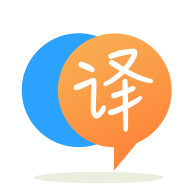
[英]How can I serve an HTML file from Azure blob storage in a web app using NodeJS?
[英]How can I parse Azure Blob URI in nodejs/javascript?
我需要在nodejs中解析Azure Blob URI,並提取存儲帳戶名稱,容器名稱和Blob名稱。
我同時研究了azure-sdk-for-node和azure-storage-node,但沒有找到這樣做的方法。
如果Blob URI無效,我也想檢測到這一點,因此正則表達式(如果可能)可能是個不錯的選擇。
Blob URI的一些示例:
https://myaccount.blob.core.windows.net/mycontainer/myblob
http://myaccount.blob.core.windows.net/myblob
https://myaccount.blob.core.windows.net/$root/myblob
通過遵循Azure的規范 ,我想出了以下函數( gist ),該函數使用regex解析blob uri,如果blob uri無效,它還會引發錯誤。
存儲帳戶名和容器名應該完全正確/精確,只是我定義的Blob名稱有些松散,因為定義起來比較復雜。
/**
* Validates and parses given blob uri and returns storage account,
* container and blob names.
* @param {string} blobUri - Valid Azure storage blob uri.
* @returns {Object} With following properties:
* - {string} storageAccountName
* - {string} containerName
* - {string} blobName
* @throws {Error} If blobUri is not valid blob uri.
*/
const parseAzureBlobUri = (blobUri) => {
const ERROR_MSG_GENERIC = 'Invalid blob uri.'
const storageAccountRegex = new RegExp('[a-z0-9]{3,24}')
const containerRegex = new RegExp('[a-z0-9](?!.*--)[a-z0-9-]{1,61}[a-z0-9]')
const blobRegex = new RegExp('.{1,1024}') // TODO: Consider making this one more precise.
const blobUriRegex = new RegExp(
`^http[s]?:\/\/(${ storageAccountRegex.source })\.blob.core.windows.net\/`
+ `(?:(\$root|(?:${ containerRegex.source }))\/)?(${ blobRegex.source })$`
)
const match = blobUriRegex.exec(blobUri)
if (!match) throw Error(ERROR_MSG_GENERIC)
return {
storageAccountName: match[1],
// If not specified, then it is implicitly root container with name $root.
containerName: match[2] || '$root',
blobName: match[3]
}
}
您可以使用url.parse
。
對我而言,主要原因是避免使用正則表達式,並且也更易於理解,閱讀和修改。
這是一個示例代碼:
const url = require('url')
const parseAzureBlobUri = (blobUrl) => {
let uri = url.parse(blobUrl)
// Extract the storage account name
let storageAccountName = uri.hostname.split('.')[0]
// Remove the 1st trailing slash then extract segments
let segments = uri.pathname.substring(1).split('/')
// If only one segment, this is the blob name
if(segments.length === 1){
return {
storageAccountName,
containerName: '$root',
blobName: segments[0]
}
}
// get the container name
let containerName = segments[0]
// Remove the containername from the segments
segments.shift()
return {
storageAccountName,
containerName,
blobName: segments.join('/')
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.