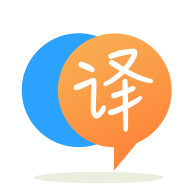
[英]C# Intersect Two Dictionaries and Store The Key and The Two Values in a List
[英]c# read lines and store values in dictionaries
我想讀取一個csv文件並以正確的方式將值存儲在詞典中。
using (var reader = new StreamReader(@"CSV_testdaten.csv"))
{
while (!reader.EndOfStream)
{
string new_line;
while ((new_line = reader.ReadLine()) != null)
{
var values = new_line.Split(",");
g.add_vertex(values[0], new Dictionary<string, int>() { { values[1], Int32.Parse(values[2]) } });
}
}
}
add_vertex函數如下所示:
Dictionary<string, Dictionary<string, int>> vertices = new Dictionary<string, Dictionary<string, int>>();
public void add_vertex(string name, Dictionary<string, int> edges)
{
vertices[name] = edges;
}
csv文件如下所示:
有多個具有相同值[0]的行(例如,值[0]為“0”)並且不應覆蓋現有字典,而應將其添加到已存在且值為[0] = 0的字典中。 :
g.add_vertex("0", new Dictionary<string, int>() { { "1", 731 } ,
{ "2", 1623 } , { "3" , 1813 } , { "4" , 2286 } , { "5" , 2358 } ,
{ "6" , 1 } , ... });
我想將具有相同ID的所有值(在csv文件的第一列中)添加到具有此ID的一個字典中。 但我不知道該怎么做。 有人可以幫忙嗎?
當我們有復雜的數據並且我們想要查詢它們時, Linq會非常有幫助:
var records = File
.ReadLines(@"CSV_testdaten.csv")
.Where(line => !string.IsNullOrWhiteSpace(line)) // to be on the safe side
.Select(line => line.Split(','))
.Select(items => new {
vertex = items[0],
key = items[1],
value = int.Parse(items[2])
})
.GroupBy(item => item.vertex)
.Select(chunk => new {
vertex = chunk.Key,
dict = chunk.ToDictionary(item => item.key, item => item.value)
});
foreach (var record in records)
g.add_vertex(record.vertex, record.dict);
這對你有用嗎?
vertices =
File
.ReadLines(@"CSV_testdaten.csv")
.Select(x => x.Split(','))
.Select(x => new { vertex = x[0], name = x[1], value = int.Parse(x[2]) })
.GroupBy(x => x.vertex)
.ToDictionary(x => x.Key, x => x.ToDictionary(y => y.name, y => y.value));
您可以將代碼分為兩部分。 首先會讀取csv行:
public static IEnumerable<(string, string, string)> ReadCsvLines()
{
using (var reader = new StreamReader(@"CSV_testdaten.csv"))
{
while (!reader.EndOfStream)
{
string newLine;
while ((newLine = reader.ReadLine()) != null)
{
var values = newLine.Split(',');
yield return (values[0], values[1], values[2]);
}
}
}
}
第二個將這些行添加到字典:
var result = ReadCsvLines()
.ToArray()
.GroupBy(x => x.Item1)
.ToDictionary(x => x.Key, x => x.ToDictionary(t => t.Item2, t => int.Parse(t.Item3)));
輸入result
將是:
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.