissing(int[] temperatures) {
return (int) IntStream.of(temperatures)
.filter(t -> t == -999)
.count();
}
}
方法getFilteredArray
完成所有工作。 它將數組轉換為IntStream
,刪除所有-999
值,然后返回流。 然后,您可以使用流運算符來計算平均值,總和,最小值,最大值等值。
public static double averageTemperature(int[] temperatures)
{
int sum = 0;
int count = 0;// number of valid values
for (int i = 0; i < temperatures.length; i++)
{
if(temperatures[i] > -999){ // before calculating check value
count ++;
sum += temperatures[i];
}
}
double average = count != 0 ? sum / count : -999 - 1; // preventing divide by zero
return average;
}
public static int lowestTemperature(int[] temperatures) {
if(temperatures == null || temperatures.length == 0)
return -999 - 1;
int min = temperatures[0];
for (int temperature : temperatures) {
if (temperature > -999 && temperature < min) {
min = temperature;
}
}
return min;
}
您只需要在每一步中檢查該值是否為排除值,然后再以sum / average / min / max方法使用它即可。
例如:
public static double averageTemperature(int[] temperatures)
{
int sum = 0;
int count = 0;
for (int i = 0; i < temperatures.length; i++)
{
if (temperatures[i] != -999) {
sum += temperatures[i];
count++;
}
}
double average = sum / count;
return average;
}
對於最低溫度,這就是我要做的:
public static int lowestTemperature(int[] temperatures) {
int min = 999;
for (int temperature : temperatures) {
if (temperature > -999 && temperature < min) {
min = temperature;
}
}
return min;
}
假設您的WeatherComputation類也將考慮999
的方式與考慮-999
(無效值)的方式相同。 原因很簡單,如果一個月內只有負值怎么辦? 然后,您的int min = 0
將是錯誤的。
至於平均溫度:
public static double averageTemperature(int[] temperatures) {
int sum = 0, validTemperatureCounter = 0;
for (int temperature : temperatures) {
if (temperature > -999 && temperature < 999) {
validTemperatureCounter++;
sum += temperature;
}
}
return sum / (double)validTemperatureCounter;
}
我也隨意忽略了999
的值。
為了好玩,有一個班輪:
public static double averageTemperatureOneLiner(int[] temperatures) {
return Arrays.stream(temperatures).filter(t -> t > -999 && t < 999).average().orElse(0);
}
public static int lowestTemperatureOneLiner(int[] temperatures) {
return Arrays.stream(temperatures).filter(t -> t > -999 && t < 999).min().orElse(0);
}
public static int highestTemperatureOneLiner(int[] temperatures) {
return Arrays.stream(temperatures).filter(t -> t > -999 && t < 999).max().orElse(0);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.