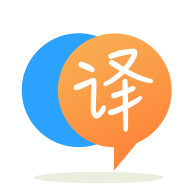
[英]How to create triggers which accepts external parameters in SQL Server?
[英]How to create a constructor which accepts up to 4 arguments?
我正在創建一個最多接受4個參數的屬性。
我用這種方式編碼:
internal class BaseAnnotations
{
public const string GET = "GET";
public const string POST = "POST";
public const string PATCH = "PATCH";
public const string DELETE = "DELETE";
public class OnlyAttribute : Attribute
{
public bool _GET = false;
public bool _POST = false;
public bool _PATCH = false;
public bool _DELETE = false;
public OnlyAttribute(string arg1)
{
SetMethod(arg1);
}
public OnlyAttribute(string arg1, string arg2)
{
SetMethod(arg1);
SetMethod(arg2);
}
public OnlyAttribute(string arg1, string arg2, string arg3)
{
SetMethod(arg1);
SetMethod(arg2);
SetMethod(arg3);
}
public OnlyAttribute(string arg1, string arg2, string arg3, string arg4)
{
SetMethod(arg1);
SetMethod(arg2);
SetMethod(arg3);
SetMethod(arg4);
}
public void SetMethod(string arg)
{
switch (arg)
{
case GET: _GET = true; break;
case POST: _POST = true; break;
case PATCH: _PATCH = true; break;
case DELETE: _DELETE = true; break;
}
}
}
}
我需要像這樣使用它:
public class ExampleModel : BaseAnnotations
{
/// <summary>
/// Example's Identification
/// </summary>
[Only(GET, DELETE)]
public long? IdExample { get; set; }
// ...
有沒有辦法只在一個構造函數中編碼上面的4個構造函數以避免重復?
我正在思考JavaScript的擴展運算符(...args) => args.forEach(arg => setMethod(arg))
。
提前致謝。
我打算建議在這里重新思考你的設計。 考慮:
[Flags]
public enum AllowedVerbs
{
None = 0,
Get = 1,
Post = 2,
Patch = 4,
Delete = 8
}
public class OnlyAttribute : Attribute
{
private readonly AllowedVerbs _verbs;
public bool Get => (_verbs & AllowedVerbs.Get) != 0;
public bool Post => (_verbs & AllowedVerbs.Post) != 0;
public bool Patch => (_verbs & AllowedVerbs.Patch) != 0;
public bool Delete => (_verbs & AllowedVerbs.Delete ) != 0;
public OnlyAttribute(AllowedVerbs verbs) => _verbs = verbs;
}
然后呼叫者可以使用:
[Only(AllowedVerbs.Get)]
要么
[Only(AllowedVerbs.Post | AllowedVerbs.Delete)]
很好的答案,但考慮使用4個屬性。 對於您的示例,這可能有效。
public class GetAttribute: Attribute {}
public class PostAttribute: Attribute {}
public class PatchAttribute: Attribute {}
public class DeleteAttribute: Attribute {}
[GET] [DELETE]
public long? IdExample { get; set; }
這很簡單直接。 當然有更多屬性,但您可能需要更多實例。
每個屬性都有一個默認構造函數。 僅僅存在每個操作的屬性就足以傳達允許的內容。
你可以試試這個
public OnlyAttribute(params string[] parameters)
{
foreach(var p in parameters) SetMethod(p);
}
public OnlyAttribute(params string[] parameters)
{
if (parameters.Length > 4) throw new ArugumentException(nameof(parameters));
foreach (var param in parameters)
{
SetMethod(param);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.