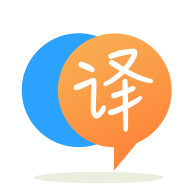
[英]C# Entity Framework - Handling destructive autogen DB scripts with model first design
[英]C# console app with DB first Entity Framework and AutoMapper, cannot convert Model to DbModel
我試圖讓一個基本的控制台應用程序工作,以便我可以使用 DB First Entity Framework 和 AutoMapper 將用戶輸入的數據保存到現有數據庫中,但是當我嘗試將本地模型保存回數據庫時,我無法編譯,因為它無法轉換本地模型到數據庫模型。
EF 生成了以下類:
//------------------------------------------------------------------------------
// <auto-generated>
// This code was generated from a template.
//
// Manual changes to this file may cause unexpected behavior in your application.
// Manual changes to this file will be overwritten if the code is regenerated.
// </auto-generated>
//------------------------------------------------------------------------------
namespace DbModels
{
using System;
using System.Collections.Generic;
public partial class contact
{
[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")]
public contact()
{
this.address = new HashSet<address>();
}
public System.Guid id { get; set; }
public string full_name { get; set; }
public string email { get; set; }
public string mobile_phone { get; set; }
public System.DateTime created_timestamp { get; set; }
public System.DateTime modified_time_stamp { get; set; }
[System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly")]
public virtual ICollection<address> address { get; set; }
}
}
然后我有一個本地contact
模型:
using System;
namespace AppModels
{
public class contact
{
public contact()
{
id = new Guid();
created_timestamp = DateTime.Now;
modified_time_stamp = DateTime.Now;
}
public Guid id { get; }
public string full_name { get; set; }
public string email { get; set; }
public string mobile_phone { get; set; }
public DateTime created_timestamp { get; }
public DateTime modified_time_stamp { get; }
}
}
在我的控制台應用程序中,我使用 AutoMapper 在模型之間創建映射,獲取一些用戶輸入,並嘗試將該輸入保存到數據庫中:
static void Main(string[] args)
{
var config = new MapperConfiguration(cfg => {
cfg.CreateMap<DbModels.contact, AppModels.contact>().ReverseMap();
});
IMapper mapper = config.CreateMapper();
var source = new DbModels.contact();
var dest = mapper.Map<DbModels.contact, AppModels.contact>(source);
AppModels.contact ContactDetails = new AppModels.contact();
Console.WriteLine("Enter your name:");
ContactDetails.full_name = Console.ReadLine();
Console.WriteLine("Enter your email:");
ContactDetails.email = Console.ReadLine();
Console.WriteLine("Enter your phone number:");
ContactDetails.mobile_phone = Console.ReadLine();
using (var dbcontext = new myDbContext())
{
dbcontext.contact.Add(mapper.Map<DbModels.contact,AppModels.contact>(ContactDetails)); // Argument 1: cannot convert from 'AppModels.contact' to 'DbModels.contact'
dbcontext.SaveChanges();
}
}
dbcontext.contact.Add(ContactDetails);
行在編譯Argument 1: cannot convert from 'AppModels.contact' to 'DbModels.contact'
引發錯誤Argument 1: cannot convert from 'AppModels.contact' to 'DbModels.contact'
我在 Web 應用程序中使用了幾乎相同的 automapper/EF 代碼並且一切正常,似乎 AutoMapper 沒有告訴EntityFramework 有一個它可以使用的模型映射。
您的異常聲明“參數 1:無法從‘AppModels.contact’轉換為‘DbModels.contact’”。
不過,您的映射是從 DbModel 版本到 AppModel 版本。 不確定您使用的是哪個版本的 AM,但有一個 ReverseMap 函數,您可以將其鏈接到地圖調用的末尾並使其雙向執行。
這是添加到 AM 的提交。 https://github.com/AutoMapper/AutoMapper/commit/bff6e2aa49af3e7b50f527376da48924efa7d81e
對於其他人的未來參考,這里是 ReverseMap 方法的文檔: http ://docs.automapper.org/en/stable/Reverse-Mapping-and-Unflattening.html
更新:我剛剛注意到您使用的是 Map() 而不是 CreateMap()。 AM 是一個 2 步過程...1 創建地圖和 1 進行映射。 您只是在執行初始化的第二步,但遺漏了第一部分。 我還更新了下面的示例,因為我剛剛逐字復制了您的代碼並添加了 Reverse 調用。
在(初始化方法)中更改此行:
var dest = mapper.Map<DbModels.contact, AppModels.contact>(source);
對此:
var dest = mapper.CreateMap<DbModels.contact, AppModels.contact>(source).ReverseMap();
然后在您的保存中更改它:
using (var dbcontext = new myDbContext())
{
dbcontext.contact.Add(ContactDetails); // Argument 1: cannot convert from 'AppModels.contact' to 'DbModels.contact'
dbcontext.SaveChanges();
}
對此:
using (var dbcontext = new myDbContext())
{
dbcontext.contact.Add(AM.Map<Src, Dest>(ContactDetails)); // This is pseudo...you have to have the mapper in scope as was pointed out in the comments of your ? and I don't think the Src type is required in most versions of AM.
dbcontext.SaveChanges();
}
這是它的用法示例:
var dest = mapper.CreateMap<DbModels.contact, AppModels.contact>(source).ReverseMap();
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.