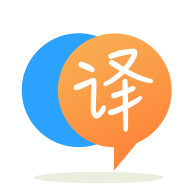
[英]Error in ASP.NET Core : no service for type […] has been registered
[英]ASP.NET CORE 2.1 Identity has been registered
我對Identity ASP.net Core 2.1有問題。 我在startup.cs中創建角色。 運行時顯示錯誤
AggregateException:發生一個或多個錯誤。 (沒有注冊用於類型'Microsoft.AspNetCore.Identity.RoleManager`1 [Microsoft.AspNetCore.Identity.IdentityRole]'的服務。)System.Threading.Tasks.Task.Wait(int毫秒超時,CancellationToken cancelToken)System.Threading。 Startup.cs + CreateRoles(serviceProvider).Wait()中的Tasks.Task.Wait()ContosoUniversity.Startup.Configure(IApplicationBuilder應用,IHostingEnvironment env,IServiceProvider serviceProvider) Microsoft.AspNetCore.Hosting.ConventionBasedStartup.Configure(IApplicationBuilder應用程序)Microsoft.AspNetCore.Server.IISIntegration.IISSetupFilter + <> c__DisplayClass4_0.b__0(IApplicationBuilder應用程序)Microsoft.AspNetCore.HostFilteringStartupFilter + <> c__DisplayClass0_0.b__0(IApplicationBuilder) .Internal.AutoRequestServicesStartupFilter + <> c__DisplayClass0_0.b__0(IApplicationBuilder構建器)Microsoft.AspNetCore.Hosting.Internal.WebHost.BuildApplication()
Statup.cs文件
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.HttpsPolicy;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.EntityFrameworkCore;
using ContosoUniversity.Models;
using Microsoft.AspNetCore.Identity;
using ContosoUniversity.Areas.Identity.Data;
namespace ContosoUniversity
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.Configure<CookiePolicyOptions>(options =>
{
// This lambda determines whether user consent for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
});
services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
services.AddDbContext<SchoolContext>(options =>
options.UseSqlServer(Configuration.GetConnectionString("SchoolContext")));
//services.AddIdentity<ContosoUniversityUser, IdentityRole>()
// .AddEntityFrameworkStores<IdentityContext>()
// .AddDefaultTokenProviders();
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env, IServiceProvider serviceProvider)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseAuthentication();
app.UseMvc();
CreateRoles(serviceProvider).Wait();
}
public async Task CreateRoles(IServiceProvider serviceProvider)
{
//adding custom roles
var RoleManager = serviceProvider.GetRequiredService<RoleManager<IdentityRole>>();
//serviceProvider.GetService<RoleManager<IdentityRole>>();
var UserManager = serviceProvider.GetRequiredService<UserManager<ContosoUniversityUser>>();
string[] roleNames = { "Admin", "Manager", "Member" };
IdentityResult roleResult;
foreach (var roleName in roleNames)
{
var roleExist = await RoleManager.RoleExistsAsync(roleName);
if (!roleExist)
{
roleResult = await RoleManager.CreateAsync(new IdentityRole(roleName));
}
}
var poweruser = new ContosoUniversityUser
{
UserName = Configuration.GetSection("UserSettings")["UserEmail"],
Email = Configuration.GetSection("UserSettings")["UserEmail"]
};
string UserPassword = Configuration.GetSection("UserSettings")["UserPassword"];
var _user = await UserManager.FindByEmailAsync(Configuration.GetSection("UserSettings")["UserEmail"]);
if (_user == null)
{
var createPowerUser = await UserManager.CreateAsync(poweruser, UserPassword);
if (createPowerUser.Succeeded)
{
await UserManager.AddToRoleAsync(poweruser, "Admin");
}
}
}
}
}
您試圖使用asp.net-identity而不將其注冊到您的容器中。
在configure-service方法中,取消注釋這些行
services.AddIdentity<ContosoUniversityUser, IdentityRole>();
.AddEntityFrameworkStores<IdentityContext>()
.AddDefaultTokenProviders();
現在,服務提供者將了解這些服務,因為該擴展方法會為您注冊它們。
另外,不要忘記遷移數據庫,這樣它就可以了解用戶,角色等信息。
我嘗試在ConfigureServices中添加以下代碼。 這可以幫助我運行:
var builder = services.AddIdentityCore<ContosoUniversityUser>(opt =>
{
// Configure Password Options
opt.Password.RequireDigit = true;
}
);
builder = new IdentityBuilder(builder.UserType, typeof(IdentityRole), builder.Services);
builder.AddRoleValidator<RoleValidator<IdentityRole>>();
builder.AddRoleManager<RoleManager<IdentityRole>>();
builder.AddSignInManager<SignInManager<ContosoUniversityUser>>();
builder.AddEntityFrameworkStores<IdentityContext>().AddDefaultTokenProviders();
services.TryAddSingleton<IHttpContextAccessor, HttpContextAccessor>();
看來您可以通過Asp.Net Core Identity Library
實現Identity
。
您可以檢查項目以找到ProjectName-> Areas-> Identity-> IdentityHostingStartup 。 更改Configure
如下所示:
public class IdentityHostingStartup : IHostingStartup
{
public void Configure(IWebHostBuilder builder)
{
builder.ConfigureServices((context, services) => {
services.AddDbContext<CoreAppContext>(options =>
options.UseSqlServer(
context.Configuration.GetConnectionString("CoreAppContextConnection")));
services.AddIdentity<ContosoUniversityUser, IdentityRole>()
.AddEntityFrameworkStores<CoreAppContext>();
});
}
}
更新
根本原因是, services.AddDefaultIdentity
沒有將IdentityRole
添加到IServiceCollection
,請檢查此源代碼IdentityServiceCollectionUIExtensions ,該代碼調用AddIdentityCore 。
嘗試下面的代碼。
public class IdentityHostingStartup : IHostingStartup
{
public void Configure(IWebHostBuilder builder)
{
builder.ConfigureServices((context, services) => {
services.AddDbContext<IdentityContext>(options =>
options.UseSqlServer(
context.Configuration.GetConnectionString("IdentityContextConnection")));
services.AddDefaultIdentity<ContosoUniversityUser>()
.AddRoles<IdentityRole>() // Add IdentityRole to ServiceCollection
.AddEntityFrameworkStores<IdentityContext>();
});
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.