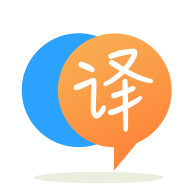
[英]How to get list of specific XML elements from XML document using C# ASP.Net?
[英]How to count XML tag with specific value to produce a summary C#; ASP.NET
我很難弄清楚如何計算具有特定值的XML標簽。 然后將其放入文本框。 到目前為止,我已經對xml文件中的數據進行反序列化,但是無法弄清楚如何獲得摘要。到目前為止,我得到的以下代碼是:
文件后面的代碼:index.aspx.cs
protected void summaryReport ()
{
XmlSerializer xmlSerializer = new XmlSerializer(typeof(Xml2CSharp.Registrations));
FileStream fileStream = null;
try
{
fileStream = new FileStream(fullFileName, FileMode.Open);
}
catch
{
txtSummary.Text = "Error: Xml file not Found.";
return;
}
Registrations registrationList = (Registrations)xmlSerializer.Deserialize(fileStream);
fileStream.Close();
txtSummary.Text = "There are " + ...numberofattendants... + " People attending the conference.";
}
XML文件:registrations.xml
<registrations>
<Registration>
<id>1</id>
<fullName>Keiran Bernal</fullName>
<emailAddress>k.bernal@gmail.com</emailAddress>
<registrationType>conference only</registrationType>
<attendingSocialEvent>yes</attendingSocialEvent>
</Registration>
<Registration>
<id>2</id>
<fullName>Cordelia Pierce</fullName>
<emailAddress>c.pierce@outlook.com</emailAddress>
<registrationType>conference and Dinner</registrationType>
<attendingSocialEvent>no</attendingSocialEvent>
</Registration>
<Registration>
<id>3</id>
<fullName>Zachery Guy</fullName>
<emailAddress>z.guy@yahoo.com</emailAddress>
<registrationType>conference only</registrationType>
<attendingSocialEvent>yes</attendingSocialEvent>
</Registration>
<Registration>
<id>4</id>
<fullName>Kiana Hawworth</fullName>
<emailAddress>k.hawworth@bigpond.com</emailAddress>
<registrationType>conference and Dinner</registrationType>
<attendingSocialEvent>no</attendingSocialEvent>
</Registration>
</registrations>
助手類:registrations.cs
namespace Xml2CSharp
{
[XmlRoot(ElementName = "Registration")]
public class Registration
{
[XmlElement(ElementName = "id")]
public string id { get; set; }
[XmlElement(ElementName = "fullName")]
public string fullName { get; set; }
[XmlElement(ElementName = "emailAddress")]
public string emailAddress { get; set; }
[XmlElement(ElementName = "registrationType")]
public string registrationType { get; set; }
[XmlElement(ElementName = "attendingSocialEvent")]
public string attendingSocialEvent { get; set; }
public Registration()
{
this.id = null;
this.fullName = null;
this.emailAddress = null;
this.registrationType = null;
this.attendingSocialEvent = null;
}
public Registration(string id, string fullName, string emailAddress, string registrationType, string attendingSocialEvent)
{
this.id = id;
this.fullName = fullName;
this.emailAddress = emailAddress;
this.registrationType = registrationType;
this.attendingSocialEvent = attendingSocialEvent;
}
}
[XmlRoot(ElementName = "registrations")]
public class Registrations
{
[XmlElement(ElementName = "Registration")]
public List<Registration> registrationList { get; set; }
public Registrations()
{
this.registrationList = new List<Registration>();
}
}
}
ASP.NET表單:index.cs
<form>
<div>
<asp:Label ID="lblSummary" runat="server" Text="Summary:" CssClass="lblsummary"></asp:Label><br />
<asp:TextBox ID="txtSummary" runat="server" TextMode="MultiLine" CssClass="txtsummary" Columns="50" Rows="5"></asp:TextBox>
</div>
</form>
我的目的是計算值為“是”的XML標簽的數量,然后將其匯總。 我相信我將不得不以某種方式將其放入變量中,但不確定如何以及如何只計算值為“是”的變量
將XML反序列化為Registrations
對象之后,可以使用Linq來計算注冊:
Registrations registrations = ....;
// check if registrations.registrationList is not null before doing this
int count = registrations.registrationList.Count(x =>
x.attendingSocialEvent.Equals("yes", StringComparison.OrdinalIgnoreCase));
或者,作為替代方法,將非序列化屬性添加到Registrations
類,如下所示:
[XmlRoot(ElementName = "registrations")]
public class Registrations
{
[XmlIgnore]
public int CountAttendances
{
get
{
if (registrationList == null)
{
return 0;
}
int count = registrationList.Count(x => x.attendingSocialEvent.Equals("yes", StringComparison.OrdinalIgnoreCase));
return count;
}
}
[XmlElement(ElementName = "Registration")]
public List<Registration> registrationList { get; set; }
public Registrations()
{
this.registrationList = new List<Registration>();
}
}
如果您只想計數,那么您實際上不需要反序列化為POCO ...
您可以按以下方式使用LINQ計算"yes"
回答者:
var xmlStr = @"<registrations>
<Registration>
<id>1</id>
<fullName>Keiran Bernal</fullName>
<emailAddress>k.bernal@gmail.com</emailAddress>
<registrationType>conference only</registrationType>
<attendingSocialEvent>yes</attendingSocialEvent>
</Registration>
<!-- SNIP -->
</registrations>";
var doc = XDocument.Parse(xmlStr);
var numYes = doc.Descendants("Registration")
.Count(r => string.Equals((string)r.Element("attendingSocialEvent"),
"yes",
StringComparison.OrdinalIgnoreCase))
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.