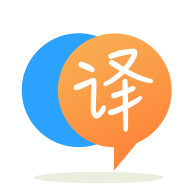
[英]Return the number of times that the string “hello” appears anywhere in the given string
[英]Return the number of times that the string “hi” appears anywhere in the given string
我編寫了以下Java代碼,它返回了一個超時錯誤。 我不確定這意味着什么,也不知道為什么代碼不會運行
public int countHi(String str) {
int pos = str.indexOf("hi");
int count = 0;
while(pos!=-1)
{
count++;
pos = str.substring(pos).indexOf("hi");
}
return count;
}
我知道一個替代解決方案,使用for循環,但我真的認為這也會起作用。
你進入了一個無限循環,因為pos
永遠不會超過第一個匹配,因為第一個匹配將包含在子字符串中。
您可以通過在while
循環中使用此重寫版本的indexOf()
來修復:
pos = str.indexOf("hi", pos + 1);
或者使用do... while
循環以避免重復調用indexOf()
:
public static int countHi(String str) {
int pos = -1, count = -1;
do {
count++;
pos = str.indexOf("hi", pos + 1);
} while (pos != -1);
return count;
}
str.substring(pos)
輸出給定索引的子字符串。 因此在你的代碼中,while循環永遠不會遍歷你的整個字符串,而是在第一個“hi”停止。使用它。
while(pos!=-1){
count++;
str = str.substring(pos+2);
pos = str.indexOf("hi");
}
str變量存儲字符串的后半部分(使用+2表示另外兩個索引用於hi的結尾)然后檢查pos變量存儲,“hi”索引出現在新字符串中。
只是為了增加樂趣......
如果要計算提供的子字符串(即:“hi”)並且它在輸入字符串(單個單詞或單詞的一部分)中的位置無關緊要,則可以使用單個字符串並使字符串。 replace()方法通過從初始輸入字符串中實際刪除想要計數的所需子字符串並計算該輸入字符串的剩余部分(這不會修改初始輸入字符串)來為您完成工作:
String inputString = "Hi there. This is a hit in his pocket";
String subString = "hi";
int count = (inputString.length() - inputString.replace(subString, "").
length()) / subString.length())
//Display the result...
System.out.println(count);
控制台將顯示: 3
您將注意到上面的代碼是字母區分大小寫的,因此在上面的示例中,子字符串“hi”與單詞“Hi”不同,因為大寫的“H”因此忽略“Hi” 。 如果要在計算所提供的子字符串時忽略字母大小寫,則可以使用相同的代碼,但在其中使用String.toLowerCase()方法:
String inputString = "Hi there. This is a hit in his pocket";
String subString = "hi";
int count = (inputString.length() - inputString.toLowerCase().
replace(substring.toLowerCase(), "").
length()) / substring.length())
//Display the result...
System.out.println(count);
控制台將顯示: 4
但是,如果要計算的提供的子字符串是一個特定的單詞(不是另一個單詞的一部分 ),那么它會變得更復雜一些。 一種方法是使用模式和匹配器類以及一個小的正則表達式 。 它可能看起來像這樣:
String inputString = "Hi there. This is a hit in his pocket";
String subString = "Hi";
String regEx = "\\b" + subString + "\\b";
int count = 0; // To hold the word count
// Compile the regular expression
Pattern p = Pattern.compile(regEx);
// See if there are matches of subString within the
// input string utilizing the compiled pattern
Matcher m = p.matcher(inputString);
// Count the matches found
while (m.find()) {
count++;
}
//Display the count result...
System.out.println(count);
控制台將顯示: 1
同樣,上面的代碼是字母區分大小寫的。 換句話說,如果提供的子字符串是“hi”,則控制台中的顯示將為0,因為“hi”與“Hi”不同, “Hi”實際上包含在輸入字符串中作為第一個字。 如果你想忽略字母大小寫,那么只需將輸入字符串和提供的子字符串轉換為全大寫或全小寫,例如:
String inputString = "Hi there. This is a hit in his pocket";
String subString = "this is";
String regEx = "\\b" + subString.toLowerCase() + "\\b";
int count = 0; // To hold the word count
// Compile the regular expression
Pattern p = Pattern.compile(regEx);
// See if there are matches of subString within the
// input string utilizing the compiled pattern
Matcher m = p.matcher(inputString.toLowerCase());
// Count the matches found
while (m.find()) {
count++;
}
//Display the count result...
System.out.println(count);
控制台將顯示: 1
正如您在上面的兩個最新代碼示例中所看到的那樣,使用了"\\\\bHi\\\\b"
的正則表達式(RegEx)(在代碼中,變量用於代替Hi ),這就是它的含義:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.