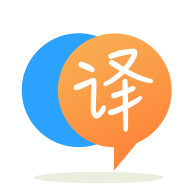
[英]How to make default focus on the search bar on a web page, as Google does
[英]Move to first 'default' focus of web page
假設您有網站,當您刷新它時,如果您檢查哪個元素具有焦點,請使用以下代碼:
setInterval(function () {
console.log('ID: ', $(document.activeElement).attr('id') + ' Tag: ' + $(document.activeElement).prop('tagName')
+ ' C: ' + $(document.activeElement).attr('class'));
}, 2000);
您將看到元素的標簽是“BODY”元素。 您如何使用 javascript 將焦點恢復到同一元素,因為諸如$('body').focus();
不起作用。
編輯:它還應該重置文檔的“焦點”流。 因此,在您單擊 TAB 后,它將聚焦相同的元素,就像您刷新頁面然后單擊 TAB 一樣。
編輯 2:我需要將某些操作(如 keyDown)的焦點重置為默認狀態 - 加載頁面后的狀態。 根據我的研究,我知道加載頁面后聚焦的元素是“正文”,然后在單擊 Tab 后,您網站的焦點流中的第一個元素被聚焦。 我不能使用 $('body').focus(); - 它不聚焦正文並且不重置文檔的當前焦點流。
編輯 3:到目前為止,我們已經設法使用以下代碼以某種方式將焦點重置為網站的body
元素: document.activeElement.blur();
所以我上面的腳本會說body
是焦點,但當您使用鍵盤導航網站(TAB 按鈕)時,它不會重置當前焦點的實際流。 可以有解決方法並選擇您想要的指定元素,但這不是問題的答案。 什么是將網站的鍵盤導航流程重置為默認狀態而不刷新頁面的通用機制?
編輯 4: https : //jsfiddle.net/4qjb5asw/5/
您可以通過執行document.activeElement.blur();
清除活動元素的焦點,而不是專門聚焦<body>
document.activeElement.blur();
這應該將焦點恢復到<body>
元素並重置焦點流。
在下面的代碼段中四處單擊以查看當前的document.activeElement
是什么。
$("#reset").on("click", function() { document.activeElement.blur(); logActiveElement(); }); $("form").children().on("focus", logActiveElement); function logActiveElement() { console.log("The active element is now: " + $(document.activeElement).prop('tagName')); }
.blur { padding: 5px; border: 2px solid blue; margin-top: 20px; }
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script> <form> <input type="text" /> <textarea></textarea> <button type="button">Test Button</button> </form> <div class="blur"> <button id="reset">RESET ACTIVE ELEMENT</button> </div>
如果您想要精細控制焦點,請考慮使用tabIndex
[1] 屬性。 基本上,它允許您通過為每個可以具有焦點的元素分配數字來指定 Tab 鍵順序。 一個額外的好處是tabIndex
使您能夠指定要采用的元素,這些元素可能不是默認的——正是您需要的。
Tab 鍵順序和焦點對於可訪問性非常重要,因為用戶沒有指點設備。 因此,此標簽旨在讓您精確控制焦點。 它使您能夠指定頁面上的所有可聚焦元素。 在您的情況下,它提供了所有可聚焦元素的句柄,使您能夠在需要時在需要的地方專門設置焦點。
不確定您使用的是哪個框架,但 jQuery 是跨瀏覽器兼容性的候選者。
從jQuery .focus()
頁面:
在最近的瀏覽器版本中,可以通過顯式設置元素的 tabindex 屬性將事件擴展為包括所有元素類型。 [2]
也許.focus()
基於 tabindex; 像這樣的東西:
$('input[tabindex='+ntabindex+']').focus(); // [3]
如果使用此功能,請務必檢查您的 Tab 鍵順序。 瀏覽您的頁面並確保您已正確排序所有可聚焦元素。
聽起來您只是想在某些事件上重置文檔的默認焦點,例如特定鍵上的keydown
,以便用戶可以在觸發事件后開始從頂部切換文檔。
您可以使用以下命令重置文檔的默認焦點:
document.activeElement.blur()
您可以添加要觸發該函數的任何事件偵聽器。 對於轉義鍵上的keydown
事件,它將是:
document.addEventListener('keydown', (event) => {
if (event.key === 'Escape') {
document.activeElement.blur()
}
});
重要提示:請注意,如果您在諸如 SO 代碼段或 jsfiddle 之類的嵌入式工具中對此進行測試,則需要添加更多代碼以將焦點設置為該工具的元素包裝器以模擬顯示區域表示時會發生的情況整個文檔(否則,您會將焦點重置到主文檔,在 SO 的情況下,您將開始在頁面上的菜單、問題、答案、評論和其他元素之間切換,然后才能到達代碼段)。
用於測試的示例代碼段將焦點重置到代碼段中的包裝器元素,以便制表符將從代碼段的開頭(而不是從該 SO 頁面的文檔的開頭)重新開始:
/* * All code below referencing the "snippet" variable is only * here to help us pretend that the snippet result * represents the entire document (not needed in a * regular implementation). */ const snippet = document.querySelector('#snippet'); snippet.focus(); const btn = document.querySelector('#button1'); const resetFocus = () => { document.activeElement.blur(); snippet.focus(); } document.addEventListener('keydown', (event) => { if (event.key === 'Escape') { resetFocus(); } }); btn.addEventListener('click', (event) => { resetFocus(); });
<!-- The "snippet" wrapper div with tabindex attribute is only here to help us pretend that the snippet result represents the entire document (not needed in a regular implementation). The tabindex helps ensure that the wrapper div is focusable. --> <div id="snippet" tabindex="0"> <div>Tab to or select input to focus. To reset focus: </div> <ul> <li>Press escape key.</li> <li>Or, click "Reset" button.</li> </ul> <div> <input id="input1" type="text" name="input1" /> </div> <div> <input id="input2" type="text" name="input2" /> </div> <button id="button1">Reset</button> </div>
你的問題可以分為三部分
如果您可以控制屏幕上的元素,或者它是可預測的靜態元素。 一個簡單的document.querySelector("#id").focus()
將聚焦默認元素。
如果不是這種情況,您需要一些可能很復雜的檢測。
瀏覽器將首先找到具有最小正非零[tabIndex]
編號的元素。 如果#anchor
存在,它會在它之后使用第一個可聚焦元素。 否則第一個可聚焦元素a[href],button,input,object,area[href]
來自 body。
因為您可能不想關注元素,但希望它成為接下來的焦點。 您可以在它之前注入一個不可見元素,使用可選的 tabIndex hack,並專注於您的不可見元素。 並在模糊時消除注入的元素。
我在頁面頂部有一個標題div
元素。 每次將新內容加載到頁面上時,我想將焦點移回頁眉,因為它位於文檔的頂部,
<div tabindex="-1" role="header">
<a href="#content">I always want this to be tabbed first</a>
</div>
重要的一點是將tabindex
設置為-1
,這會將其從瀏覽器的默認焦點流中刪除,然后允許我使用 javascript 以編程方式設置焦點。
document.querySelector('div[role="header"]').focus();
每次顯示新內容時都會運行此代碼段,這意味着使用鍵盤的人可以在單頁應用程序中為每個頁面獲得一致的起點。
現在,當我按下 Tab 鍵時, <a>
標簽總是首先被聚焦。
這將使您的指定元素保持專注
$(document).ready(function(){
setInterval(function(){
var focusbox = document.getElementById("element_to_focus");
focusbox.focus();
});
})
帶有 tabindex 屬性的“snippet”包裝 div 只是為了幫助我們假裝片段結果代表整個文檔(在常規實現中不需要)。 標簽索引有助於確保包裝 div 是可聚焦的。
創建一個帶有href="#"
和style="display: none; position: absolute;"
在你身體的開始。 當您想重置焦點時:顯示元素,將其聚焦並將其隱藏。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.