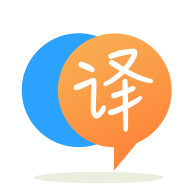
[英]Should I use Thread.Sleep between a socket reception and another?
[英]Should I use Thread.Sleep() to check if a process is still running?
如標題所述,我目前正在制作WPF應用程序,我需要檢測應用程序是否正在運行並在關閉時執行某些操作。 我想到的方法是運行一個單獨的線程,每兩秒鍾檢查一次進程是否仍在運行,如下所示:
while(Process.GetProcessesByName(processName).Length != 0) {
Thread.Sleep(2000);
}
//Do something
這將是一個好的解決方案,還有其他方法嗎?
謝謝
這將是一個好的解決方案嗎?
不,因為這幾乎浪費了整個線程。
最好在WPF應用程序中最好使用計時器,最好是DispatcherTimer:
var timer = new DispatcherTimer { Interval = TimeSpan.FromSeconds(2) };
timer.Tick += (s, e) =>
{
if (Process.GetProcessesByName(processName).Length > 0)
{
// ...
}
};
timer.Start();
如果要在UI線程上執行冗長的操作,則可以使用等待Task
的異步Tick
事件處理程序(它將在后台在線程池線程上運行):
var timer = new DispatcherTimer { Interval = TimeSpan.FromSeconds(2) };
timer.Tick += async (s, e) =>
{
if (Process.GetProcessesByName(processName).Length > 0)
{
await Task.Run(() =>
{
// lengthy operation here which runs on a thread pool thread
});
// udate UI here
}
};
timer.Start();
由於您已經在處理流程,因此建議您直接使用它來確定它是否已退出。 您可以為代碼使用Exited事件處理程序。 因此,例如:
foreach (var process in Process.GetProcessesByName(processName))
{
process.Exited += new EventHandler(DoSomething);
}
…
public void DoSomething(object sender, System.EventArgs e)
{
// do something
}
當具有該名稱的進程結束時,這將調用DoSomething
。
您可以使用每隔x秒執行一次檢查的System.Timers.Timer
:
public sealed partial class Window1 : Window, IDisposable
{
private readonly System.Timers.Timer _timer = new System.Timers.Timer(TimeSpan.FromSeconds(2).TotalMilliseconds);
public Window1()
{
InitializeComponent();
_timer.Elapsed += _timer_Elapsed;
_timer.Start();
}
private void _timer_Elapsed(object sender, System.Timers.ElapsedEventArgs e)
{
if (Process.GetProcessesByName("processName...").Length == 0)
{
_timer.Stop();
_timer.Dispose();
//do something...
}
}
public void Dispose()
{
_timer.Dispose();
}
}
與DispatcherTimer
的Tick
事件不同, Timer
的Elapsed
事件始終在線程池線程中排隊等待執行。
從文檔 :
如果在WPF應用程序中使用
System.Timers.Timer
,則值得注意的是System.Timers.Timer
在與用戶界面(UI)線程不同的線程上運行...使用DispatcherTimer
原因,而不是System.Timers.Timer
是該DispatcherTimer
運行同一個線程中的Dispatcher
和DispatcherPriority
可以在設置DispatcherTimer
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.