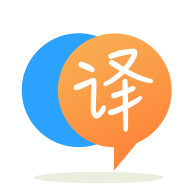
[英]Transferring specific data from one excel file to another using python
[英]Transferring data from excel to word in python 3
我正在嘗試編寫一個腳本,該腳本允許我從輸入的excel文件中讀取數據(保存為.csv格式,因為有人告訴我這樣做),然后將這些數據的選定部分寫入到word文檔中。
它是一個腳本,用於為參與者的餐食選擇創建個性化的送餐單(這些餐食已累積添加到輸入電子表格中)。
到目前為止,我已經創建了一個虛擬輸入電子表格,並保存了一個空白的虛擬輸出字文件(分別為dummy.csv和dummy.txt)。
到目前為止,我編寫的代碼將電子表格讀入終端,並進行了一些格式化以使其整潔。
import csv
f = open("dummy.csv")
csv_f = csv.reader(f)
for row in csv_f:
print('{:<15} {:<15} {:<20} {:<25}'.format(*row))
輸出看起來像這樣:(為方便起見,虛擬餐的選擇保持不變)
Participant ID Breakfasts Lunches/dinners Snacks
1111 Full english Risotto Granola
1111 Full english Risotto Granola
1111 Full english Risotto Granola
1111 Full english Risotto Granola
1111 Full english Risotto Granola
1111 Full english Risotto Granola
1111 Full english Risotto Granola
1111 Risotto Granola
1111 Risotto Granola
1111 Risotto Granola
1111 Risotto Granola
1111 Risotto Granola
1111 Risotto Granola
1111 Risotto Granola
2222 Avocado toast Bean chilli Apple
2222 Avocado toast Bean chilli Apple
2222 Avocado toast Bean chilli Apple
2222 Avocado toast Bean chilli Apple
2222 Avocado toast Bean chilli Apple
2222 Avocado toast Bean chilli Apple
2222 Avocado toast Bean chilli Apple
2222 Bean chilli Apple
2222 Bean chilli Apple
2222 Bean chilli Apple
2222 Bean chilli Apple
2222 Bean chilli Apple
2222 Bean chilli Apple
2222 Bean chilli Apple
我的下一個挑戰是以某種方式將此數據寫入到參與者1111的單詞文件中,另一個寫入參與者2222的單詞文件中,依此類推。 我不希望腳本將這些行中的確切數據寫入Word文件,而是如果輸入文件中的食物選擇不同,則這些行中的數據可以是什么。
最好在輸出交貨單上將餐點分為早餐,午餐/晚餐和零食。
我可以稍后整理字體,我只希望現在就餐選擇。 我也想說“ 7 x Full english”,而不是“ Full english,Full english,Full english等”。
感謝您的閱讀,我們將不勝感激!
基蘭
只是為了展示您可以使用pandas
榜樣:
import pandas as pd
df = pd.read_csv('whereverfilemayroam/filename')
Participant ID Breakfasts Lunches/dinners Snacks
0 1111 Full english Risotto Granola
1 1111 Full english Risotto Granola
2 1111 Full english Risotto Granola
3 1111 Full english Risotto Granola
4 1111 Full english Risotto Granola
5 1111 Full english Risotto Granola
6 1111 Full english Risotto Granola
7 1111 None Risotto Granola
8 1111 None Risotto Granola
9 1111 None Risotto Granola
10 1111 None Risotto Granola
11 1111 None Risotto Granola
12 1111 None Risotto Granola
13 1111 None Risotto Granola
14 2222 Avocado toast Bean chilli Apple
15 2222 Avocado toast Bean chilli Apple
16 2222 Avocado toast Bean chilli Apple
17 2222 Avocado toast Bean chilli Apple
18 2222 Avocado toast Bean chilli Apple
19 2222 Avocado toast Bean chilli Apple
20 2222 Avocado toast Bean chilli Apple
21 2222 None Bean chilli Apple
22 2222 None Bean chilli Apple
23 2222 None Bean chilli Apple
24 2222 None Bean chilli Apple
25 2222 None Bean chilli Apple
26 2222 None Bean chilli Apple
27 2222 None Bean chilli Apple
如果您願意,這就是您在pandas數據框中的文件,pandas中的標准容器。 現在,您可以使用它進行大量的統計工作...只需在文檔中進行一些挖掘
例子:
df.groupby(['Participant ID', 'Breakfasts']).Breakfasts.count()
Participant ID Breakfasts
1111 Full english 7
2222 Avocado toast 7
Name: Breakfasts, dtype: int64
df.groupby(['Participant ID', 'Lunches/dinners'])['Lunches/dinners'].count()
Participant ID Lunches/dinners
1111 Risotto 14
2222 Bean chilli 14
Name: Lunches/dinners, dtype: int64
當然,您可以按參與者ID分開:
oneoneoneone = df[df['Participant ID'] == 1111]
oneoneoneone
Participant ID Breakfasts Lunches/dinners Snacks
0 1111 Full english Risotto Granola
1 1111 Full english Risotto Granola
2 1111 Full english Risotto Granola
3 1111 Full english Risotto Granola
4 1111 Full english Risotto Granola
5 1111 Full english Risotto Granola
6 1111 Full english Risotto Granola
7 1111 None Risotto Granola
8 1111 None Risotto Granola
9 1111 None Risotto Granola
10 1111 None Risotto Granola
11 1111 None Risotto Granola
12 1111 None Risotto Granola
13 1111 None Risotto Granola
oneoneoneone.to_csv('target_file')
所以也許
twotwotwotwo.to_csv('another_target_file')
也可以遍歷各個組,然后對每個組始終應用相同的操作。
例如:
for name, group in df.groupby('Participant ID'):
print(name)
print(group.groupby('Breakfasts').Breakfasts.count().to_string())
print(group.groupby('Lunches/dinners')['Lunches/dinners'].count().to_string())
print(group.groupby('Snacks').Snacks.count().to_string(), '\n')
收益:
1111
Breakfasts
Full english 7
Lunches/dinners
Risotto 14
Snacks
Granola 14
2222
Breakfasts
Avocado toast 7
Lunches/dinners
Bean chilli 14
Snacks
Apple 14
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.