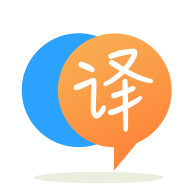
[英]Looking for most efficient way to calc all distance vectors between set of points in 3D
[英]Pythonic way to calc distance between list of points
我有一個未排序的點(2D)列表,我想計算它們之間的距離之和。 由於我的背景是 c++ 開發人員,所以我會這樣做:
import math
class Point:
def __init__(self, x,y):
self.x = x
self.y = y
def distance(P1, P2):
return math.sqrt((P2.x-P1.x)**2 + (P2.y-P1.y)**2)
points = [Point(rand(1), rand(1)) for i in range(10)]
#this part should be in a nicer way
pathLen = 0
for i in range(1,10):
pathLen += distance(points[i-1], points[i])
有沒有更pythonic的方法來替換for循環? 例如減少或類似的東西?
最好的祝福!
您可以使用sum
, zip
和itertools islice
的生成器islice
來避免重復數據:
from itertools import islice
paathLen = sum(distance(x, y) for x, y in zip(points, islice(points, 1, None)))
這里有實例
一些修復,作為C ++方法可能不是最好的:
import math
# you need this import here, python has no rand in the main namespace
from random import random
class Point:
def __init__(self, x,y):
self.x = x
self.y = y
# there's usually no need to encapsulate variables in Python
def distance(P1, P2):
# your distance formula was wrong
# you were adding positions on each axis instead of subtracting them
return math.sqrt((P1.x-P2.x)**2 + (P1.y-P2.y)**2)
points = [Point(random(), random()) for i in range(10)]
# use a sum over a list comprehension:
pathLen = sum([distance(points[i-1], points[i]) for i in range(10)])
@Robin Zigmond的zip
方法也是實現它的一種巧妙方法,盡管我可以在這里使用它並不是很明顯。
我遇到了類似的問題並拼湊了一個我認為效果很好的 numpy 解決方案。
即,如果將點列表轉換為 numpy 數組,則可以執行以下操作:
pts = np.asarray(points)
dist = np.sqrt(np.sum((pts[np.newaxis, :, :]-pts[:, np.newaxis, :])**2, axis=2))
dist 產生一個 nxn numpy 對稱數組,其中每個點到每個其他點之間的距離在對角線上方或下方給出。 對角線是每個點到自身的距離,因此僅為 0。
然后你可以使用:
path_leng = np.sum(dist[np.triu_indices(pts.shape[0], 1)].tolist())
收集 numpy 數組的上半部分並對它們求和以獲得路徑長度。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.