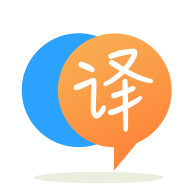
[英]Consuming non-asmx SOAP 1.1 Web Service in C# with Header Security
[英]passing parameter to asmx web service while consuming from c#
我已經創建了如下的asmx Web服務
[WebMethod]
[ScriptMethod(UseHttpGet = true, ResponseFormat = ResponseFormat.Json)]
public void GetEmployeesJSONByID(int sId)
{
try
{
string sResult = string.Empty;
List<Employee> lstEmp = new List<Employee>();
List<Employee> lstEmpNew = new List<Employee>();
lstEmp.Add(new Employee { Id = 101, Name = "Nitin", Salary = 10000 });
lstEmp.Add(new Employee { Id = 102, Name = "Dinesh", Salary = 20000 });
switch (sId)
{
case 101:
lstEmpNew.Add(new Employee { Id = 101, Name = "Nitin", Salary = 10000 });
break;
case 102:
lstEmpNew.Add(new Employee { Id = 102, Name = "Dinesh", Salary = 20000 });
break;
default:
break;
}
JavaScriptSerializer js = new JavaScriptSerializer();
sResult = js.Serialize(lstEmpNew);
Context.Response.Write(sResult);
}
catch (Exception ex)
{
Context.Response.Write(ex.Message.ToString());
}
}
我想在C#中使用此Web服務。 所以我用下面的代碼
string url = http://XXXXXX/SampleWebService/Service.asmx/GetEmployeesJSONByID;
HttpWebRequest webreq = (HttpWebRequest)WebRequest.Create(url);
webreq.Headers.Clear();
webreq.Method = "GET";
Encoding encode = Encoding.GetEncoding("utf-8");
HttpWebResponse webres = null;
webres = (HttpWebResponse)webreq.GetResponse();
Stream reader = null;
reader = webres.GetResponseStream();
StreamReader sreader = new StreamReader(reader, encode, true);
string result = sreader.ReadToEnd();
sOutput = result;
如何從C#將此sId作為參數傳遞來測試此Web服務?
要使用網絡服務,請右鍵單擊您的項目,然后選擇“添加服務參考”並添加您的網址
http://XXXXXX/SampleWebService/Service.asmx
它將在您的項目中創建代理類。 然后,在后面的代碼中創建該代理類的實例,並將必需的參數傳遞給調用方法。 例如
ClsWebserviceProxy objProxy = new ClsWebserviceProxy();
objProxy.GetEmployeesJSONByID(5);
要使用動態網址,您可以嘗試以下代碼,
public void add()
{
var _url = "http://www.dneonline.com/calculator.asmx";
var _action = "http://www.dneonline.com/calculator.asmx?op=Add";
string methodName = "Add";
XmlDocument soapEnvelopeXml = CreateSoapEnvelope(methodName);
HttpWebRequest webRequest = CreateWebRequest(_url, _action);
InsertSoapEnvelopeIntoWebRequest(soapEnvelopeXml, webRequest);
// begin async call to web request.
IAsyncResult asyncResult = webRequest.BeginGetResponse(null, null);
// suspend this thread until call is complete. You might want to
// do something usefull here like update your UI.
asyncResult.AsyncWaitHandle.WaitOne();
// get the response from the completed web request.
string soapResult;
using (WebResponse webResponse = webRequest.EndGetResponse(asyncResult))
{
using (StreamReader rd = new StreamReader(webResponse.GetResponseStream()))
{
soapResult = rd.ReadToEnd();
}
Console.Write(soapResult);
}
}
private static HttpWebRequest CreateWebRequest(string url, string action)
{
HttpWebRequest webRequest = (HttpWebRequest)WebRequest.Create(url);
webRequest.Headers.Add("SOAPAction", action);
webRequest.ContentType = "text/xml;charset=\"utf-8\"";
webRequest.Accept = "text/xml";
webRequest.Method = "POST";
return webRequest;
}
private static XmlDocument CreateSoapEnvelope(string methodName)
{
XmlDocument soapEnvelopeDocument = new XmlDocument();
string soapStr =
@"<?xml version=""1.0"" encoding=""utf-8""?>
<soap:Envelope xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance""
xmlns:xsd=""http://www.w3.org/2001/XMLSchema""
xmlns:soap=""http://schemas.xmlsoap.org/soap/envelope/"">
<soap:Body>
<{0} xmlns=""http://tempuri.org/"">
{1}
</{0}>
</soap:Body>
</soap:Envelope>";
string postValues = "";
Dictionary<string, string> Params = new Dictionary<string, string>();
//< "name" > Name of the WebMethod parameter (case sensitive)</ param >
//< "value" > Value to pass to the paramenter </ param >
Params.Add("intA", "5"); // Add parameterName & Value to dictionary
Params.Add("intB", "10");
foreach (var param in Params)
{
postValues += string.Format("<{0}>{1}</{0}>", param.Key, param.Value);
}
soapStr = string.Format(soapStr, methodName, postValues);
soapEnvelopeDocument.LoadXml(soapStr);
return soapEnvelopeDocument;
}
private static void InsertSoapEnvelopeIntoWebRequest(XmlDocument soapEnvelopeXml, HttpWebRequest webRequest)
{
try
{
using (Stream stream = webRequest.GetRequestStream())
{
soapEnvelopeXml.Save(stream);
}
}
catch (Exception ex)
{
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.