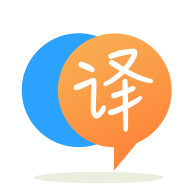
[英]Best way in Python to determine all possible intersections in a matrix?
[英]What is the best way to find ALL possible intersections of multiple sets?
假設我有以下 4 套
Set1 = {1,2,3,4,5}
Set2 = {4,5,6,7}
Set3 = {6,7,8,9,10}
Set4 = {1,8,9,15}
我想找到任何這些集合之間的所有可能的交集,例如:
Set1 and Set4: 1
Set1 and Set2: 4,5
Set2 and Set3: 6,7
Set3 and Set4: 8,9
就python而言,最好的方法是什么? 謝謝!
從這里:
# Python3 program for intersection() function
set1 = {2, 4, 5, 6}
set2 = {4, 6, 7, 8}
set3 = {4,6,8}
# union of two sets
print("set1 intersection set2 : ", set1.intersection(set2))
# union of three sets
print("set1 intersection set2 intersection set3 :", set1.intersection(set2,set3))
從文檔中:
交叉點(*其他)
設置 & 其他 & ...
返回一個新的集合,其中包含集合和所有其他元素共有的元素。
您需要找到 2 組組合(從所需的輸出中減去)。 這可以使用[Python 3.Docs]: itertools來實現。 組合( iterable, r ) 。 對於每個組合,都應該進行 2 組之間的交集。
為了執行上述操作,(輸入)集被“分組”在列表中(可迭代)。
還指出[Python 3.docs]: class set ( [iterable] ) 。
代碼.py :
#!/usr/bin/env python3
import sys
import itertools
def main():
set1 = {1, 2, 3, 4, 5}
set2 = {4, 5, 6, 7}
set3 = {6, 7, 8, 9, 10}
set4 = {1, 8, 9, 15}
sets = [set1, set2, set3, set4]
for index_set_pair in itertools.combinations(enumerate(sets, start=1), 2):
(index_first, set_first), (index_second, set_second) = index_set_pair
intersection = set_first.intersection(set_second)
if intersection:
print("Set{:d} and Set{:d} = {:}".format(index_first, index_second, intersection))
if __name__ == "__main__":
print("Python {:s} on {:s}\n".format(sys.version, sys.platform))
main()
print("\nDone.")
請注意[Python 3.Docs]: Built-in Functions - enumerate ( iterable, start=0 )僅用於打印目的( Set 1 , Set 2 , ... 在輸出中)。
輸出:
[cfati@CFATI-5510-0:e:\\Work\\Dev\\StackOverflow\\q056551261]> "e:\\Work\\Dev\\VEnvs\\py_064_03.07.03_test0\\Scripts\\python.exe" code.py Python 3.7.3 (v3.7.3:ef4ec6ed12, Mar 25 2019, 22:22:05) [MSC v.1916 64 bit (AMD64)] on win32 Set1 and Set2 = {4, 5} Set1 and Set4 = {1} Set2 and Set3 = {6, 7} Set3 and Set4 = {8, 9} Done.
如果您只是在尋找兩個集合的交集,您可以簡單地進行嵌套 for 循環:
Set1 = {1,2,3,4,5}
Set2 = {4,5,6,7}
Set3 = {6,7,8,9,10}
Set4 = {1,8,9,15}
sets = [Set1,Set2,Set3,Set4]
for i,s1 in enumerate(sets[:-1]):
for j,s2 in enumerate(sets[i+1:]):
print(f"Set{i+1} and Set{i+j+2} = {s1&s2}")
# Set1 and Set2 = {4, 5}
# Set1 and Set3 = set()
# Set1 and Set4 = {1}
# Set2 and Set3 = {6, 7}
# Set2 and Set4 = set()
# Set3 and Set4 = {8, 9}
如果您正在尋找任意數量的這些集合的交集,那么您可以使用 itertools 中的組合()來生成索引的冪集並為每個組合執行交集:
from itertools import combinations
for comboSize in range(2,len(sets)):
for combo in combinations(range(len(sets)),comboSize):
intersection = sets[combo[0]]
for i in combo[1:]: intersection = intersection & sets[i]
print(" and ".join(f"Set{i+1}" for i in combo),"=",intersection)
Set1 and Set2 = {4, 5}
Set1 and Set3 = set()
Set1 and Set4 = {1}
Set2 and Set3 = {6, 7}
Set2 and Set4 = set()
Set3 and Set4 = {8, 9}
Set1 and Set2 = {4, 5}
Set1 and Set3 = set()
Set1 and Set4 = {1}
Set2 and Set3 = {6, 7}
Set2 and Set4 = set()
Set3 and Set4 = {8, 9}
Set1 and Set2 and Set3 = set()
Set1 and Set2 and Set4 = set()
Set1 and Set3 and Set4 = set()
Set2 and Set3 and Set4 = set()
comboSize in range(2,len(sets))
上的循環combinations
函數獲得sets
列表中的索引combinations
。 例如,對於集合中的 comboSize=3 和 4 個項目,組合將獲得: (0, 1, 2) (0, 1, 3) (0, 2, 3) (1, 2, 3)&
運算符(集交集)與這些索引處的所有集相交,從第一個索引 ( combo[0]
) 開始並將剩余的索引 ( combo[1:]
) 相交成一個集合。f"Set{i+1}"
) 與" and "
字符串連接起來,並在同一行上打印生成的交集。f"Set{i+1}"
是一個格式字符串,它用來自組合 (+1) 的集合索引替換 {i+1}。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.