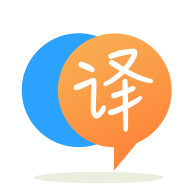
[英]Enable/disable Button on TextChanged event of TextBox in WPF by XAML
[英]disable/enable a button depending on textbox in xaml
我有一個文本框
<TextBox x:Name="searchTextBox" Width="200" Height="30" HorizontalAlignment="Left"/>
和兩個按鈕
<Button x:Name="previous" Style="{StaticResource AppBarButtonStyle}" Tapped="OnOptionItemTapped" IsEnabled="False">
<Image Source="/Assets/images/left_arrow.png"/>
</Button>
<Button x:Name="next" Style="{StaticResource AppBarButtonStyle}" Tapped="OnOptionItemTapped" IsEnabled="False">
<Image Source="/Assets/images/right_arrow.png"/>
</Button>
是否有一個簡單的解決方案可以通過 TextBox 啟用/禁用按鈕?
例如,如果 TextBox 為空,則 Buttons 被禁用。 如果 TextBox 不為空,則啟用按鈕。
您可以應用文本更改事件,在每次更改時檢查輸入。
<TextBox x:Name="searchTextBox" Width="200" Height="30" HorizontalAlignment="Left" TextChanged="TextBox_TextChanged" />
如果文本是您需要的方式,您可以啟用/禁用按鈕。
public void TextBox_TextChanged(object sender, TextChangedEventArgs e)
{
if (searchTextBox.Text == result)
next.IsEnabled = false;
}
編輯:除了這種方法背后的代碼之外,您還可以了解MVVM設計模式。 其他答案部分使用了MVVM 中常見的做法。
網絡上有各種很好的教程。
使用綁定+轉換器怎么樣? 我想這個概念也適用於 UWP。
例如,您有一個帶有SearchText
屬性的視圖模型,該屬性綁定到 TextBox 中的文本。 然后您可以執行以下操作:
<Window.Resources>
<local:StringToBoolConverter x:Key="StringToBoolConverter" />
</Window.Resources>
...
<TextBox x:Name="searchTextBox" Width="200" Height="30" HorizontalAlignment="Left" Text="{Binding SearchText}"/>
<Button x:Name="previous" IsEnabled="{Binding SearchText, Converter={StaticResource StringToBoolConverter}}"/>
轉換器代碼非常簡單:
public class StringToBoolConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
return !string.IsNullOrEmpty(value?.ToString());
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
另一種方法是對按鈕使用命令模式。 ICommand
接口具有CanExecute
方法,可以根據返回值禁用或啟用您的按鈕。 請參閱互聯網或此處的示例。
對 IsEnabled 使用綁定。
<Button x:Name="previous" Style="{StaticResource AppBarButtonStyle}"
Tapped="OnOptionItemTapped" IsEnabled="{Binding ElementName=searchTextBox,
Path=Text.Length, Mode=OneWay}"></Button>
您也可以使用數據觸發器,但以上是最簡單的。 不需要轉換器。
我使用Xamarin Forms (XAML) 中的轉換器來實現這一點,這應該與您的場景相同/相似。 通常是IsEnabled
和Opacity
的組合,因為我喜歡使禁用的按鈕更透明。
例如,您可以創建兩個轉換器來解釋一個字符串(輸入字段中的文本)。
第一個轉換器會判斷文本是否為空,有文本時返回true。
public class StringToBoolConverter
: IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
return !string.IsNullOrEmpty(value?.ToString());
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
第二個轉換器將確定不透明度級別,當有文本時返回 100%(例如: 1.0
),當字段為空時返回0.3
。
public class StringToFloatConverter
: IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
return !string.IsNullOrEmpty(value?.ToString())? 1.0 : 0.3;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
}
現在我在App.xaml
中用更有意義的名稱標記兩個轉換器,如下所示:
<?xml version="1.0" encoding="utf-8" ?>
<Application xmlns="http://xamarin.com/schemas/2014/forms"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:d="http://xamarin.com/schemas/2014/forms/design"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:converters="clr-namespace:Sasw.EasyQr.Converters;assembly=Sasw.EasyQr"
mc:Ignorable="d"
x:Class="Sasw.EasyQr.App">
<Application.Resources>
<ResourceDictionary>
<converters:StringToBoolConverter x:Key="EnabledWhenFilledConverter"></converters:StringToBoolConverter>
<converters:StringToFloatConverter x:Key="OpaqueWhenFilledConverter"></converters:StringToFloatConverter>
</ResourceDictionary>
</Application.Resources>
</Application>
現在我可以從任何按鈕控件中引用這些轉換器,例如:
<Entry
Text = "{Binding Text}"
Placeholder="{x:Static resources:AppResources.PlaceholderEnterText}"
HorizontalOptions="FillAndExpand"
VerticalTextAlignment="Center"
VerticalOptions="End"></Entry>
<ImageButton
Command="{Binding TapClear}"
IsEnabled="{Binding Text, Converter={StaticResource EnabledWhenFilledConverter}}"
Source ="backspace.png"
WidthRequest="30"
Opacity="{Binding Text, Converter={StaticResource OpaqueWhenFilledConverter}}"
BackgroundColor="Transparent"
HorizontalOptions="End"
VerticalOptions="CenterAndExpand"></ImageButton>
並查看按鈕如何在條目中輸入文本時自動啟用並使其不透明,以及在刪除文本時禁用並使其透明。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.