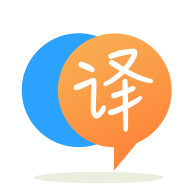
[英]ItemsControl with WrapPanel as ItemsPanel - combine a “static” child and ItemsSource
[英]How to redraw ItemsControl ItemsPanel when property in the ItemsSource collection is changed?
我制作了一個自定義的WeightedUniformGrid類,將其用作ItemsControl中的ItemsPanel。 使用ItemsSource集合中對象中的Weight屬性對每個網格元素進行加權。
但是,當我在視圖模型中更改Weight屬性時,它不會立即顯示在View中。 我必須更改用新值繪制的WeightedUniformGrid的窗口大小。
如何獲取ItemsSource集合中的屬性更改以導致ItemsControl重繪? 我在想也許在WeightedUniformGrid中添加一個DependencyProperty,它將同時影響AffectsArrange和AffectsMeasure。 但是我不確定我可以綁定到什么。
我已經搜索了很長時間,並且有些問題很相似(例如這個問題)。 但是我一直無法適應他們的需求。
MainWindow.xaml:
<ItemsControl ItemsSource="{Binding Path=ElementGroupCollection}">
<ItemsControl.ItemsPanel>
<ItemsPanelTemplate>
<local:WeightedUniformGrid Rows="2" />
</ItemsPanelTemplate>
</ItemsControl.ItemsPanel>
<ItemsControl.ItemTemplate>
<DataTemplate>
<Border BorderBrush="Black" BorderThickness="1"
RenderOptions.EdgeMode="Aliased">
<Viewbox Stretch="Uniform">
<TextBlock Text="{Binding Path=Number}" Foreground="Black" FontSize="20"
HorizontalAlignment="Center" VerticalAlignment="Center"/>
</Viewbox>
</Border>
</DataTemplate>
</ItemsControl.ItemTemplate>
</ItemsControl>
MainWindowVM.cs:
public class MainWindowVM
{
public MainWindowVM()
{
_elementGroupCollection = new ObservableCollection<ElementGroup>()
{
new ElementGroup(1, 60),
new ElementGroup(2, 150),
new ElementGroup(3, 90),
new ElementGroup(4, 80),
new ElementGroup(5, 60),
new ElementGroup(6, 160)
};
}
private ObservableCollection<ElementGroup> _elementGroupCollection;
public ObservableCollection<ElementGroup> ElementGroupCollection
{
get { return _elementGroupCollection; }
}
}
ElementGroup.cs:
public class ElementGroup : INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
public ElementGroup(int number, double? weight)
{
Number = number;
Weight = (weight >= 0) ? weight : null;
}
public int Number { get; }
private double? _weight;
public double? Weight
{
get { return _weight; }
set { SetNotify(ref _weight, value); }
}
public void SetNotify<T>(ref T storage,
T value,
[CallerMemberName] string propertyName = null)
{
storage = value;
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
WeightedUniformGrid.cs:
public class WeightedUniformGrid : UniformGrid
{
protected override Size MeasureOverride(Size constraint)
{
var size = base.MeasureOverride(constraint);
double elementsPerRow = Math.Ceiling((double)Children.Count / Rows);
double elementHeight = size.Height / Rows;
double unweightedElementWidth = size.Width / elementsPerRow;
for (int i = 0; i < Children.Count; ++i)
{
var child = (FrameworkElement)Children[i];
var dc = child.DataContext;
int rowNumber = (int)Math.Floor(i / elementsPerRow);
double? weight = dc.GetType().GetProperty("Weight")?.GetValue(dc, null) as double?;
if (weight == null) { weight = 100; }
double weightedElementWidth = unweightedElementWidth * (double)weight / 100;
child.Measure(new Size(weightedElementWidth, elementHeight));
}
return size;
}
protected override Size ArrangeOverride(Size arrangeSize)
{
var size = base.ArrangeOverride(arrangeSize);
int elementsPerRow = (int)Math.Ceiling((double)Children.Count / Rows);
double elementHeight = size.Height / Rows;
double unweightedElementWidth = size.Width / elementsPerRow;
double[] accumulatedWidthPerRow = new double[Rows];
for (int i = 0; i < Children.Count; ++i)
{
var child = (FrameworkElement)Children[i];
var dc = child.DataContext;
int rowNumber = i / elementsPerRow;
double? weight = dc.GetType().GetProperty("Weight")?.GetValue(dc, null) as double?;
if (weight == null) { weight = 100; }
double weightedElementWidth = unweightedElementWidth * (double)weight / 100;
child.Arrange(new Rect(new Point(accumulatedWidthPerRow[rowNumber], rowNumber * elementHeight),
new Point(accumulatedWidthPerRow[rowNumber] + weightedElementWidth, (rowNumber + 1) * elementHeight)));
accumulatedWidthPerRow[rowNumber] += weightedElementWidth;
}
return size;
}
}
您的WeightedUniformGrid不得直接訪問其子元素的DataContext中的Weight屬性。 除此之外,這是一種不好的做法,它將無法正常工作,因為沒有機制可以在視圖模型項的“權重”更改時強制布局通過。
相反,應該有一個綁定到Weight的附加屬性。 附加屬性的FrameworkPropertyMetadataOptions
將強制進行布局傳遞。
public class WeightedUniformGrid : UniformGrid
{
public static readonly DependencyProperty WeightProperty =
DependencyProperty.RegisterAttached(
"Weight", typeof(double), typeof(WeightedUniformGrid),
new FrameworkPropertyMetadata(double.NaN,
FrameworkPropertyMetadataOptions.AffectsParentMeasure |
FrameworkPropertyMetadataOptions.AffectsParentArrange));
public static double GetWeight(UIElement element)
{
return (double)element.GetValue(WeightProperty);
}
public static void SetWeight(UIElement element, double value)
{
element.SetValue(WeightProperty, value);
}
protected override Size MeasureOverride(Size constraint)
{
var size = base.MeasureOverride(constraint);
double elementsPerRow = Math.Ceiling((double)Children.Count / Rows);
double elementHeight = size.Height / Rows;
double unweightedElementWidth = size.Width / elementsPerRow;
for (int i = 0; i < Children.Count; ++i)
{
var child = Children[i];
int rowNumber = (int)Math.Floor(i / elementsPerRow);
// get attached property value
double weight = GetWeight(child);
if (double.IsNaN(weight)) { weight = 100; }
double weightedElementWidth = unweightedElementWidth * weight / 100;
child.Measure(new Size(weightedElementWidth, elementHeight));
}
return size;
}
protected override Size ArrangeOverride(Size arrangeSize)
{
var size = base.ArrangeOverride(arrangeSize);
int elementsPerRow = (int)Math.Ceiling((double)Children.Count / Rows);
double elementHeight = size.Height / Rows;
double unweightedElementWidth = size.Width / elementsPerRow;
double[] accumulatedWidthPerRow = new double[Rows];
for (int i = 0; i < Children.Count; ++i)
{
var child = Children[i];
int rowNumber = i / elementsPerRow;
// get attached property value
double weight = GetWeight(child);
if (double.IsNaN(weight)) { weight = 100; }
double weightedElementWidth = unweightedElementWidth * (double)weight / 100;
child.Arrange(new Rect(new Point(accumulatedWidthPerRow[rowNumber], rowNumber * elementHeight),
new Point(accumulatedWidthPerRow[rowNumber] + weightedElementWidth, (rowNumber + 1) * elementHeight)));
accumulatedWidthPerRow[rowNumber] += weightedElementWidth;
}
return size;
}
}
將附加的屬性綁定到ItemContainerStyle中:
<ItemsControl ItemsSource="{Binding Path=ElementGroupCollection}">
<ItemsControl.ItemsPanel>
<ItemsPanelTemplate>
<local:WeightedUniformGrid Rows="2" />
</ItemsPanelTemplate>
</ItemsControl.ItemsPanel>
<ItemsControl.ItemContainerStyle>
<Style TargetType="ContentPresenter">
<Setter Property="local:WeightedUniformGrid.Weight" Value="{Binding Weight}"/>
</Style>
</ItemsControl.ItemContainerStyle>
...
</ItemsControl>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.