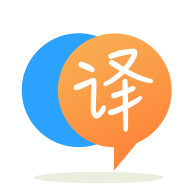
[英]How can I target the response from an ajax to a specific div that was clicked
[英]How can I insert my ajax response values to a specific div element?
我有一個 ajax 調用,它檢索數據並將其插入到所需的 div 框中。 但是,我在success方法中只能使用一個參數來獲取數據,不能使用多個參數。 一個超級糟糕/便宜的修復是多次 ajax 調用,但我只知道必須有一個比創建不同的調用/腳本來獲取我的數據更簡單、更不臟的解決方案。 如上所述,我也嘗試過使用多個參數,希望可能會奏效。
我的 ajax 調用如下所示:
function retrieveQuestion() {
$.ajax({
url: 'getSpecificPost.php',
type: 'POST',
success: function(response) {
console.log(response);
}
});
}
我的 php 腳本看起來像這樣(不是全部,考慮到腳本有效,我的主要問題是如何將某些數據附加到某些 div):
$sqli = 'SELECT * FROM posts WHERE title = ?';
$stmt = mysqli_stmt_init($conn);
if (!mysqli_stmt_prepare($stmt, $sqli)) {
echo 'Error no stmt prepared';
exit();
} else {
mysqli_stmt_bind_param($stmt, 's', $title);
mysqli_stmt_execute($stmt);
$result = mysqli_stmt_get_result($stmt);
$resultCheck = mysqli_num_rows($result);
if ($resultCheck > 0) {
if ($row = mysqli_fetch_assoc($result)) {
echo $row['title'];
echo $row['name'];
echo $row['id'];
}
}
所以讓我們說,我有 3 個已經制作好的 div 框,所以它們不會動態創建。
我想將 $row['title'] 附加到帶有 id 標題的 div 框,將 $row['name'] 附加到帶有 id 名稱的 div 框,將帶有 $row['id'] 的附加到一個帶有 id 的 div 框,id。
由於 Ajax 將所有數據一起響應。 您有兩個選擇要做... 1. 在服務器 php 頁面中構建您的 html div 並直接插入您要更新的所需 div。 2. 你必須通過復雜的 JavaScript 和 json 數據......以 json 格式接收數據,然后手動放置在所需的位置。 但我建議第一個選項在服務器中使用各自的 id 創建您的 div,並在成功事件中直接將其插入 div
您是否嘗試過返回包含所有數據的 JSON? 像這樣的東西:
echo '{ title:', $row['title'], ', name: ', $row['name'], ', id: ', $row['id'], ' }'
如果您不想自己構建 JSON,也可以創建一個對象並使用json_encode
。
然后在您的 JavaScript 中,您可以將成功函數更改為:
success: function(response) {
response = JSON.parse(response); // converts string to Object
for(var property in response) { // loops for title, name and id
// Sets the text of the element with id: "title", "name" or "id"
document.querySelector("#" + property).textContent = result[property];
}
}
JavaScript 中的代碼將 JSON 從字符串解析為 Ob
JSON
JSON 是一種人類可讀的文本格式,通常用於在服務器和客戶端之間傳輸數據。
PHP 中的json_enocde()函數將數組轉換為 json 字符串,javascript 中的JSON.parse()函數將 json 字符串轉換為對象數組,其中填充了適當的值。
json_encode() 的 Javascript 等價物是JSON.stringify() ,而 JSON.parse() 的 PHP 等價物是json_decode() 。
PHP
$sqli = 'SELECT * FROM posts WHERE title = ?';
$stmt = mysqli_stmt_init($conn);
if (!mysqli_stmt_prepare($stmt, $sqli)) {
echo 'Error no stmt prepared';
exit();
} else {
mysqli_stmt_bind_param($stmt, 's', $title);
mysqli_stmt_execute($stmt);
$result = mysqli_stmt_get_result($stmt);
$resultCheck = mysqli_num_rows($result);
if ($resultCheck > 0) {
$json_response = [];
if ($row = mysqli_fetch_assoc($result)) {
$json_response[] = $row;
}
//Echo data as JSON
echo json_encode($json_response);
}
Javascript
function retrieveQuestion() {
$.ajax({
url: 'getSpecificPost.php',
type: 'POST',
success: function(response) {
response = JSON.parse(response);
$("#title").text(response[0].title);
$("#name").text(response[0].name);
$("#id").text(response[0].id);
}
});
}
嘗試在 php 中將它作為一個字符串發送並使用逗號然后在你的 ajax 中使用這個:
$.ajax({
url: 'getSpecificPost.php',
type: 'POST',
success: function(response) {
var data = response.split(",");
$('#title').val(data[0]);
$('#name').val(data[1]);
$('#id').val(data[2]);
}
});
}
嘗試在 ajax 成功時訪問您的 DOM html。 看這個例子:
編輯你的 php 並設置“return $row”
//...
if ($resultCheck > 0) {
if ($row = mysqli_fetch_assoc($result)) {
return $row
}
}
//here is your Succes ajas response //success: function(response) { var response=[];//this line is not necesari , i write because i dont hae response; response['name'] ='testName'; response['title'] ='testtitle'; response['id'] ='testid'; $('#title').html(response['title']); $('#name').html(response['name']); $('#id').html(response['id']); // }
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <div id="title"></div> <div id="name"></div> <div id="id"></div>
如果我理解正確,您希望使用從服務器獲得的響應動態更新靜態頁面。
HTML
並向其添加值由於您使用的是 jquery,這是一個非常好的DOM
操作庫,因此您可以按原樣使用HTML
。 並在收到回復時更新您的 DOM
-- 注意 - 我只是想復制您的問題並向您展示可能的方法。
嘗試在輸入框中輸入任何產品名稱
//Suppose your response contains this form of data let products = [ {id : '1', product_name : 'amazon', img : 'something'}, {id : '2', product_name : 'snapdeal', img : 'something'}, {id : '3', product_name : 'google', img : 'something'} ] let input = $('#search') input.keyup(function(){ let showingContacts; if($(this).val()){ let match = new RegExp($(this).val()) showingProducts = products.filter(product=> match.test(product.product_name)) }else showingProducts = products updateDom(showingProducts) }) const updateDom = products =>{ $('.contents').html('') products.forEach(product =>{ $('.contents').append(`<div class="card"> <h1 id="title">${product.product_name}</h1> </div>`) }) } updateDom(products)
.contents {display: flex; flex-flow: wrap;} .card { background: wheat; padding: 1.875rem; margin-right: 10px; margin-bottom: 10px; }
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <input type="text" id="search"> <div class = "contents"> </div>
您可以通過創建一個包含所有行的數組並將其編碼為 JSON 從您的服務器返回 JSON
$sqli = 'SELECT * FROM posts WHERE title = ?';
$stmt = mysqli_stmt_init($conn);
if (!mysqli_stmt_prepare($stmt, $sqli)) {
echo 'Error no stmt prepared';
exit();
} else {
mysqli_stmt_bind_param($stmt, 's', $title);
mysqli_stmt_execute($stmt);
$result = mysqli_stmt_get_result($stmt);
$resultCheck = mysqli_num_rows($result);
if ($resultCheck > 0) {
$response = [];
if ($row = mysqli_fetch_assoc($result)) {
$response[] = $row;
}
//Return the array as json
print json_encode($response);
}
}
然后當你得到響應時,你可以在你的 ajax 調用的成功方法中循環響應
function retrieveQuestion() {
$.ajax({
url: 'getSpecificPost.php',
type: 'POST',
success: function(response) {
$("#title").html("<ul>");
$("#name").html("<ul>");
$("#id").html("<ul>");
for (var val in response) {
if (response.hasOwnProperty(val)) {
$("#title").append("<li>"+response[val].title+"</li>");
$("#name").append("<li>"+response[val].name+"</li>");
$("#id").append("<li>"+response[val].id+"</li>");
}
}
$("#title").append("</ul>");
$("#name").append("</ul>");
$("#id").append("</ul>");
}
});
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.