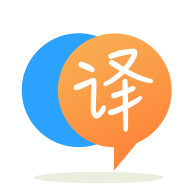
[英]How to update a ViewModel class variable when ApplicationData settings have changed?
[英]How to get View to update when the ViewModel gets changed
我正在構建WPF應用程序來管理存儲在SharePoint列表中的學生數據庫。 我是使用MVVM的新手,並且已經完成了一些教程。 我已經成功地創建了視圖和模型並將其綁定到數據網格控件。 我想做的是根據組合框的輸出更新視圖中的數據。
這是我的模型:
using System.ComponentModel;
namespace StudentManagement.Model
{
public class Student : INotifyPropertyChanged
{
private string _Title;
private string _FullName;
public string Title
{
get { return _Title; }
set
{
if (_Title != value)
{
_Title = value;
RaisePropertyChanged("Title");
}
}
}
public string FullName
{
get { return _FullName; }
set
{
if (_FullName != value)
{
_FullName = value;
RaisePropertyChanged("FullName");
}
}
}
public event PropertyChangedEventHandler PropertyChanged;
private void RaisePropertyChanged(string property)
{
if (PropertyChanged != null)
{
PropertyChanged(this, new PropertyChangedEventArgs(property));
}
}
}
}
這是視圖模型:
using System.Collections.ObjectModel;
using StudentManagement.Model;
using SP = Microsoft.SharePoint.Client;
using StudentManagement.publicClasses;
namespace StudentManagement.ViewModel
{
public class StudentViewModel
{
public ObservableCollection<Student> Students { get; set; }
public void LoadStudents(string query)
{
ObservableCollection<Student> _students = new
ObservableCollection<Student>();
SP.ClientContext ctx = clientContext._clientContext;
SP.CamlQuery qry = new SP.CamlQuery();
qry.ViewXml = query;
SP.ListItemCollection splStudents =
ctx.Web.Lists.GetByTitle("Students").GetItems(qry);
ctx.Load(splStudents);
ctx.ExecuteQuery();
foreach (SP.ListItem s in splStudents)
{
_students.Add(new Student { Title = (string)s["Title"], FullName = (string)s["FullName"] });
}
Students = _students;
}
}
}
這是我的XAML
<UserControl x:Class="StudentManagement.Views.StudentsView" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" mc:Ignorable="d" xmlns:local="clr-namespace:StudentManagement.Views" > <Grid> <StackPanel HorizontalAlignment="Left" Width="200" > <TextBox Name="txtSearch" AcceptsReturn="True" ></TextBox> <ComboBox Name="cmbStatus" SelectionChanged="cmbStatus_SelectionChanged" SelectedIndex="0"> <ComboBoxItem>Active</ComboBoxItem> <ComboBoxItem>Inquiring</ComboBoxItem> <ComboBoxItem>Inactive</ComboBoxItem> <ComboBoxItem>Monitoring</ComboBoxItem> </ComboBox> <DataGrid Name="dgStudentList" ItemsSource="{Binding Path=Students}" AutoGenerateColumns="False"> <DataGrid.Columns> <DataGridTextColumn Header="Name" Binding="{Binding Title, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}" Width="100"/> <DataGridTextColumn Header="Parent" Binding="{Binding FullName, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}" Width="100" /> </DataGrid.Columns> </DataGrid> </StackPanel> </Grid> </UserControl>
...以及該視圖后面的代碼:
using System.Windows.Controls;
using StudentManagement.ViewModel;
namespace StudentManagement.Views
{
/// <summary>
/// Interaction logic for StudentsView.xaml
/// </summary>
public partial class StudentsView : UserControl
{
private StudentViewModel _viewModel = new
StudentViewModel();
public StudentsView()
{
InitializeComponent();
DataContext = _viewModel;
}
private void cmbStatus_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
string combotext = ((sender as ComboBox).SelectedItem as ComboBoxItem).Content as string;
string qry = @"<View>
<Query>
<Where><Eq><FieldRef Name='Current_x0020_Status' /><Value Type='Choice'>" + combotext + @"</Value></Eq></Where>
</Query>
</View>";
_viewModel.LoadStudents(qry);
}
}
}
從目前的情況來看,學生可以很好地加載到數據網格中。 當事件cmbStatus_SelectionChanged觸發時,我已經完成了測試,並且可以看到LoadStudents函數觸發並返回正確數量的條目,但是在datagrid上沒有任何更新。
我敢肯定這是一個菜鳥錯誤,我想念一些基本的東西,但是我正在努力,我將不勝感激。
由於StudentViewModel.LoadStudents()
改變的價值Students
屬性,視圖模型需要通知認為,這改變了。 您可以通過讓StudentViewModel
實現INotifyPropertyChanged
來做到這一點(就像Student
一樣)。 DataGrid
將預訂PropertyChanged
事件,並在觸發該事件時更新其內容。
如果組合框的選擇發生更改,則每次都在初始化學生集合。
ObservableCollection<Student> _students = new ObservableCollection<Student>();
您不應在ViewModel中使用綁定集合來執行此操作。 您可以像這樣清除集合並添加新項目。
public class StudentViewModel
{
public ObservableCollection<Student> Students { get; set; } = new ObservableCollection<Student>();
public void LoadStudents(string query)
{
Students.Clear();
SP.ClientContext ctx = clientContext._clientContext;
SP.CamlQuery qry = new SP.CamlQuery();
qry.ViewXml = query;
SP.ListItemCollection splStudents = ctx.Web.Lists.GetByTitle("Students").GetItems(qry);
ctx.Load(splStudents);
ctx.ExecuteQuery();
foreach (SP.ListItem s in splStudents)
{
Students.Add(new Student { Title = (string)s["Title"], FullName = (string)s["FullName"] });
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.