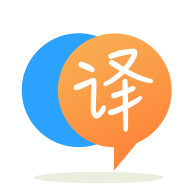
[英]Segmentation fault while iterating a std::set<std::string> in C++
[英]Segmentation fault in C++ with std::cin while macro is defined
我正在嘗試解決與堆棧數據結構有關的問題,我有一個堆棧的實現,以及使用它的主要方法,因為我是初學者,這是一個有學習意義的問題,你們能告訴我,為什么我得到這個錯誤?:
GDB trace:
Reading symbols from solution...done.
[New LWP 24202]
Core was generated by `solution'.
Program terminated with signal SIGSEGV, Segmentation fault.
#0 main () at solution.cc:70
70 cin >> N;
#0 main () at solution.cc:70
我的代碼如下:
#include <cmath>
#include <cstdio>
#include <vector>
#include <iostream>
#include <algorithm>
using namespace std;
#define MAX_SIZE 5000000
class Stack
{
private:
int A[MAX_SIZE]; // array to store the stack
int top; // variable to mark the top index of stack.
public:
// constructor
Stack()
{
top = -1; // for empty array, set top = -1
}
// Push operation to insert an element on top of stack.
void Push(int x)
{
if(top == MAX_SIZE -1) { // overflow case.
printf("Error: stack overflow\n");
return;
}
A[++top] = x;
}
// Pop operation to remove an element from top of stack.
void Pop()
{
if(top == -1) { // If stack is empty, pop should throw error.
printf("Error: No element to pop\n");
return;
}
top--;
}
// Top operation to return element at top of stack.
int Top()
{
return A[top];
}
// This function will return 1 (true) if stack is empty, 0 (false) otherwise
int IsEmpty()
{
if(top == -1) return 1;
return 0;
}
// ONLY FOR TESTING - NOT A VALID OPERATION WITH STACK
// This function is just to test the implementation of stack.
// This will print all the elements in the stack at any stage.
void Print() {
int i;
printf("Stack: ");
for(i = 0;i<=top;i++)
printf("%d ",A[i]);
printf("\n");
}
};
int main() {
int N;
cin >> N;
Stack S1;
Stack S2;
for(int i = 0; i < N; i++)
{
int q;
cin >> q;
if(q == 1)
{
int x;
cin >> x;
if(S1.IsEmpty() || S2.IsEmpty())
{
S1.Push(x);
S2.Push(x);
}
else
{
S1.Push(x);
if(x >= S2.Top()) S2.Push(x);
}
}
if(q==2)
{
if(S1.Top() == S2.Top())
{
S1.Pop();
S2.Pop();
}else
{
S1.Pop();
}
}
if(q==3)
{
cout << S2.Top() << endl;
}
}
return 0;
}
如果我將MAX_SIZE變量設置為較小的數字,則代碼運行良好,我想知道為什么是這種情況,std :: cin與宏如何相互作用?,我是初學者,很抱歉,如果這是一個簡單的問題,那是我第一次在stackoverflow中詢問時,
MAX_SIZE
太大。 MAX_SIZE
確定Stack
對象的大小。 由於函數中局部變量的總大小限制為幾兆字節(取決於平台),因此您只需超出此大小即可。
在您的情況下,您在main
有兩個本地Stack
對象( S1
和S2
),每個對象大約占用20 MB(假設sizeof int
為4)。
不過,這與cin
完全無關。
您的Stack
對象分配在堆棧上。
默認情況下,根據您的平台,每個線程的堆棧限制為1-8 MB。
每個堆棧對象占用20 MB,因此您的堆棧空間不足。 要解決此問題,請將您的代碼更改為:
std::unique_ptr<Stack> S1(new Stack());
std::unique_ptr<Stack> S2(new Stack());
這將在堆上分配對象,該對象僅受計算機上可用內存和交換空間大小的限制。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.