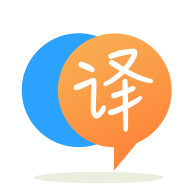
[英]ECDSA Verify Signature in C# using public key and signature from Java
[英]In C# How to verify JWT using ECDSA public key which was signed with ECDSA private key
我想驗證通過 ECDSA SHA256 算法簽名的 jwt。 我使用的點網庫是 System.IdentityModel.Tokens.Jwt 。 我可以訪問公鑰。
如果您有權訪問公鑰,一種方法是利用JwtSecurityTokenHandler
類,該類也用於生成 Jwt。 它位於相同的命名空間 ( System.IdentityModel.Tokens
) 下。
示例代碼:
bool ValidateEcdsa384JwtToken(string tokenString, ECDsa pubKey)
{
try
{
var securityToken = new JwtSecurityToken(tokenString);
var securityTokenHandler = new JwtSecurityTokenHandler();
var validationParameters = new TokenValidationParameters() {
ValidIssuer = securityToken.Issuer,
ValidAudience = securityToken.Audiences.First(),
IssuerSigningKey = new ECDsaSecurityKey(pubKey)
};
SecurityToken stoken;
var claims = securityTokenHandler.ValidateToken(tokenString, validationParameters, out stoken);
return true;
}
catch (System.Exception e)
{
return false;
}
}
// using System.Security.Cryptography;
// using System.Text.RegularExpressions;
// using Microsoft.IdentityModel.Tokens;
// `openssl ecparam -name prime256v1 -genkey -noout -out es256-private.pem`
// `openssl ec -in es256-private.pem -pubout -out es256-public.pem`
const string es256PublicKey = @"-----BEGIN PUBLIC KEY-----
MFkwEwYHKoZIzj0CAQYIKoZIzj0DAQcDQgAEWWNSXIcIZ7iKiSnNVOzzkZEEpDvf
sPux0GlqPl1aamHIiZgj364xcIrmaazMb1dsZaNBGLyvyJk0xRKk7BSSrg==
-----END PUBLIC KEY-----";
// Remove all whitespace and also remove '-----XXX YYY-----'
// '\s+' Matches one or more whitespace characters
// '|' Is a logical OR operator
// '(?: )' Is a non-capturing group
// '-+[^-]+-+' Matches one or more hyphens, followed by one or more non-hyphens, followed by one or more hyphens
var pemData = Regex.Replace(es256PublicKey, @"\s+|(?:-+[^-]+-+)", string.Empty);
var keyBytes = Convert.FromBase64String(keyData);
// Example of DER encoded P-256 curve at https://tools.ietf.org/html/rfc5759
var pointBytes = keyBytes.TakeLast(64);
var pubKeyX = pointBytes.Take(32).ToArray();
var pubKeyY = pointBytes.TakeLast(32).ToArray();
var ecdsa = ECDsa.Create(new ECParameters
{
Curve = ECCurve.NamedCurves.nistP256,
Q = new ECPoint
{
X = pubKeyX,
Y = pubKeyY
}
});
var tokenValidationParameters = new TokenValidationParameters
{
IssuerSigningKey = new ECDsaSecurityKey(ECDsaPublic.Value),
ValidAlgorithms = new[]
{
@"ES256"
}
};
// https://stackoverflow.com/a/39974628/414655
var handler = new JwtSecurityTokenHandler();
var claimsPrincipal = handler.ValidateToken(jwt, tokenValidationParameters, out SecurityToken securityToken);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.