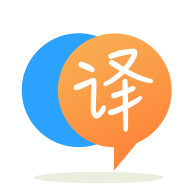
[英]Reason: CORS header ‘Access-Control-Allow-Origin’ missing
[英]Multiple CORS header ‘Access-Control-Allow-Origin’ not allowed / CORS header ‘Access-Control-Allow-Origin’ missing)
當我從 Angular 7 應用程序(托管在http://localhost:4200 上)向我的 Spring-Boot 應用程序(托管在https://localhost:8924 上)從 Firefox 發送 HTTP 請求時,我遇到了 CORS 標頭問題。
我的 Spring-Boot 應用程序中有一個 CORS 過濾器,它已添加到我的請求中:
@Component
public class CorsFilter extends OncePerRequestFilter {
@Override
protected void doFilterInternal(final HttpServletRequest request, final HttpServletResponse response,
final FilterChain filterChain) throws ServletException, IOException {
response.addHeader("Access-Control-Allow-Origin", "*");
response.addHeader("Access-Control-Allow-Methods", "GET, POST, DELETE, PUT, PATCH, HEAD");
response.setHeader("Access-Control-Allow-Headers", "X-Requested-With, Authorization, Content-Type");
response.addIntHeader("Access-Control-Max-Age", 3600);
if ("OPTIONS".equalsIgnoreCase(request.getMethod()))
{
response.setStatus(HttpServletResponse.SC_OK);
}
else
{
filterChain.doFilter(request, response);
}
}
}
@Override
protected void configure(HttpSecurity http) throws Exception
{
http.addFilterBefore(corsFilter(), SessionManagementFilter.class);
http.csrf().disable().
authorizeRequests()
.antMatchers("/api/auth/**","/api/MentorCalendar","/api/user/role/**").permitAll()
.anyRequest().authenticated()
.and()
.exceptionHandling().authenticationEntryPoint(unauthorizedHandler).and()
.sessionManagement().sessionCreationPolicy(SessionCreationPolicy.STATELESS);
http.addFilterBefore(authenticationJwtTokenFilter(), UsernamePasswordAuthenticationFilter.class);
}
發送請求時,Firefox 控制台返回此錯誤消息:
跨域請求被阻止:同源策略不允許讀取位於http://localhost:8924/api/auth/signin的遠程資源。 (原因:不允許多個 CORS 標頭“Access-Control-Allow-Origin”)。
注釋掉 Access-Control-Allow-Origin:
@Component
public class CorsFilter extends OncePerRequestFilter {
@Override
protected void doFilterInternal(final HttpServletRequest request, final HttpServletResponse response,
final FilterChain filterChain) throws ServletException, IOException {
// response.addHeader("Access-Control-Allow-Origin", "*");
response.addHeader("Access-Control-Allow-Methods", "GET, POST, DELETE, PUT, PATCH, HEAD");
response.setHeader("Access-Control-Allow-Headers", "X-Requested-With, Authorization, Content-Type");
response.addIntHeader("Access-Control-Max-Age", 3600);
if ("OPTIONS".equalsIgnoreCase(request.getMethod()))
{
response.setStatus(HttpServletResponse.SC_OK);
}
else
{
filterChain.doFilter(request, response);
}
}
}
返回此錯誤消息:
跨域請求被阻止:同源策略不允許讀取位於http://localhost:8924/api/auth/signin的遠程資源。 (原因:缺少 CORS 標頭“Access-Control-Allow-Origin”)。
src/app/home/login.component.ts 提取
export class LoginComponent {
constructor(private LoginService: LoginService, private router: Router) {}
login(inputUsername, inputPassword){
this.LoginService.login({username: inputUsername, password: inputPassword})
.subscribe(data => {
localStorage.setItem("jwtToken", data.accessToken);
src/app/home/login.service.ts
const httpOptions = {
headers: new HttpHeaders({
'Content-Type': 'application/json'
})
};
@Injectable()
export class LoginService {
loginUrl = "http://localhost:8924/api/auth/signin";
constructor( private http: HttpClient ) {}
login(loginCreds: any): Observable<any> {
return this.http.post<any>(this.loginUrl, loginCreds, httpOptions);
}
}
response.addHeader("Access-Control-Allow-Origin", "*"); 行在包含時設置多個標題,但在刪除時沒有設置?
您是否嘗試在 springboot 控制器中啟用@CrossOrigin() 。 下面是基本片段。
@CrossOrigin(origins = "http://localhost:9000")
@GetMapping("/greeting")
public Greeting greeting(@RequestParam(required=false, defaultValue="World") String name) {
System.out.println("==== in greeting ====");
return new Greeting(counter.incrementAndGet(), String.format(template, name));
}
有關更多信息,請訪問啟用 CORS
我有同樣的問題。 通過上面的解決方法解決了。 您在使用 Internet Explorer 時一定不是 CORS 問題。
這為我解決了這個問題:
if(!response.containsHeader("Access-Control-Allow-Origin"))
{
response.addHeader("Access-Control-Allow-Origin", "*");
}
不是讓它工作的理想方式
在我的情況下,當我在 nginx conf 文件和 Django 中間件中設置 CORS 支持時會發生這種情況。
Nginx部分:
if ($request_method = 'OPTIONS') {
add_header 'Access-Control-Allow-Origin' '*';
add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS';
add_header 'Access-Control-Allow-Headers' 'DNT,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Range';
add_header 'Access-Control-Max-Age' 1728000;
add_header 'Content-Type' 'text/plain; charset=utf-8';
add_header 'Content-Length' 0;
return 204;
}
if ($request_method = 'POST') {
add_header 'Access-Control-Allow-Origin' '*';
add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS';
add_header 'Access-Control-Allow-Headers' 'DNT,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Range';
add_header 'Access-Control-Expose-Headers' 'Content-Length,Content-Range';
}
if ($request_method = 'GET') {
add_header 'Access-Control-Allow-Origin' '*';
add_header 'Access-Control-Allow-Methods' 'GET, POST, OPTIONS';
add_header 'Access-Control-Allow-Headers' 'DNT,User-Agent,X-Requested-With,If-Modified-Since,Cache-Control,Content-Type,Range';
add_header 'Access-Control-Expose-Headers' 'Content-Length,Content-Range';
}
姜戈部分:
MIDDLEWARE = (
'corsheaders.middleware.CorsMiddleware',
解決方案非常簡單 - 我只是刪除了 nginx 中的 CORS 部分並且一切正常
location / {
uwsgi_pass django_upstream;
include uwsgi_params;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.