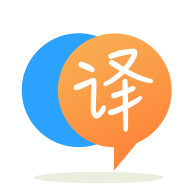
[英]Reading data from a text file into a struct with different data types c#
[英]C# retrieve different Data Types data from a text file and store it to variables
因此,我遇到了什么問題-我試圖創建一個復雜的(對我來說很復雜)的銀行管理系統。 它從一個人那里獲取各種數據。 它需要字符串和整數。 我設法將數據存儲到文本文件中。
文本文件如下所示:
編號:74016 | NAME:任何名字| 年齡:18 | 姓氏:anylastname | 平衡:300 | 帳戶類型:儲蓄帳戶|
(此數據在文本文件的一行中)
這是我存儲的方式:
var StoreInFile = new StreamWriter(@"C:\Accounts.txt", true);
StoreInFile.Write("AccountID: " + AccID + " | ");
StoreInFile.Write("NAME: " + _Name + " | ");
StoreInFile.Write("AGE: " + _Age + " | ");
StoreInFile.Write("LASTNAME: " + _LastName + " | ");
StoreInFile.Write("BALANCE: " + Balance + " | ");
StoreInFile.Write("ACCOUNT TYPE: " + accountType + " | ");
StoreInFile.WriteLine(" ");
StoreInFile.WriteLine(" ");
StoreInFile.Close();
現在的問題是如何從中獲取數據? 我如何將所有這些不同的字符串/整數存儲回變量,然后在哪里可以操縱它們?
理想情況下,我只想在登錄時輸入AccountID,然后它將所有Name,LastName,Age ...等數據自動存儲到該行代碼中的變量中。 希望我的問題清楚。
var properties = new prop();
var json = JsonConvert.SeralizeObject(properties);
public class prop
{
public string account_id { get; set;}
public string name { get; set;}
public int age { get; set;}
}
這樣的事情會幫助你很多。
您可以使用您使用的屬性創建帳戶類:
class Account
{
public int Id { get; set;}
public string Name { get; set;}
public int Age{ get; set;}
public string Lastname { get; set;}
public int Balance { get; set;}
public string AccountType { get; set;} // this is better to be enum but your example shows you have stored string
}
然后您可以創建一個Account
類的實例,讀取該文件,並使用|
並設置其屬性(在需要時解析為int):
string[] data = File.ReadAllText("filepath.txt").Split('|');
Account account = new Account();
foreach (string item in data)
{
string[] sitem = item.Split(':');
switch(sitem[0].Trim())
{
case "AccountID":
account.Id = int.Parse(sitem[1]); break;
case "Name":
account.Name = int.Parse(sitem[1]); break;
....
}
}
實際上,我建議您將其保留為Json / XML格式。 但是也許BinaryFormatter在這里可以使用一些示例來幫助您解決問題。
class Program {
static void Main (string[] args) {
// Create Object
Account account = new Account {
Id = 100,
Name = "Berkay"
};
// You can use BinaryFormatter
IFormatter formatter = new BinaryFormatter ();
Stream stream = new FileStream ("D:\\account.txt", FileMode.Create, FileAccess.Write);
formatter.Serialize (stream, account);
stream.Close ();
stream = new FileStream ("D:\\account.txt", FileMode.Open, FileAccess.Read);
Account readAccount = formatter.Deserialize(stream) as Account;
System.Console.WriteLine(readAccount.Id);
System.Console.WriteLine(readAccount.Name);
Console.ReadLine();
}
}
// Serializable attribute
[Serializable]
public class Account {
public int Id { get; set; }
public string Name { get; set; }
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.