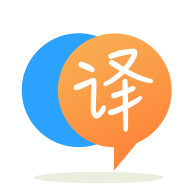
[英]Java netty client cannot send message to server, but telnet to sever ok
[英]I write an application with netty, send message from client to server, but server cannot receive the message
我用netty編寫了一個應用程序,客戶端使用channelActive方法將字符串消息發送到服務器,但是服務器未收到該消息。 我不知道原因。
服務器代碼:
public static void main(String[] args) throws InterruptedException {
NioEventLoopGroup boss = new NioEventLoopGroup();
NioEventLoopGroup worker = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(boss,worker);
bootstrap.channel(NioServerSocketChannel.class);
bootstrap.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new NettyServerHandler());
}
});
bootstrap.option(ChannelOption.SO_BACKLOG,1024);
bootstrap.childOption(ChannelOption.SO_KEEPALIVE,true);
ChannelFuture sync = bootstrap.bind(8080).sync();
System.out.println("server start");
sync.channel().closeFuture().sync();
System.out.println("server end");
} finally {
worker.shutdownGracefully();
boss.shutdownGracefully();
}
}
public class NettyServerHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
System.out.println("channelRead");
super.channelRead(ctx, msg);
System.out.println(((ByteBuf) msg).toString(Charset.defaultCharset()));
}
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
System.out.println("channelActive");
super.channelActive(ctx);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
System.out.println("exceptionCaught");
super.exceptionCaught(ctx, cause);
cause.printStackTrace();
ctx.close();
}
}
我在服務器處理程序的方法channelRead中從客戶端打印消息。
以下是客戶端代碼:
public static void main(String[] args) throws InterruptedException {
NioEventLoopGroup worker = new NioEventLoopGroup();
try {
Bootstrap bootstrap = new Bootstrap();
bootstrap.group(worker);
bootstrap.channel(NioSocketChannel.class);
bootstrap.handler(new ChannelInitializer<Channel>() {
@Override
protected void initChannel(Channel ch) throws Exception {
ch.pipeline().addLast(new NettyClientHandler());
}
});
ChannelFuture future = bootstrap.connect("localhost", 8080).sync();
future.channel().closeFuture().sync();
}finally {
worker.shutdownGracefully();
}
}
public class NettyClientHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
System.out.println("channelActive");
super.channelActive(ctx);
ctx.writeAndFlush("message from client");
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
System.out.println("channelRead");
super.channelRead(ctx, msg);
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
System.out.println("exceptionCaught");
super.exceptionCaught(ctx, cause);
cause.printStackTrace();
ctx.close();
}
}
我從通道處於活動狀態的客戶端發送了消息,但服務器未收到消息,字符串“來自客戶端的消息”
那是因為您在調用writeAndFlush(...)
時使用了String
。 返回的ChannelFuture
應該告訴您不支持的消息類型。 如果要使用String
,則需要將StringEncoder
放在ChannelPipeline
,否則僅需要編寫ByteBuf
實例。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.