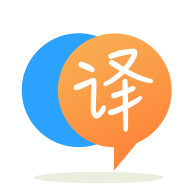
[英]How can I find all the possible combinations of a list of lists (in Python)?
[英]How can I find the locations of an item in a Python list of lists?
我想在列表列表中找到特定項目的位置。 它應該返回一個元組列表,其中每個元組代表該項的特定實例的索引。 例如:
list = [['1', '2', '4', '6'], ['7', '0', '1', '4']]
getPosition('1') #returns [(0, 0), (1, 2)]
and getPosition('7') #returns [(1,0)]
如果你想要兩種東西
你可以做以下的事情:
def get_positions(xs, item):
if isinstance(xs, list):
for i, it in enumerate(xs):
for pos in get_positions(it, item):
yield (i,) + pos
elif xs == item:
yield ()
測試這個:
>>> xs = [['1', '2', '4', '6'],
... ['7', '0', '1', '4'],
... [ [ '0', '1', '1'], ['1']]
... ]
>>> print list(get_positions(xs, '1'))
[(0, 0), (1, 2), (2, 0, 1), (2, 0, 2), (2, 1, 0)]
對於子列表和給定項目的列表,您看起來想要返回每對對的列表列表(子列表的索引,子列表中項目的索引)。 您可以使用列表推導和Python內置的enumerate()
函數來實現:
def getPosition(list, item):
return [(i, sublist.index(item)) for i, sublist in enumerate(list)]
編輯:請參閱上面/下面的@ scribble的回答。
def getPosition(list, item):
return [(i, sublist.index(item)) for i, sublist in enumerate(list)
if item in sublist]
def get_positions(xs, target):
return [(i,e.index(target)) for i,e in enumerate(xs)]
這是一個很好的起點。 大概你有某種類,比如
class SomeClass:
def __init__(self):
self.xs = [['1','2','4','6'], ['7','0','1','4']]
def get_positions(self, target):
return [(i,e.index(target)) for i,e in enumerate(self.xs)]
在這種情況下會讓你說
model = SomeClass()
model.get_position(1) # returns [(0,0), (1,2)]
請注意,在這兩種情況下,如果您的目標不在每個子列表中,您將獲得異常。 問題沒有說明這是否是所需的行為。
如果您不希望例外,如果該項目不在列表中,請嘗試此操作。 也作為發電機,因為它們很酷且功能多樣。
xs = [['1', '2', '4', '6'], ['7', '0', '1', '4']]
def get_positions(xs, item):
for i, xt in enumerate( xs ):
try: # trying beats checking
yield (i, xt.index(item))
except ValueError:
pass
print list(get_positions(xs, '1'))
print list(get_positions(xs, '6'))
# Edit for fun: The one-line version, without try:
get_positions2 = lambda xs,item: ((i,xt.index(item)) for i, xt in enumerate(xs) if item in xt)
print list(get_positions2(xs, '1'))
print list(get_positions2(xs, '6'))
前段時間我為python寫了一個庫來做列表匹配,很適合這個賬單。 它使用令牌?,+和*作為通配符,在哪里? 表示單個原子,+是非貪婪的一個或多個,*是貪婪的一個或多個。 例如:
from matching import match
match(['?', 2, 3, '*'], [1, 2, 3, 4, 5])
=> [1, [4, 5]]
match([1, 2, 3], [1, 2, 4])
=> MatchError: broken at 4
match([1, [2, 3, '*']], [1, [2, 3, 4]])
=> [[4]]
match([1, [2, 3, '*']], [1, [2, 3, 4]], True)
=> [1, 2, 3, [4]]
在此處下載: http : //www.artfulcode.net/wp-content/uploads/2008/12/matching.zip
這是一個沒有try..except的版本,返回一個迭代器和for
[['1', '1', '1', '1'], ['7', '0', '4']]
回報
[(0, 0), (0, 1), (0, 2), (0, 3)]
def getPosition1(l, val):
for row_nb, r in enumerate(l):
for col_nb in (x for x in xrange(len(r)) if r[x] == val):
yield row_nb, col_nb
最緊張,也可能是最慢的方法是:
>>> value = '1'
>>> l = [['1', '2', '3', '4'], ['3', '4', '5', '1']]
>>> m = []
>>> for i in range(len(l)):
... for j in range(len(l[i])):
... if l[i][j] == value:
... m.append((i,j))
...
>>> m
[(0, 0), (1, 3)]
這是另一種不使用生成器的直接方法。
def getPosition(lists,item):
positions = []
for i,li in enumerate(lists):
j = -1
try:
while True:
j = li.index(item,j+1)
positions.append((i,j))
except ValueError:
pass
return positions
l = [['1', '2', '4', '6'], ['7', '0', '1', '4']]
getPosition(l,'1') #returns [(0, 0), (1, 2)]
getPosition(l,'9') # returns []
l = [['1', '1', '1', '1'], ['7', '0', '1', '4']]
getPosition(l,'1') #returns [(0, 0), (0, 1), (0,2), (0,3), (1,2)]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.